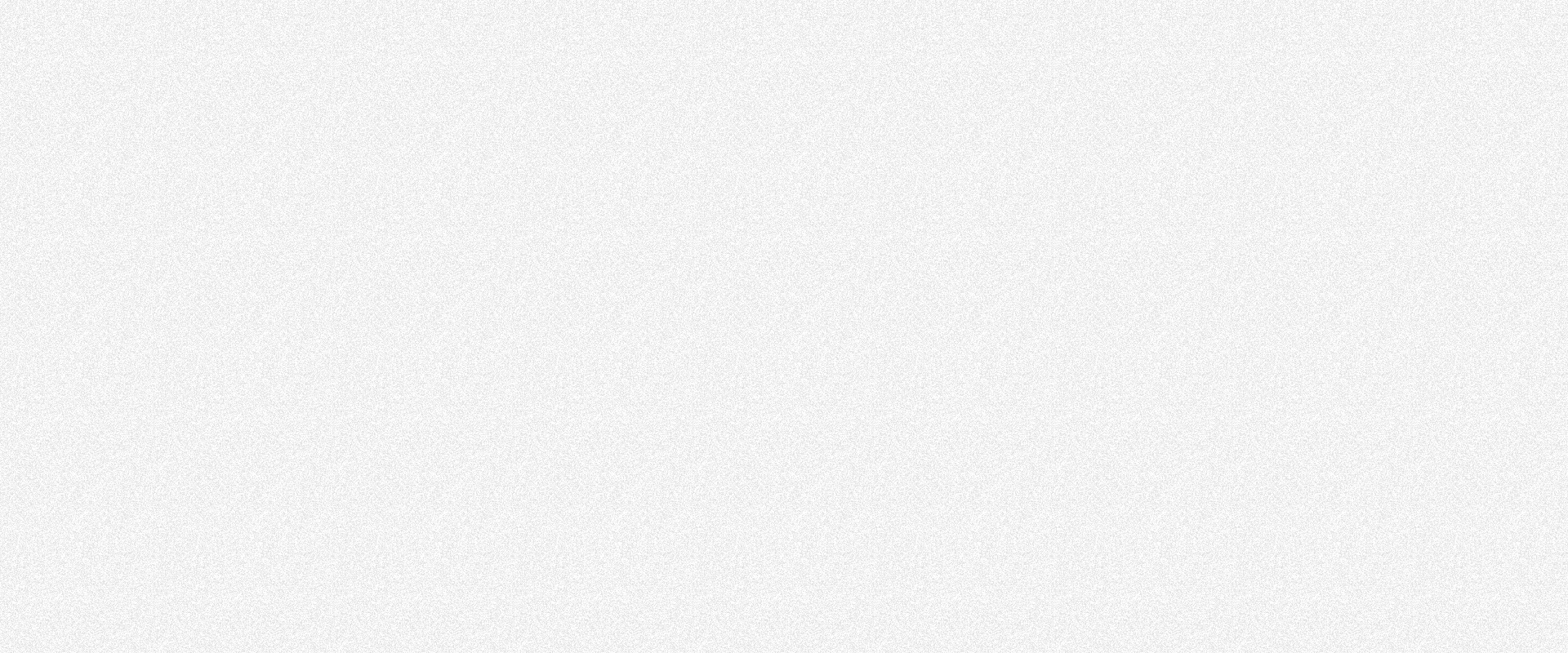
How can you write test cases for a PHP class using PHPUnit?
Writing test cases for your PHP class is an essential aspect of software development. Test cases help verify that your code works as expected, prevent regressions, and improve the overall quality of your PHP applications. PHPUnit is a widely used testing framework for PHP that makes it easy to write and run unit tests. In this article, we will delve into the process of writing test cases for a PHP class using PHPUnit. We will cover various topics, from the installation of PHPUnit to creating test suites, data providers, and more. Let’s get started!
How Can You Write Test Cases for a PHP Class Using PHPUnit?
Installing PHPUnit
Before we start writing test cases, we need to set up PHPUnit. PHPUnit can be installed using Composer, a popular dependency management tool for PHP. Follow these steps to install PHPUnit:
- Open your terminal or command prompt.
- Navigate to your project directory.
- Run the following command to install PHPUnit:
bashCopy codecomposer require --dev phpunit/phpunit
Writing Your First Test Case
Now that PHPUnit is installed, let’s write our first test case. In PHPUnit, test cases are written as methods within a test class, which extends the PHPUnit\Framework\TestCase
class. The test methods typically follow the “Arrange-Act-Assert” pattern. Here’s an example of a simple test case:
use PHPUnit\Framework\TestCase;
class YourClassTest extends TestCase
{
public function testSomething()
{
// Arrange
$yourClassInstance = new YourClass();
// Act
$result = $yourClassInstance->someMethod();
// Assert
$this->assertEquals(expectedValue, $result);
}
}
In this example, we create an instance of YourClass
, call a method someMethod()
, and then use an assertion to check if the result matches the expected value.
