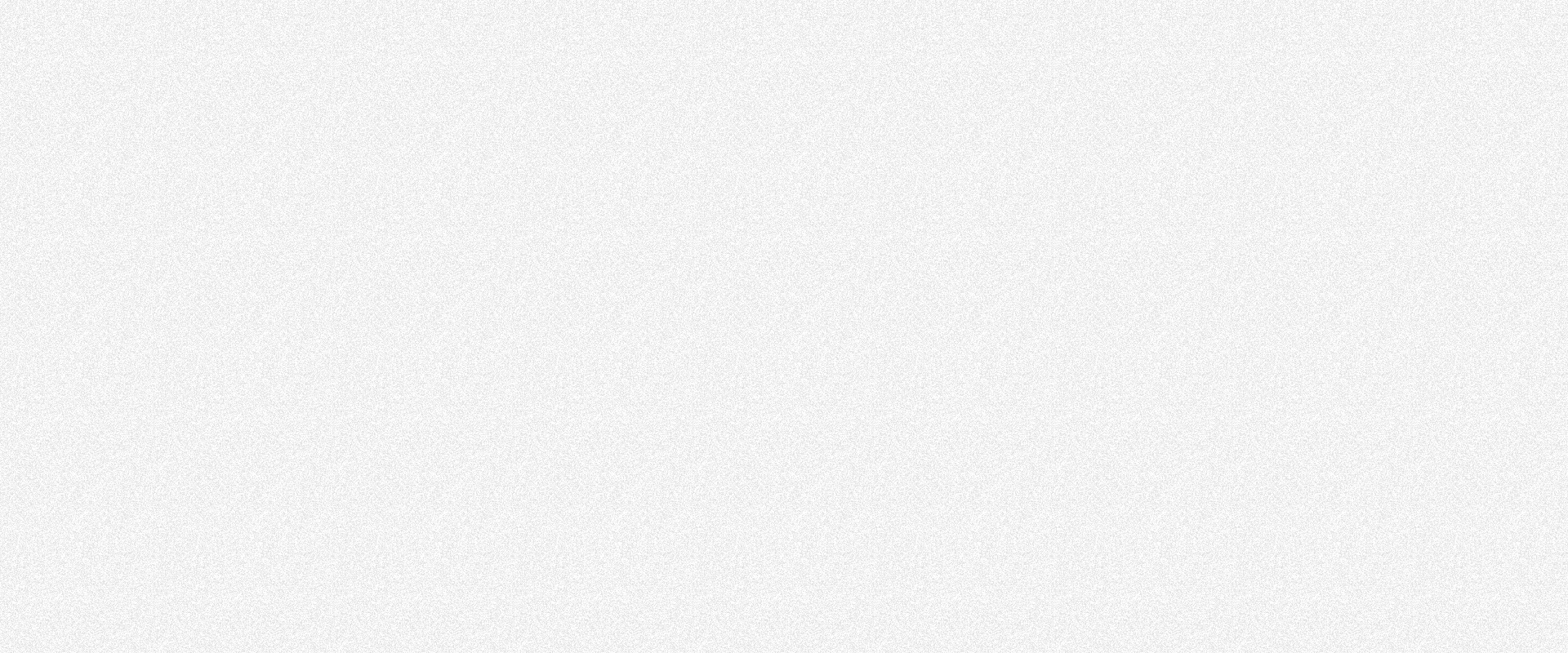
How can you create a user-defined function in PHP?
If you are a developer working with PHP, you might often find yourself in situations where you need to reuse a specific block of code multiple times. Rather than writing the same code over and over again, you can create a user-defined function in PHP. Functions are blocks of code that can be called and executed whenever needed, making your code more organized, efficient, and easier to maintain. In this article, we will guide you through the process of creating a user-defined function in PHP.
1. Introduction to User-Defined Functions
A user-defined function is a custom function created by the programmer to perform specific tasks or calculations. It allows you to break down your code into smaller, manageable parts, making it easier to read and maintain.
2. Syntax of a Function in PHP
In PHP, the basic syntax for creating a function is as follows:
function functionName(parameters) {
// Function body - code to be executed
}
3. Declaring and Calling a Function
To declare a function, use the function
keyword followed by the desired function name. Make sure the function name is descriptive and relates to the task it performs. To call a function, simply write the function name followed by parentheses.
function greetUser() {
echo "Hello, user!";
}
// Call the function
greetUser();
4. Passing Parameters to a Function
Functions can accept parameters, allowing you to pass values to the function when calling it. These parameters act as variables inside the function.
function greetUserByName($name) {
echo "Hello, $name!";
}
// Call the function with a parameter
greetUserByName("John");
5. Returning Values from a Function
A function can also return a value using the return
statement. This is useful when you want the function to calculate something and provide the result.
function addNumbers($a, $b) {
return $a + $b;
}
// Call the function and store the result in a variable
$sum = addNumbers(10, 5);
echo "The sum is: " . $sum;
6. Variable Scope in Functions
Variables declared inside a function are considered local to that function, and they cannot be accessed outside of it. However, you can use the global
keyword to access global variables from within a function.
