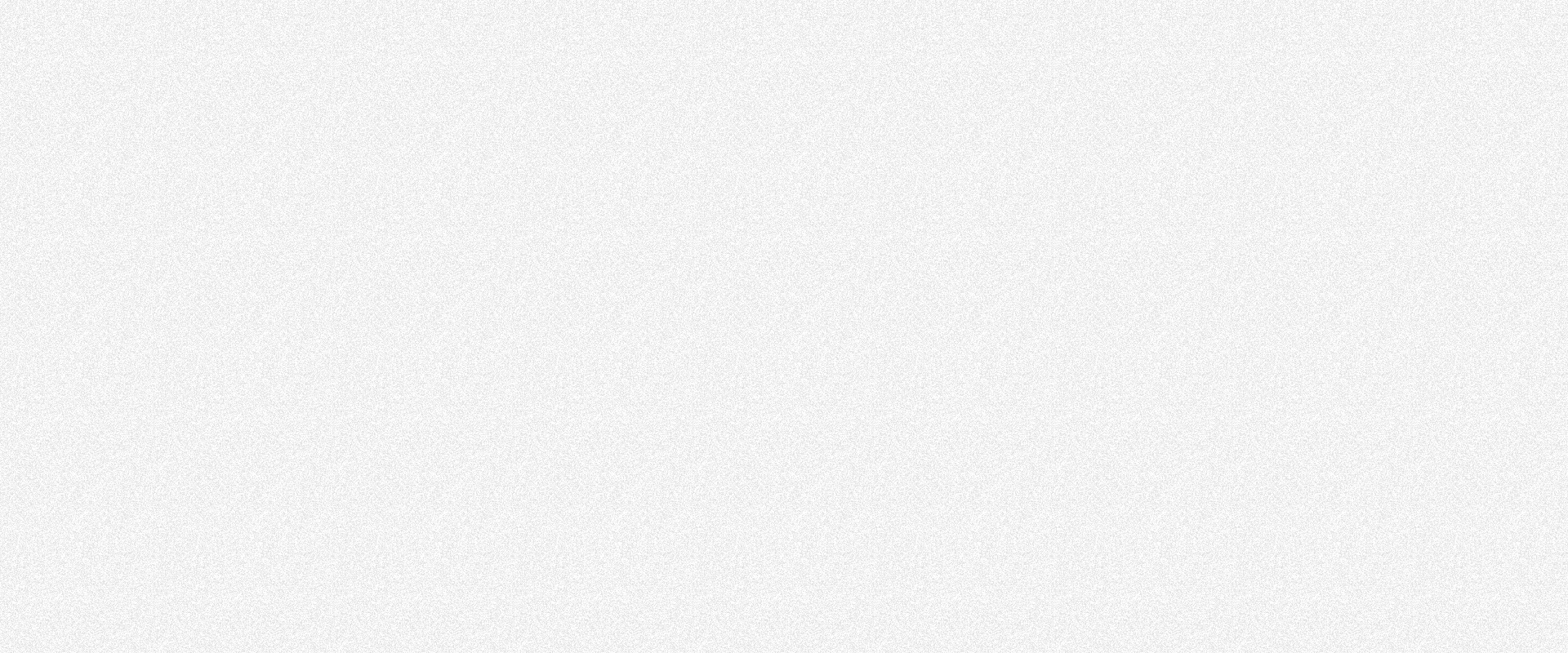
Understanding Laravel routing
1. What is Routing?
- Routing is the process of mapping HTTP requests to appropriate actions or handlers.
- In the context of web development, it determines how URLs are interpreted and processed by the application.
- In Laravel, routing plays a crucial role in defining the structure and behavior of web applications.
2. The Importance of Routing in Laravel
- Routing is a fundamental part of any web application, and Laravel makes it easy to define and manage routes.
- It allows developers to handle different types of HTTP requests, such as GET, POST, PUT, DELETE, and more.
- Routing also enables developers to organize their application’s URLs and define clean and meaningful routes.
3. Basic Routing in Laravel
In Laravel, defining basic routes is straightforward. Routes are typically defined in the routes/web.php
file. Here’s an example of a basic route that responds to a GET request
Route::get('/hello', function () {
return 'Hello, Laravel!';
});
In this example, when a user visits the /hello
URL, the closure function is executed, and the response 'Hello, Laravel!'
is returned.
4. Route Parameters and Wildcards
Routes in Laravel can also accept parameters, allowing developers to create dynamic routes. Parameters are defined by prefixing a route segment with a colon :
.