Organizing Test Cases in Test Suites
As your project grows, you may have multiple test cases for different classes. PHPUnit allows you to organize your test cases into test suites. Test suites are groups of test cases that can be executed together. This helps manage and run specific sets of tests easily. To create a test suite, you can use the PHPUnit\Framework\TestSuite
class and add test cases to it. Here’s an example:
use PHPUnit\Framework\TestSuite;
class AllTests
{
public static function suite()
{
$suite = new TestSuite('All Tests');
$suite->addTestSuite('YourClassTest');
$suite->addTestSuite('AnotherClassTest');
// Add more test cases here...
return $suite;
}
}
Using Data Providers
Data providers are handy when you want to run the same test with multiple sets of input data. This helps test different scenarios using a single test method. To use a data provider, you need to annotate your test method with the @dataProvider
annotation and create a separate method that returns an array of test data. Here’s an example:
use PHPUnit\Framework\TestCase;
class YourClassTest extends TestCase
{
/**
* @dataProvider additionDataProvider
*/
public function testAddition($a, $b, $expected)
{
$calculator = new Calculator();
$result = $calculator->add($a, $b);
$this->assertEquals($expected, $result);
}
public function additionDataProvider()
{
return [
[2, 3, 5],
[5, 5, 10],
[0, 0, 0],
];
}
}
Leveraging PHPUnit Annotations
PHPUnit provides various annotations that allow you to control the behavior of your test cases. Some commonly used annotations include:
@depends
: Specifies dependencies between test methods, ensuring a specific order of execution.@before
: Indicates a method to be executed before each test method.@after
: Indicates a method to be executed after each test method.
Utilizing these annotations can make your test cases more organized and efficient.
Mocking Dependencies with PHPUnit
When writing unit tests, it’s essential to isolate the class under test from its dependencies. PHPUnit allows you to create mock objects that simulate the behavior of dependencies, enabling you to test your class in isolation. Mocking dependencies ensures that any failures are related to the class being tested and not its collaborators. Here’s an example of using PHPUnit’s mocking feature:
use PHPUnit\Framework\TestCase;
class YourClassTest extends TestCase
{
public function testSomethingWithMock()
{
$dependencyMock = $this->createMock(YourDependency::class);
$dependencyMock->method('someMethod')->willReturn('Mocked Result');
$yourClassInstance = new YourClass($dependencyMock);
$result = $yourClassInstance->someMethodThatUsesDependency();
$this->assertEquals('Mocked Result', $result);
}
}
Code Coverage and Reporting
PHPUnit provides code coverage reports that help you identify which parts of your codebase are covered by tests. This information is crucial for identifying areas that require more thorough testing. You can generate code coverage reports using the --coverage-html
or --coverage-text
options. For example:
vendor/bin/phpunit --coverage-html report
Frequently Asked Questions (FAQs)
- How do I install PHPUnit?
- PHPUnit can be installed using Composer. Simply run
composer require --dev phpunit/phpunit
in your project directory.
- PHPUnit can be installed using Composer. Simply run
- What is the purpose of writing test cases for a PHP class?
- Writing test cases helps verify that your code works as expected, prevents regressions, and improves the overall quality of your PHP applications.
- How can I organize my test cases into test suites with PHPUnit?
- You can create test suites by using the
PHPUnit\Framework\TestSuite
class and adding your test cases to it.
- You can create test suites by using the
- How do data providers work in PHPUnit?
- Data providers allow you to run the same test with multiple sets of input data, helping you test different scenarios using a single test method.
- Why is it essential to mock dependencies in PHPUnit?
- Mocking dependencies isolates the class under test from its collaborators, ensuring that any failures are related to the class being tested and not its dependencies.
- How can I generate code coverage reports in PHPUnit?
- You can generate code coverage reports using the
--coverage-html
or--coverage-text
options with PHPUnit.
- You can generate code coverage reports using the
Conclusion
Writing test cases for a PHP class using PHPUnit is a crucial aspect of software development. It ensures the reliability and quality of your code, helping you catch bugs early in the development process. In this article, we covered various aspects of writing test cases with PHPUnit, from installation to leveraging advanced features like data providers and mocking dependencies. By following these practices and continuously improving your test suite, you can maintain a robust and efficient codebase.
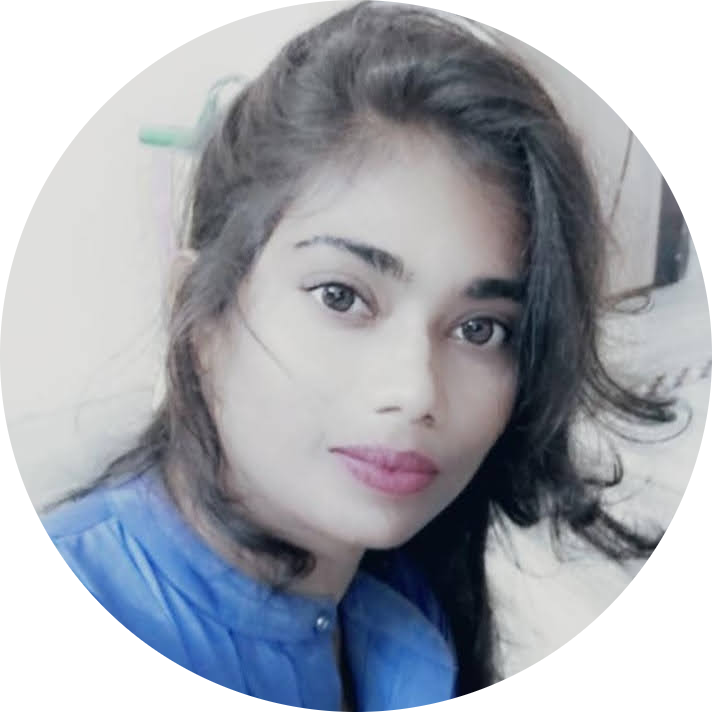
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
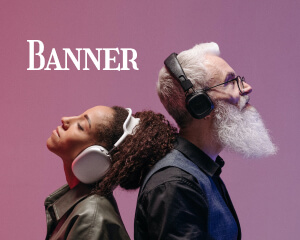