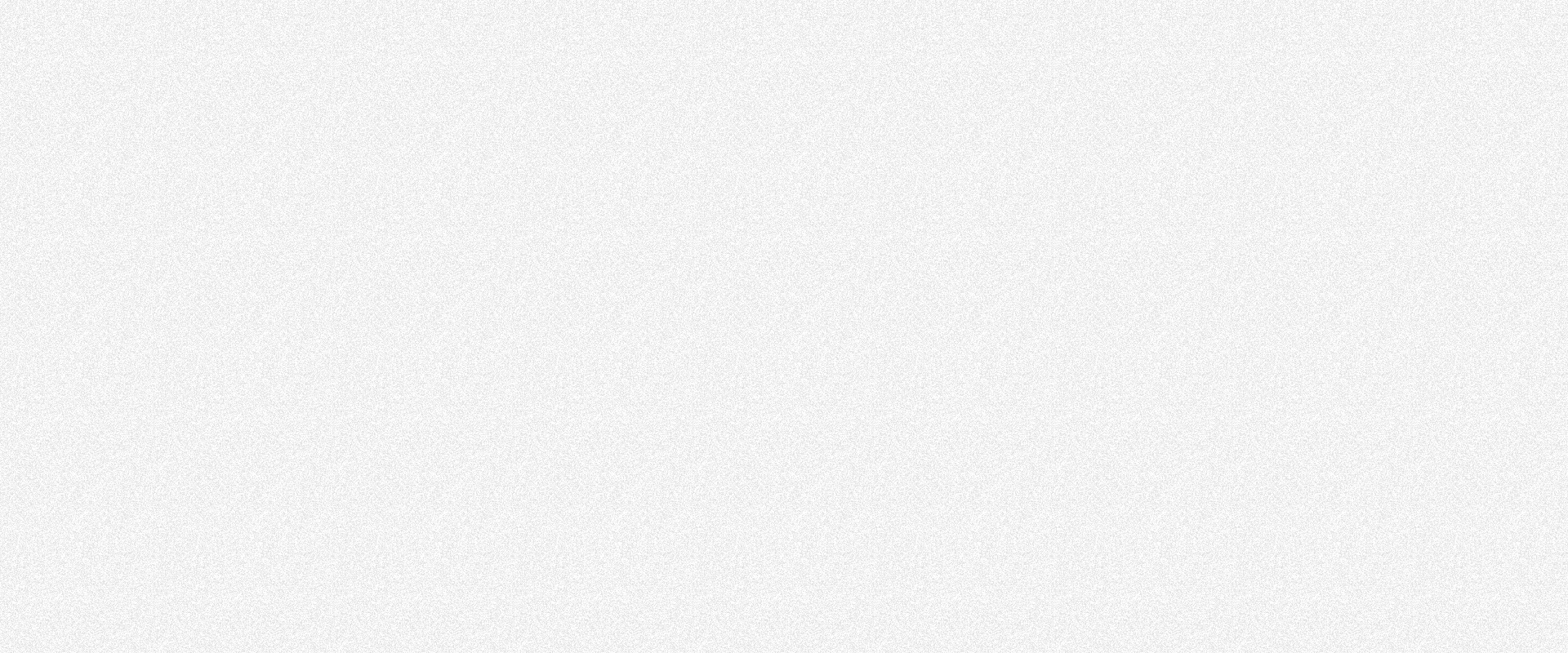
How do you handle transactions in PHP?
In the world of web development, PHP stands as one of the most popular programming languages for building dynamic and interactive websites. When it comes to managing databases and ensuring data integrity, handling transactions becomes crucial. This article aims to provide you with a detailed understanding of how to handle transactions in PHP effectively. Whether you are a seasoned developer or just getting started, this guide will equip you with the knowledge and techniques to manage transactions efficiently.
How do you handle transactions in PHP?
Handling transactions in PHP involves managing a series of database operations as a single unit. Transactions ensure that either all the operations within the transaction are completed successfully, or none of them take effect if any operation fails. The process of handling transactions in PHP is achieved through a set of built-in functions and methods provided by PHP, combined with SQL statements executed on the database.