Here’s an example:
Route::get('/users/{id}', function ($id) {
return "User ID: " . $id;
});
In this case, the id
parameter can be accessed within the route’s closure function. For example, visiting /users/123
will return the response "User ID: 123"
.
5. Named Routes in Laravel
Named routes provide a convenient way to refer to routes by a specific name. They make it easier to generate URLs or redirect to specific routes within the application. Here’s an example of a named route:
Route::get('/profile', function () {
//
})->name('profile');
With a named route, we can generate its URL using the route()
function:
$url = route('profile');
6. Route Groups and Prefixes
Route groups allow developers to apply common attributes or middleware to a set of routes. It helps in organizing routes and reduces redundancy. Route prefixes can be used to prefix a group of routes with a common URL segment. Here’s an example:
Route::prefix('admin')->group(function () {
Route::get('/dashboard', function () {
return 'Admin Dashboard';
});
Route::get('/users', function () {
return 'User Management';
});
});
In this example, the routes /admin/dashboard
and /admin/users
are grouped together and share the /admin
prefix.
7. Route Caching in Laravel
Laravel provides route caching to optimize the performance of your application. When route caching is enabled, Laravel will compile all the routes into a single file, improving the response time of your application. Route caching is particularly useful in production environments where the routes don’t change frequently.
To cache routes, you can use the following artisan command:
php artisan route:cache
8. Route Model Binding
Route model binding is a powerful feature in Laravel that allows you to automatically inject model instances into route callbacks or controller actions. It simplifies the process of fetching model data based on route parameters. Here’s an example:
Route::get('/users/{user}', function (App\Models\User $user) {
return $user;
});
In this example, Laravel will automatically resolve the user
parameter and fetch the corresponding User
model instance.
9. Middleware in Laravel Routing
- Middleware provides a convenient way to filter HTTP requests entering your application.
- It allows you to perform tasks such as authentication, authorization, and request preprocessing.
- Middleware can be applied to individual routes or route groups.
- Laravel comes with several built-in middlewares, and you can also create custom middleware.
10. Route Model Binding with Middleware
- Route model binding can be combined with middleware to perform additional checks or operations on the model instance before executing the route’s callback or controller action.
- This allows you to enforce specific conditions or permissions based on the resolved model.
- For example, you can check if a user has the necessary authorization to access a specific resource.
11. Redirecting Routes in Laravel
Laravel provides a convenient way to redirect routes to another URL or route. It can be useful when you want to create friendly or canonical URLs for your application. Here’s an example:
Route::redirect('/old-url', '/new-url');
In this example, any requests to /old-url
will be redirected to /new-url
.
12. Route Model Binding vs. Implicit Binding
- Route model binding and implicit binding are two techniques in Laravel for automatically injecting model instances into routes or controller actions.
- Implicit binding relies on route parameter naming conventions, whereas route model binding provides more flexibility and control.
- Route model binding is recommended for more complex scenarios.
13. Route Resource Controllers
- Route resource controllers allow you to define multiple routes for CRUD (Create, Read, Update, Delete) operations with a single line of code.
- They provide a convenient way to structure and handle RESTful routes in Laravel.
- Resource controllers can be created using the
--resource
option with the artisan command.
14. 30 Basic Route Examples in Laravel with Explanation and Code
- Basic GET Route:
Route::get('/hello', function () {
return 'Hello, World!';
});
This route responds to a GET request to the /hello
URL and returns the string “Hello, World!”.
- Route with Parameters:
Route::get('/user/{id}', function ($id) {
return "User ID: " . $id;
});
This route accepts a parameter {id}
in the URL and returns the string “User ID: ” followed by the value of the id
parameter.
- Route with Optional Parameter:
This route accepts an optional parameter {id}
in the URL. If the parameter is present, it displays the user ID; otherwise, it displays a message indicating no user ID was provided.
Route::get('/user/{id?}', function ($id = null) {
if ($id) {
return "User ID: " . $id;
}
return "No User ID provided.";
});
- Named Route:
Route::get('/profile', function () {
// Route logic here
})->name('profile');
This route is assigned a name “profile” using the name()
method. The named route can be referenced in other parts of the application, such as generating URLs.
- Route with Middleware:
Route::get('/admin', function () {
// Route logic here
})->middleware('auth');
This route is assigned the “auth” middleware, which ensures that the user must be authenticated before accessing the /admin
URL.
- Route Grouping:
Route::prefix('admin')->group(function () {
Route::get('/dashboard', function () {
// Route logic here
});
Route::get('/users', function () {
// Route logic here
});
});
This example demonstrates how to group multiple routes under the /admin
prefix. The routes within the group share the same prefix and can have additional middleware or other route attributes.