$globalVar = 10;
function modifyGlobalVar() {
global $globalVar;
$globalVar = 20;
}
modifyGlobalVar();
echo $globalVar; // Output: 20
7. Recursive Functions in PHP
A recursive function is a function that calls itself, either directly or indirectly. This technique is useful for solving problems that can be broken down into smaller sub-problems.
function factorial($n) {
if ($n <= 1) {
return 1;
} else {
return $n * factorial($n - 1);
}
}
echo factorial(5); // Output: 120
8. Anonymous Functions (Closures)
Anonymous functions, also known as closures, are functions without a specified name. They can be assigned to variables and used as callback functions.
$greet = function($name) {
echo "Hello, $name!";
};
$greet("Alice"); // Output: Hello, Alice!
9. Predefined PHP Functions
PHP comes with a vast collection of built-in functions that can be directly used in your code. These functions cover various tasks like string manipulation, array operations, mathematical calculations, and more.
10. Best Practices for Function Naming
When naming functions, use descriptive and meaningful names that reflect the purpose of the function. This makes your code easier to understand for other developers.
11. Avoiding Common Function Mistakes
Be careful not to use the same function name multiple times, as it will lead to conflicts and unexpected behavior. Additionally, ensure that your function calls have the correct number and order of parameters.
12. Tips for Optimizing Function Performance
To improve the performance of your functions, avoid using too many nested functions and prioritize efficient algorithms.
13. Utilizing Functions for Code Reusability
Creating reusable functions is an essential aspect of writing maintainable and organized code. Aim to create functions that can be easily adapted for different use cases.
14. Debugging Functions in PHP
When encountering issues with functions, use debugging techniques such as var_dump()
and echo
statements to inspect variables and the flow of your code.
15. Conclusion
In conclusion, user-defined functions play a crucial role in PHP development. They allow you to create modular and reusable code, making your applications more efficient and maintainable. By mastering the art of function creation and utilization, you can significantly enhance your PHP programming skills.
FAQs
- Can I create functions with the same name but different parameters? Yes, PHP supports function overloading, allowing you to create functions with the same name but different parameter lists.
- What is the maximum number of parameters a PHP function can accept? PHP does not have a strict limit on the number of parameters a function can accept, but it is advisable to keep the parameter list reasonable for better code readability.
- Is it mandatory to return a value from a function? No, functions can have a
void
return type, meaning they do not return any value. - Can I call a function from within another function? Yes, functions can call other functions, including calling themselves in the case of recursive functions.
- How do I ensure my function names are unique to avoid conflicts? It is good practice to use a unique prefix or namespace for your functions to minimize the chance of conflicts with functions from external libraries or frameworks.
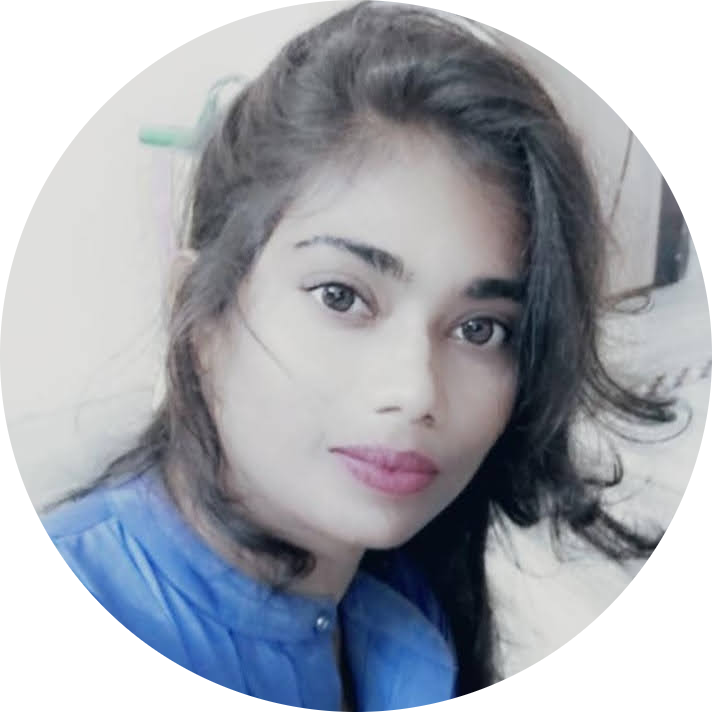
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
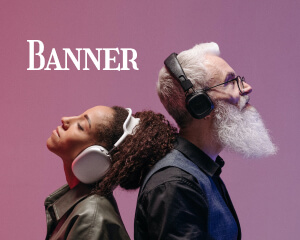