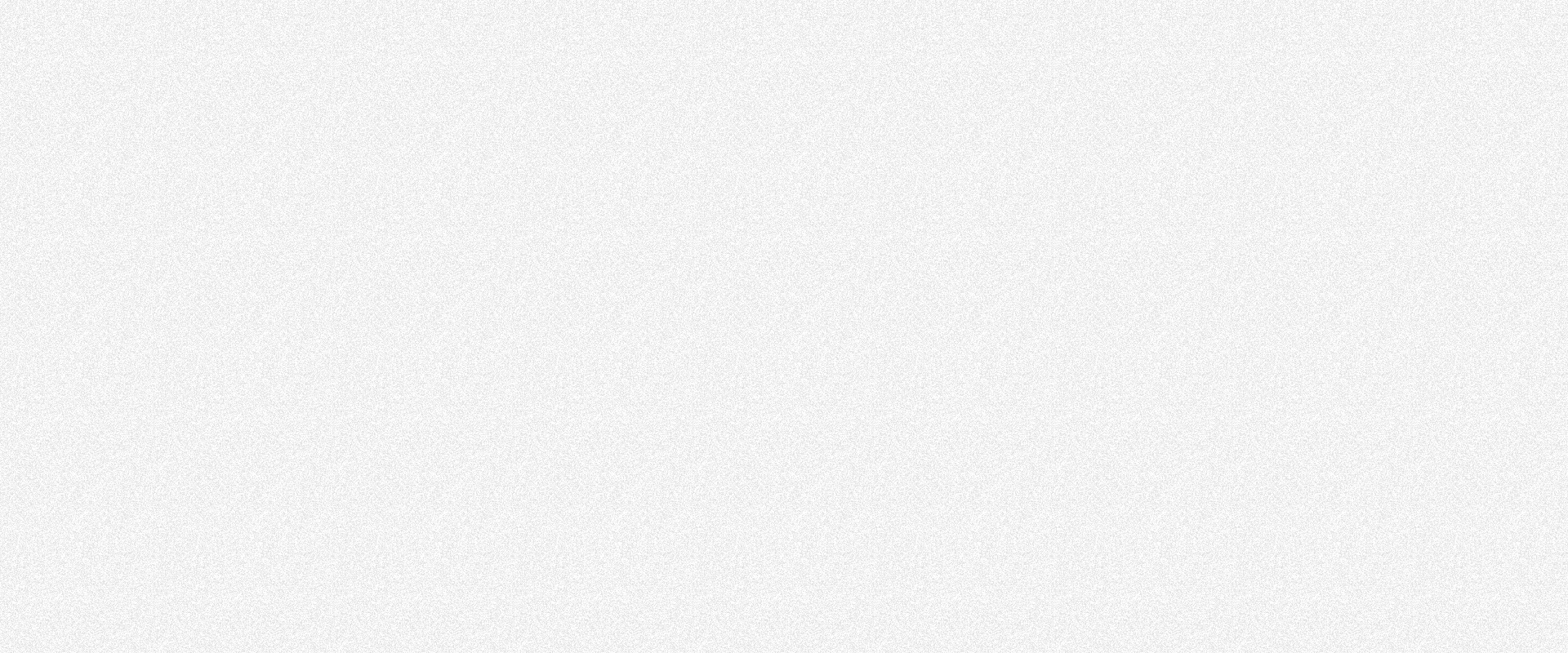
How do you manage cookies in PHP using $_COOKIE?
Cookies play a vital role in web development, enabling websites to store user data and preferences to enhance the user experience. PHP, being a powerful server-side scripting language, provides a convenient way to manage cookies using the $_COOKIE
superglobal array. In this comprehensive guide, we will explore various techniques, best practices, and tips to effectively manage cookies in PHP using $_COOKIE
. Whether you are a seasoned developer or a beginner, this article will equip you with the knowledge and expertise to handle cookies like a pro.
How do you manage cookies in PHP using $_COOKIE?
Understanding the Basics
Before we dive into the nitty-gritty of managing cookies in PHP using $_COOKIE
, let’s first understand what cookies are and how they work. Cookies are small pieces of data stored on the user’s device by the web browser. They are used to retain information about the user’s interactions with a website. PHP’s $_COOKIE
superglobal array allows developers to access and manipulate these cookies effortlessly.
