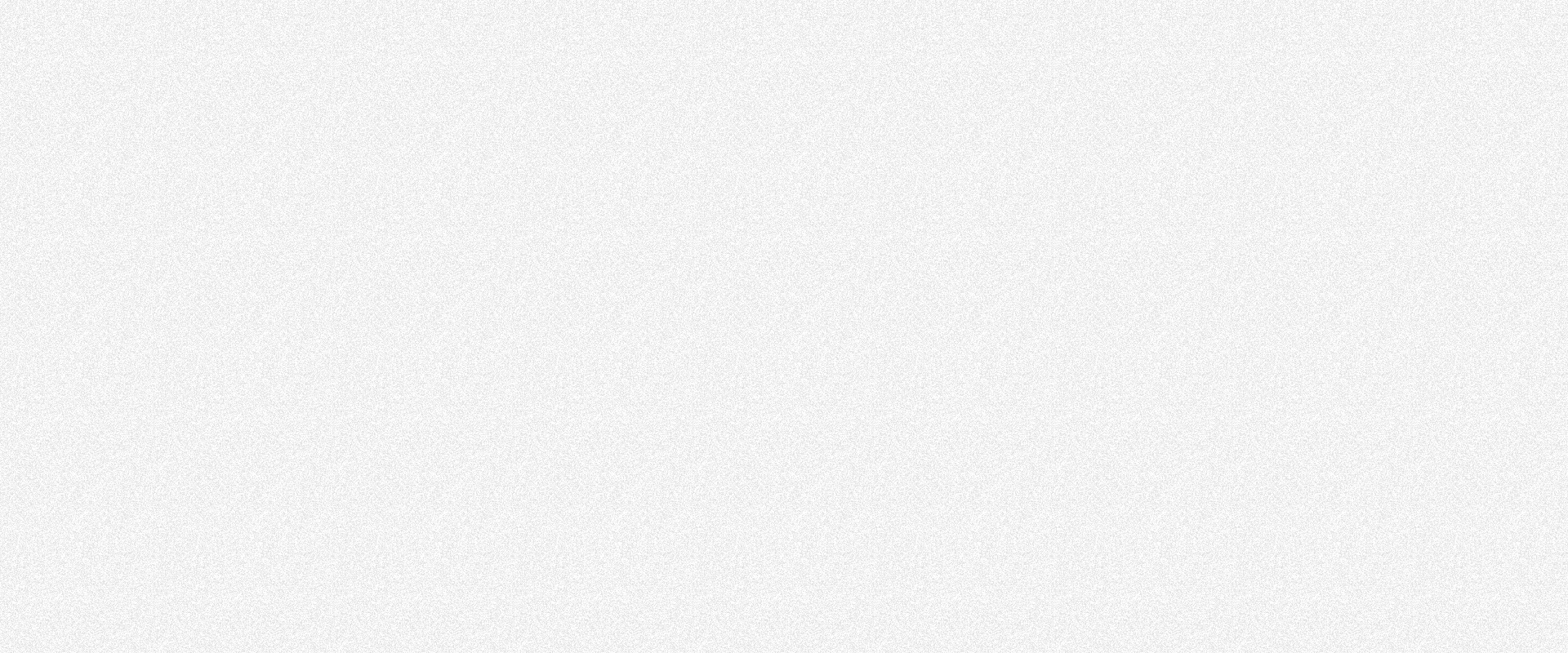
How do you define routes in a PHP framework?
In the world of web development, PHP frameworks play a crucial role in simplifying the process of building robust and scalable applications. One fundamental aspect of PHP frameworks is the management of routes, which determines how the application handles incoming requests. In this article, we will dive deep into the topic of defining routes in a PHP framework. We will cover everything from the basics to advanced techniques, providing you with a complete understanding of the process. So, let’s get started!
How do you define routes in a PHP framework?
Defining routes in a PHP framework involves mapping URLs to specific actions or controllers in your application. It allows you to direct user requests to the appropriate code, ensuring seamless navigation and interaction with your web application.
Understanding the Structure of a Route
Before we delve into the specifics of defining routes, let’s understand the structure of a typical route. A route is comprised of three main components:
- HTTP Verb: This determines the type of request the route should respond to, such as GET, POST, PUT, DELETE, etc.
- URL Pattern: The URL pattern represents the route’s unique identifier and is used to match incoming requests.
- Action/Controller: It refers to the code that should be executed when the corresponding URL is accessed.
Creating Routes in PHP Frameworks
Different PHP frameworks may have varying methods for defining routes. However, most frameworks follow a similar approach. Let’s explore how you can create routes in some popular PHP frameworks:
1. Laravel
Laravel, being one of the most widely used PHP frameworks, offers a concise and expressive syntax for defining routes. Here’s an example:
Route::get('/home', 'HomeController@index');
In this example, when a GET request is made to the URL ‘/home,’ the index
method of the HomeController
will be executed.
2. Symfony
Symfony, another powerful PHP framework, utilizes the YAML configuration format for defining routes. An example is as follows:
homepage:
path: /home
controller: App\Controller\HomeController::index
methods: [GET]
This code snippet sets up a route where a GET request to ‘/home’ will trigger the index
method of the HomeController
.
3. CodeIgniter
CodeIgniter, known for its simplicity and ease of use, allows you to define routes using a straightforward approach:
$route['home'] = 'HomeController';
This route will direct all requests to ‘/home’ to the HomeController
.
Best Practices for Defining Routes
To ensure a well-structured and maintainable application, follow these best practices when defining routes:
- Use descriptive and meaningful URL patterns to enhance readability and SEO-friendliness.
