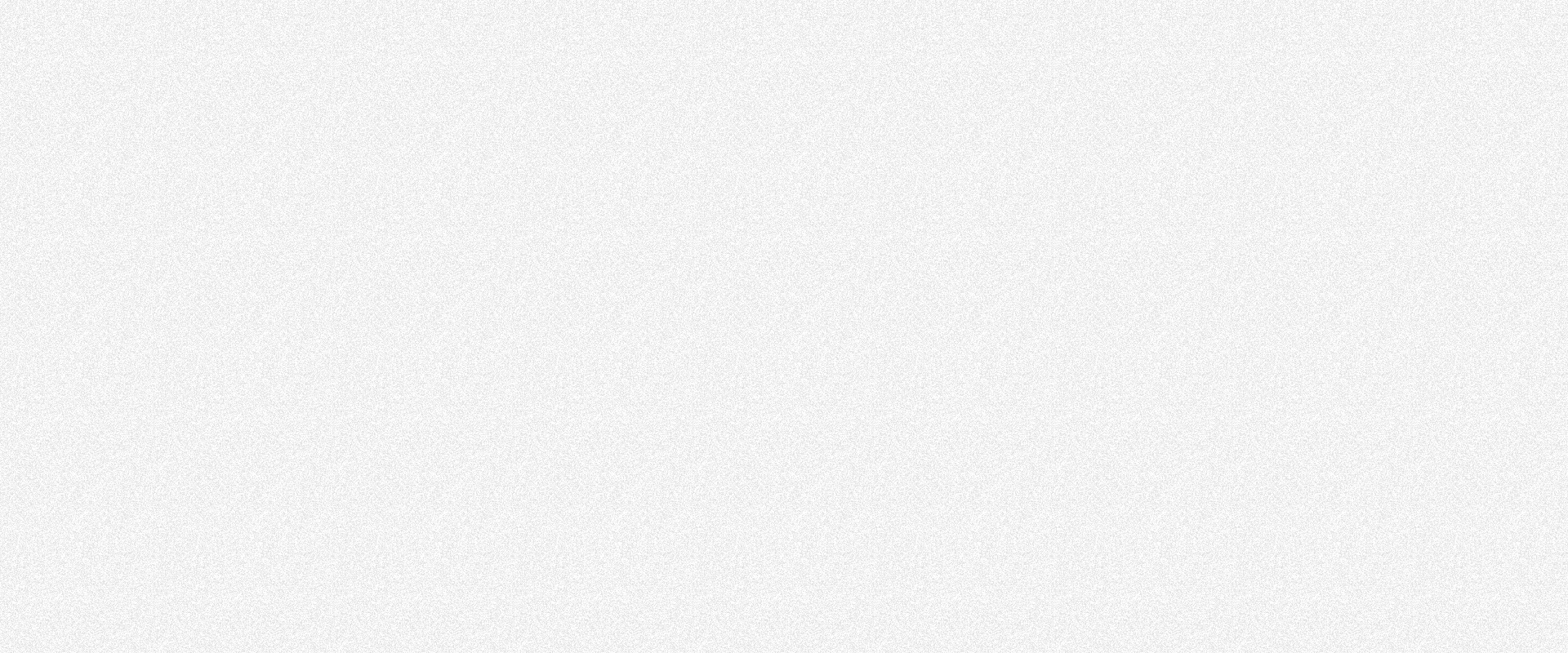
How can you send emails using PHP?
In today’s digital world, email remains an essential means of communication. Whether it’s for sending notifications, newsletters, or transactional messages, integrating email functionality into web applications is crucial. PHP, being a versatile server-side scripting language, provides powerful tools for sending emails. In this article, we will explore how to send emails using PHP effectively.
How can you send emails using PHP?
Sending emails using PHP is a straightforward process. Let’s dive into the steps you need to follow to accomplish this task.
Step 1: Setting up a Local or Remote SMTP Server
Before you start sending emails, you need access to an SMTP (Simple Mail Transfer Protocol) server. An SMTP server handles the outgoing email delivery process. You can either set up a local SMTP server or use a third-party service like Gmail’s SMTP server.
Step 2: Installing PHPMailer
To streamline the email-sending process and overcome PHP’s limitations, we recommend using the PHPMailer library. PHPMailer provides a simple and reliable interface to send emails via PHP. To install PHPMailer, follow these steps:
- Download the PHPMailer library from the official GitHub repository.
- Extract the files and include the PHPMailerAutoload.php file in your PHP project.
Step 3: Configuring SMTP Settings
Next, you need to configure PHPMailer with the SMTP server settings. This involves providing the SMTP server address, port number, username, password, and encryption type. Here’s an example of how you can configure PHPMailer:
<?php
require 'PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->Port = 587;
$mail->SMTPSecure = 'tls';
$mail->SMTPAuth = true;
$mail->Username = 'your_email@example.com';
$mail->Password = 'your_email_password';
$mail->setFrom('your_email@example.com', 'Your Name');
$mail->addAddress('recipient@example.com', 'Recipient Name');
$mail->Subject = 'Test Email from PHPMailer';
$mail->Body = 'This is a test email sent using PHPMailer.';
$mail->send();
?>
Step 4: Composing and Sending Emails
With PHPMailer properly configured, you can now compose and send emails. Use the following code as an example:
<?php
require 'PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP();
// Configure SMTP settings here
$mail->setFrom('your_email@example.com', 'Your Name');
$mail->addAddress('recipient@example.com', 'Recipient Name');
$mail->Subject = 'Test Email from PHPMailer';
$mail->Body = 'This is a test email sent using PHPMailer.';
$mail->AltBody = 'If you cannot view this message, please switch to the HTML view.';
$mail->send();
?>
Make sure to replace placeholders with actual email addresses and content.