Understanding Transactions and ACID Properties
Before diving into the technicalities, it’s essential to understand the fundamental concepts behind transactions and the ACID properties. ACID stands for Atomicity, Consistency, Isolation, and Durability, which are the four key properties that a transaction must possess to maintain data integrity. Let’s briefly explore each of these properties:
- Atomicity: Transactions are atomic, meaning they either occur entirely or not at all. If any part of the transaction fails, the entire transaction is rolled back, leaving the database in its original state.
- Consistency: Transactions bring the database from one consistent state to another. The data should satisfy all the integrity constraints defined in the database schema.
- Isolation: Transactions occur independently of each other, ensuring that the concurrent execution of multiple transactions does not affect the outcome of each other.
- Durability: Once a transaction is committed, the changes made to the database become permanent and survive system failures.
Starting and Committing a Transaction
In PHP, you can initiate a transaction using the beginTransaction()
method. This marks the beginning of a transaction. All subsequent database operations will be part of this transaction until it is either committed or rolled back. To commit a transaction, you can use the commit()
method.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// ...
// Commit the transaction
$pdo->commit();
} catch (PDOException $e) {
// Handle exception and roll back the transaction
$pdo->rollBack();
}
?>
Rolling Back a Transaction
In some cases, you may want to undo a transaction and revert any changes made so far. This can be achieved using the rollBack()
method. The rollback will cancel all the changes made within the transaction.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// ...
// Check for some condition to decide whether to commit or rollback
if ($someCondition) {
$pdo->commit();
} else {
$pdo->rollBack();
}
} catch (PDOException $e) {
// Handle exception and roll back the transaction
$pdo->rollBack();
}
?>
Setting Savepoints
Savepoints are intermediate points within a transaction that allow you to roll back to a specific point without undoing the entire transaction. PHP supports savepoints using the setSavepoint()
method and rolling back to savepoints using the rollBackTo()
method.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// Set a savepoint
$pdo->setSavepoint("savepoint1");
// More database operations
// Roll back to the savepoint
$pdo->rollBackTo("savepoint1");
// More database operations
// Commit the transaction
$pdo->commit();
} catch (PDOException $e) {
// Handle exception and roll back the transaction
$pdo->rollBack();
}
?>
Handling Errors and Exceptions
When dealing with transactions in PHP, it’s essential to handle errors and exceptions properly. Uncaught exceptions can leave the database in an inconsistent state. Always use a try-catch block to handle exceptions and roll back the transaction when necessary.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// Commit the transaction
$pdo->commit();
} catch (PDOException $e) {
// Handle exception and roll back the transaction
$pdo->rollBack();
echo "An error occurred: " . $e->getMessage();
}
?>
Implementing Transaction Isolation Levels
Transaction isolation levels determine how transactions interact with each other in a concurrent environment. PHP supports different isolation levels, allowing you to balance data consistency and performance.
- READ UNCOMMITTED: Allows a transaction to read uncommitted changes made by other transactions.
- READ COMMITTED: Allows a transaction to read only committed changes made by other transactions.
- REPEATABLE READ: Ensures that a transaction sees the same data throughout its execution, even if other transactions are modifying the data.
- SERIALIZABLE: Ensures that transactions are executed serially, preventing concurrent changes to the data.
You can set the isolation level using the setIsolation()
method.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Set isolation level to SERIALIZABLE
$pdo->setAttribute(PDO::ATTR_ISOLATION_LEVEL, PDO::SQLSRV_TXN_SERIALIZABLE);
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// Commit the transaction
$pdo->commit();
} catch (PDOException $e) {
// Handle exception and roll back the transaction
$pdo->rollBack();
}
?>
Handling Deadlocks
Deadlocks occur when two or more transactions are waiting for each other to release locks on resources. PHP provides a method to detect deadlocks and handle them gracefully.
Example:
<?php
try {
// Establish a database connection
$pdo = new PDO("mysql:host=localhost;dbname=mydatabase", "username", "password");
// Set the error mode to EXCEPTION
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
// Begin the transaction
$pdo->beginTransaction();
// Perform database operations here
// Commit the transaction
$pdo->commit();
} catch (PDOException $e) {
// Handle deadlock and retry the transaction if necessary
if ($e->getCode() == 40001) {
// Retry the transaction
// ...