- Resourceful Route:
Route::resource('posts', 'PostController');
This route automatically generates CRUD (Create, Read, Update, Delete) routes for a resource named “posts” and associates them with the PostController
.
- API Resource Route:
Route::apiResource('posts', 'PostController');
Similar to the resource route, the apiResource
the method generates RESTful API routes for the “posts” resource, typically used in API-only applications.
- Route Redirect:
Route::redirect('/old', '/new', 301);
This route redirects requests from /old
to /new
with an HTTP status code 301 (Moved Permanently).
- Fallback Route:
Route::fallback(function () {
return "404 Not Found";
});
The fallback route handles requests that do not match any defined routes. It is typically used to display a custom 404 page.
- Route Caching:
Route::get('/hello', function () {
return 'Hello, World!';
})->name('hello')->middleware('auth')->cache(3600);
This route demonstrates how to enable route caching. The route is assigned a name, middleware, and caching for 3600 seconds.
- Route Model Binding:
Route::get('/user/{user}', function (App\Models\User $user) {
return $user->name;
});
This route uses route model binding to automatically fetch a User
model instance based on the value of the {user}
parameter.
- Route with Regular Expression Constraint:
Route::get('/user/{id}', function ($id) {
return "User ID: " . $id;
})->where('id', '[0-9]+');
This route restricts the {id}
parameter to only accept numeric values using a regular expression constraint.
- Route with Multiple Constraints:
Route::get('/user/{id}/{name}', function ($id, $name) {
return "User: " . $name . " (ID: " . $id . ")";
})->where(['id' => '[0-9]+', 'name' => '[a-zA-Z]+']);
This route sets constraints for both the {id}
and {name}
parameters, allowing only numeric values for {id}
and alphabetic values for {name}
.
- Route with Custom Middleware:
Route::post('/user', function (App\Http\Requests\UserRequest $request) {
// Route logic here
});
This route assigns a custom middleware CheckAdmin
to perform additional checks or validations before allowing access to the /admin
URL.
- Route with Form Request Validation:
Route::post('/user', function (App\Http\Requests\UserRequest $request) {
// Route logic here
});
This route utilizes a form request validation class UserRequest
to validate the incoming request data before processing it further.
- Route with Prefix and Namespace:
Route::prefix('admin')->namespace('Admin')->group(function () {
// Routes within the "Admin" namespace and prefixed with "admin"
});
This route group sets a namespace and prefix for all routes within it. It is useful for organizing routes under a specific namespace or URL prefix.
- Route with Controller Method:
Route::get('/user/{id}', 'UserController@show');
This route maps the /user/{id}
URL to the show
method of the UserController
class.
- Route with Subdomain:
Route::domain('api.example.com')->group(function () {
// Routes for the "api.example.com" subdomain
});
This route group defines routes that are specific to a subdomain, such as api.example.com
.
- Route with Rate Limiting:
Route::middleware('throttle:rate_limit,1')->group(function () {
// Routes with rate limiting
});
This route group applies rate limiting to restrict the number of requests per minute for the enclosed routes.
- Route with Route Caching Exclusion:
Route::get('/hello', function () {
return 'Hello, World!';
})->name('hello')->middleware('auth')->withoutMiddleware('cache');
This route excludes the cache
middleware from being applied, allowing the route to bypass route caching.
- Route with Route Name Prefix:
Route::name('admin.')->group(function () {
Route::get('/dashboard', function () {
// Route logic here
})->name('dashboard');
});
This route group assigns a prefix “admin.” to the names of all routes within it. The example shows a route named “admin.dashboard”.
- Route with Global Constraints:
Route::pattern('id', '[0-9]+');
Route::get('/user/{id}', function ($id) {
return "User ID: " . $id;
});
This example sets a global constraint for the {id}
parameter, ensuring that it accepts only numeric values for all routes.
- Route with Request Methods:
Route::match(['get', 'post'], '/user', function () {
// Route logic here
});
This route responds to both GET and POST requests to the /user
URL.
- Route with CSRF Protection:
Route::post('/user', function () {
// Route logic here
})->middleware('csrf');
This route is protected from Cross-Site Request Forgery (CSRF) attacks by applying the csrf
middleware, which adds CSRF tokens to the requests.
- Route with Route Model Binding Cache:
Route::get('/user/{user}', function (App\Models\User $user) {
return $user->name;
})->middleware('bindings');
This route uses route model binding with caching to improve performance when fetching the associated model based on the {user}
parameter.