Step 5: Handling Errors
To ensure your email functionality is robust, implement error handling. Check for errors during the email sending process and provide appropriate feedback to users when necessary.
Step 6: Including Attachments
To send attachments with your emails, use the addAttachment
method provided by PHPMailer. This allows you to attach files from your server to outgoing emails.
$mail->addAttachment('/path/to/file.pdf', 'Attachment Name');
Step 7: Personalizing Emails
Personalization enhances user engagement. You can customize email content using variables and placeholders. PHPMailer allows you to set the email’s body using HTML, making it easier to create visually appealing and personalized emails.
$mail->Body = 'Hello, [NAME]! Welcome to our newsletter.';
$mail->AltBody = 'Hello, [NAME]! Welcome to our newsletter.';
$mail->replace('NAME', 'John Doe');
Advantages of Sending Emails Using PHP
Now that you know how to send emails using PHP, let’s explore the benefits of using PHP for email functionality:
- Ease of Integration: PHP’s integration with various databases and web frameworks makes it seamless to incorporate email features into web applications.
- Customization: With PHPMailer and PHP’s flexible nature, you can create customized email templates and tailor them to suit your branding and user preferences.
- Automation: PHP allows you to automate email sending, making it efficient for tasks like sending order confirmations, password resets, and notifications.
- Cost-Effective: PHPMailer is an open-source library, and PHP itself is free, making it a cost-effective solution for email functionality.
- Error Handling: PHP provides excellent error handling capabilities, enabling you to identify and address issues promptly.
- Versatility: PHP supports various email protocols and can be used with both local and third-party SMTP servers.
Frequently Asked Questions (FAQs)
- Can I send HTML emails using PHP?
- Yes, PHPMailer supports sending HTML emails. You can include HTML tags in the email body to create visually appealing content.
- Is PHP the only language to send emails from a website?
- No, other server-side languages like Python and Node.js can also be used to send emails. However, PHP remains a popular choice due to its simplicity and wide usage.
- Can I use PHP to send bulk emails?
- Yes, you can use PHP to send bulk emails. However, keep in mind that some email providers may have limitations on the number of emails you can send per day.
- How do I handle email attachments in PHP?
- You can use the
addAttachment
method provided by PHPMailer to attach files to your outgoing emails. Make sure to specify the correct file path and name.
- You can use the
- What are the common reasons for email delivery failure?
- Email delivery failure can occur due to various reasons, such as incorrect SMTP settings, blocked email addresses, or issues with the recipient’s email server.
- Can I send emails without using a third-party SMTP server?
- Yes, you can set up and use a local SMTP server to send emails directly. However, using a reliable third-party SMTP service is recommended for better deliverability and fewer configuration hassles.
Conclusion
Sending emails using PHP opens up a world of possibilities for web developers. By following the steps outlined in this guide, you can seamlessly integrate email functionality into your web applications. PHP’s flexibility and the power of PHPMailer make it a robust choice for handling email communication. Whether it’s sending personalized newsletters or transactional emails, PHP has got you covered.
So, go ahead and implement email functionality in your PHP projects, and watch your web applications communicate effectively with your users!
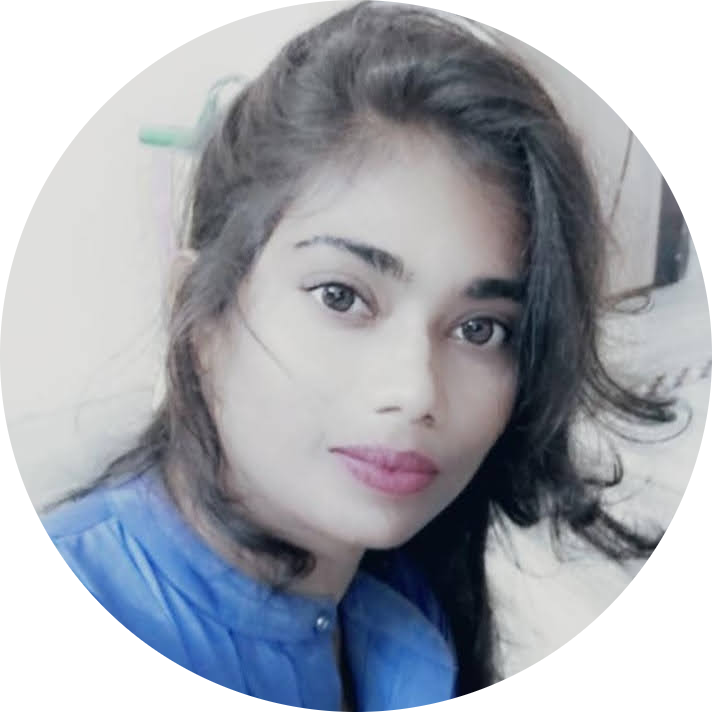
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
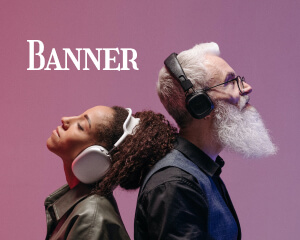