} else {
// Roll back the transaction
$pdo->rollBack();
}
}
?>
Best Practices for Transaction Management
To ensure smooth and efficient transaction management in PHP, consider the following best practices:
- Always use try-catch blocks to handle exceptions and errors during transactions.
- Keep transactions short and focused on specific tasks to reduce the likelihood of deadlocks.
- Avoid long-running transactions that can lead to resource contention and performance issues.
- Use appropriate isolation levels based on your application’s requirements.
- Implement proper error logging and monitoring to track transaction-related issues.
- Test your transactions thoroughly to ensure they work as expected in different scenarios.
Frequently Asked Questions (FAQs)
Q: How do transactions ensure data integrity in PHP?
A: Transactions in PHP ensure data integrity by grouping a series of database operations together as a single unit. If any part of the transaction fails, the entire transaction is rolled back, preventing incomplete or inconsistent changes to the database.
Q: Can I use nested transactions in PHP?
A: PHP does not support true nested transactions. However, you can use savepoints to achieve a similar effect, allowing you to roll back to specific points within the transaction.
Q: What happens if a transaction is not committed or rolled back in PHP?
A: If a transaction is not committed or rolled back explicitly, PHP will automatically roll back the transaction when the script execution ends. This behavior ensures data consistency.
Q: Are there any performance implications of using transactions in PHP?
A: Transactions can have some performance overhead, especially in high-concurrency environments. It’s crucial to keep transactions short and avoid unnecessary locks on resources to maintain optimal performance.
Q: Can I use transactions with all types of databases in PHP?
A: Transactions are supported by most modern databases, including MySQL, PostgreSQL, Oracle, and SQL Server. However, it’s essential to check the specific database’s documentation for transaction support and behavior.
Q: How can I handle large transactions that involve multiple tables?
A: Breaking down large transactions into smaller, more manageable units can help prevent resource contention and improve performance. Consider using savepoints to divide the transaction into logical steps.
Conclusion
Mastering transaction management in PHP is vital for ensuring the reliability and consistency of your database operations. By understanding how to initiate, commit, and roll back transactions, as well as implementing best practices, you can effectively handle transactions in PHP. Remember to use the appropriate isolation levels and handle errors gracefully to maintain data integrity. Whether you’re building a small web application or a complex enterprise system, proper transaction management will contribute to a robust and efficient database-driven application.
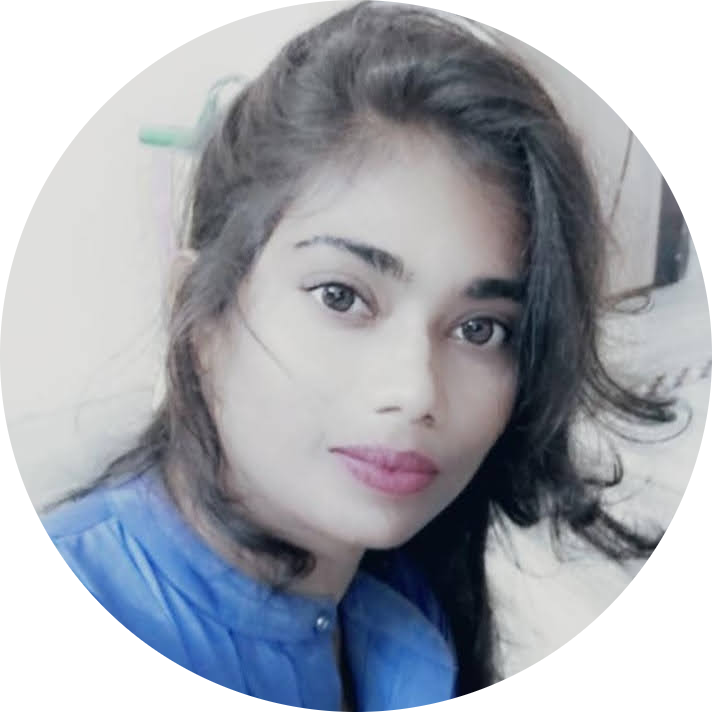
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
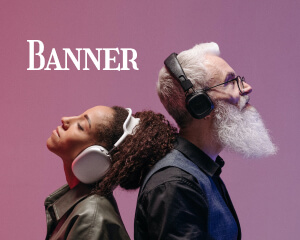