- Route with API Versioning:
Route::prefix('v1')->group(function () {
// Routes for API version 1
});
This route group sets a prefix “v1” for the API version, allowing different versions of the API to coexist and be easily managed.
- Route with Subdirectory Namespace:
Route::namespace('Admin')->group(function () {
// Routes within the "Admin" namespace
});
This route group sets a namespace “Admin” for the routes within it, useful for organizing routes under a specific directory structure.
- Route with Multiple Middleware:
Route::get('/admin', function () {
// Route logic here
})->middleware(['auth', 'admin']);
This route is assigned multiple middlewares, such as auth
and admin
, which both need to pass before allowing access to the /admin
URL.
- Route with Custom Route Resolver:
Route::get('/user/{username}', function ($username) {
$user = resolve(UserResolver::class)->resolve($username);
return $user->name;
});
This route utilizes a custom route resolver UserResolver
to fetch the user based on the {username}
parameter, allowing custom logic for resolving route bindings.
15. Conclusion
In this article, we have explored the basics of Laravel routing for beginners. We have covered topics such as basic routing, route parameters, named routes, route groups, route caching, route model binding, middleware, redirecting routes, and more. Laravel’s routing system provides a flexible and powerful way to handle incoming HTTP requests and build web applications. By mastering the concepts of Laravel routing, you can create efficient and well-structured applications.
FAQs
Q1: Can I use Laravel routing for API development?
Yes, Laravel routing can be used for API development as well. Laravel provides dedicated features and tools for building RESTful APIs, including route resource controllers, API resource routes, and API rate limiting.
Q2: How can I handle form submissions in Laravel routing?
To handle form submissions in Laravel, you can define a route that responds to the appropriate HTTP method (usually POST) and specify the action to be taken when the form is submitted. You can access the submitted form data using the Request
object or by using the request()
helper function.
Q3: Can I have multiple route files in Laravel?
Yes, you can have multiple route files in Laravel. It allows you to organize your routes based on different sections or features of your application. You can include these route files in the main routes/web.php
or routes/api.php
file using the include
or require
functions.
Q4: How can I protect certain routes in Laravel from unauthorized access?
You can protect routes in Laravel by applying middleware to them. Middleware provides a convenient way to filter requests and perform authorization checks. Laravel includes built-in middleware like auth
for authentication and guest
for guest access. You can also create custom middleware to enforce specific access rules.
Q5: Can I use Laravel routing for handling AJAX requests?
Yes, Laravel routing can be used to handle AJAX requests. You can define routes that respond to specific AJAX requests using the appropriate HTTP methods (e.g., GET, POST, etc.). In the route’s closure function or controller action, you can process the AJAX request and return the desired response, usually in JSON format.
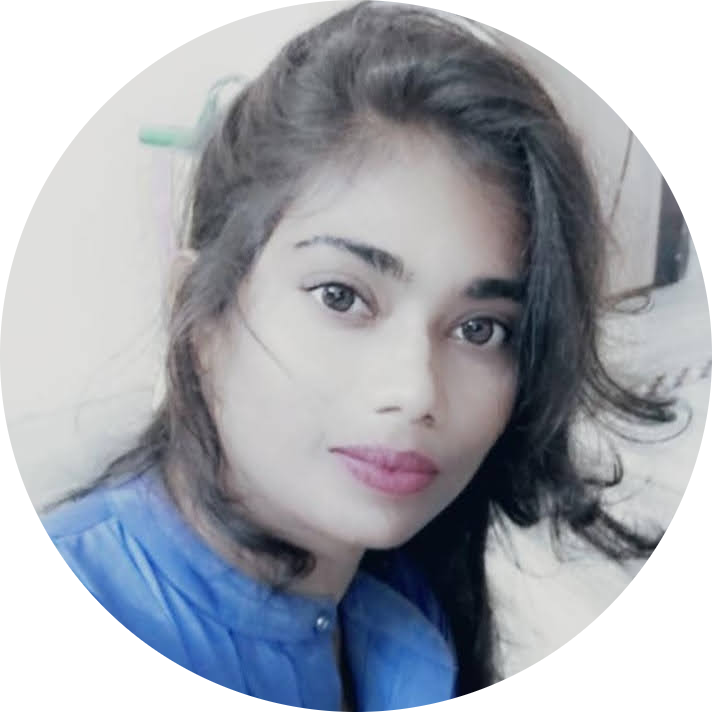
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
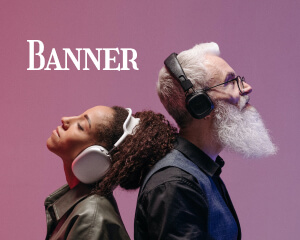