- Leverage HTTP verbs appropriately. Use GET for fetching data, POST for creating resources, PUT for updating resources, and DELETE for removing resources.
- Implement route grouping to organize related routes and apply middleware for common functionalities.
- Avoid using complex logic within routes; delegate the actual processing to controllers or dedicated service classes.
Advanced Techniques for Routing
As you become more proficient with route definition, you can explore some advanced techniques to optimize your application’s routing system:
1. Route Parameters
Route parameters allow you to capture dynamic values from the URL and pass them to the controller. For instance:
Route::get('/user/{id}', 'UserController@show');
Here, the {id}
segment acts as a placeholder, capturing the user ID from the URL and passing it to the show
method in the UserController
.
2. Named Routes
Named routes give your routes a unique identifier, making it easier to reference them throughout the application. This is especially useful for generating URLs for specific routes:
Route::get('/product/{id}', 'ProductController@show')->name('product.show');
Now, you can generate the URL for this route using the route’s name instead of the URL pattern.
3. Route Prefixing
Route prefixing allows you to add a common prefix to a group of routes, reducing duplication and improving code organization:
Route::prefix('admin')->group(function () {
Route::get('/dashboard', 'AdminController@dashboard');
Route::get('/users', 'AdminController@users');
// More routes...
});
In this example, all routes within the ‘admin’ prefix will be accessible via ‘/admin/…’.
FAQs
How do you define a default route in a PHP framework?
In most PHP frameworks, you can define a default route that will be executed when no other matching route is found. For example, in Laravel:
Route::fallback(function () {
return '404 Not Found';
});
This code sets up a fallback route that will be triggered if no other route matches the incoming request.
Can you have multiple actions for a single route?
Yes, many PHP frameworks allow you to define multiple actions for a single route, often known as route chaining. For instance, in Laravel, you can chain middleware and multiple actions to a route:
Route::get('/profile', 'ProfileController@show')
->middleware('auth')
->name('profile.show');
Here, the route has a middleware (auth
) and an action (ProfileController@show
).
How do you handle 404 Not Found errors in routes?
Handling 404 errors is essential to improve user experience. In PHP frameworks, you can define a fallback route or utilize custom error handling methods to gracefully handle 404 errors and display user-friendly messages.
What is the role of route caching in PHP frameworks?
Route caching is a performance optimization technique where the framework compiles all defined routes into a single, cached file. This significantly speeds up the route resolution process, resulting in faster application performance.
Can you nest routes in PHP frameworks?
Yes, many PHP frameworks allow you to nest routes, enabling better organization and control over your application’s routing structure. For instance, in Laravel:
Route::prefix('admin')->group(function () {
Route::get('/dashboard', 'AdminController@dashboard');
Route::prefix('users')->group(function () {
Route::get('/', 'UserController@index');
Route::get('/{id}', 'UserController@show');
});
});
Here, the ‘users’ routes are nested under the ‘admin’ prefix.
What is route model binding in PHP frameworks?
Route model binding is a feature that automatically injects model instances into your controller methods based on route parameters. This saves you from manually fetching the model instance. For example, in Laravel:
Route::get('/user/{user}', 'UserController@show');
The {user}
parameter will be automatically resolved to the corresponding User
model instance.
Conclusion
Defining routes is a critical aspect of building PHP web applications. It allows you to manage user requests efficiently and create a seamless user experience. In this article, we explored the fundamentals of defining routes in PHP frameworks, along with best practices and advanced techniques. Armed with this knowledge, you can now create well-structured and high-performing applications. So go ahead, experiment with different PHP frameworks, and unleash the full potential of routing in your projects!
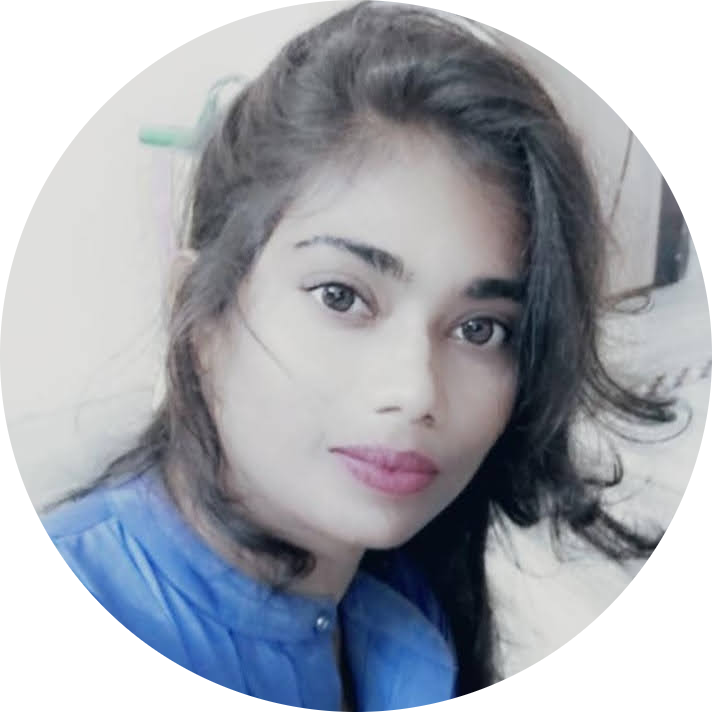
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
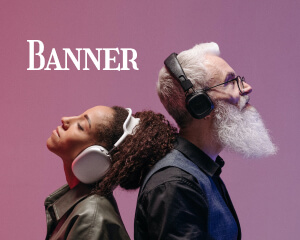