Setting Cookies
To set a cookie in PHP, you need to use the setcookie()
function. The function takes several parameters, including the cookie name, value, expiration time, path, domain, and security options. Here’s an example of setting a cookie to remember the user’s preferred theme:
setcookie('theme', 'dark', time() + (86400 * 30), '/', 'example.com', true, true);
In this example, the cookie name is “theme,” the value is “dark,” it expires in 30 days, and it is valid for the entire domain “example.com.” The last two parameters enable secure and HTTP-only options.
Retrieving Cookies
Once you’ve set a cookie, you can retrieve its value using the $_COOKIE
superglobal array. For instance, to get the value of the “theme” cookie we previously set, you can use the following code:
if (isset($_COOKIE['theme'])) {
$theme = $_COOKIE['theme'];
// Use the theme value for further processing
}
Updating and Deleting Cookies
To update the value of a cookie, you can simply set it again with a new value and an updated expiration time. If you want to delete a cookie, set its expiration time to a past date:
// Update the "theme" cookie value
setcookie('theme', 'light', time() + (86400 * 30), '/', 'example.com', true, true);
// Delete the "theme" cookie
setcookie('theme', '', time() - 3600, '/', 'example.com', true, true);
Handling Cookie Security
Cookie security is crucial to protect sensitive user data. When setting cookies, always enable the secure and HTTP-only options. The secure option ensures that the cookie is only transmitted over HTTPS, preventing it from being exposed during insecure communications. The HTTP-only option restricts the cookie’s access to client-side scripts, reducing the risk of cross-site scripting (XSS) attacks.
Managing Cookie Consent
In many jurisdictions, websites must obtain user consent before setting non-essential cookies. To comply with regulations like GDPR and CCPA, you can implement a cookie consent banner using PHP and JavaScript. The banner should provide users with the option to accept or decline cookie usage.
Handling Cookie Conflicts
In some cases, cookies set by different scripts on the same website may have conflicting names, leading to unexpected behavior. To avoid such conflicts, use unique and descriptive names for your cookies. Additionally, consider using subdomains or specific paths for individual cookies to isolate them from one another.
Creating Persistent User Sessions
Cookies are often used to create persistent user sessions, allowing users to stay logged in even after closing the browser. To achieve this, set a session cookie with a more extended expiration time and store the necessary session data on the server.
Implementing Remember Me Functionality
The “Remember Me” feature is commonly used on login pages to provide users with the option to stay logged in across sessions. To implement this functionality, set a long-lived cookie with a unique token upon successful login. On subsequent visits, check for the presence of this token and authenticate the user accordingly.
Handling Cookie Expiration and Deletion
Expired cookies can clutter the user’s browser, leading to a less-than-optimal browsing experience. Regularly check for expired cookies and delete them from the user’s device using PHP.
Storing JSON Data in Cookies
While cookies are traditionally used to store simple key-value pairs, you can also store JSON-encoded data to manage more complex information efficiently. Use json_encode()
and json_decode()
to convert data between PHP arrays and JSON strings.
Encrypting Cookie Data
For an added layer of security, you can encrypt sensitive cookie data before storing it on the user’s device. Use strong encryption algorithms and securely manage encryption keys to prevent unauthorized access.
Detecting Disabled Cookies
In some cases, users may have disabled cookies in their browsers, preventing your website from functioning correctly. Implement a fallback mechanism to handle situations where cookies are disabled, such as using session-based data.
Managing Cookie Size
Browsers have limitations on the size of cookies they can store. If your cookies exceed these limits, they may not be set or read correctly. Always keep an eye on your cookie sizes and consider using alternative storage mechanisms, such as session variables or local storage, for larger data sets.
Dealing with Cookie Theft
Cookie theft is a serious security concern, as it allows attackers to impersonate users and gain unauthorized access to their accounts. Use secure authentication mechanisms and implement measures like CSRF protection to mitigate cookie theft risks.
Using Cookies for A/B Testing
Cookies are instrumental in conducting A/B testing, where different versions of a webpage are shown to users. You can use cookies to ensure that a user consistently sees the same version during their visit to gather accurate test results.
Implementing Cross-Origin Cookie Sharing
Sometimes, you may need to share cookies between different subdomains or even unrelated domains. To enable cross-origin cookie sharing, set the SameSite
attribute to “None” and ensure the Secure
attribute is set for HTTPS connections.
Debugging Cookie Issues
During development, you may encounter cookie-related issues that need debugging. Use browser developer tools and server-side debugging to identify and resolve any problems.
FAQs
Can I access cookies directly using $_COOKIE
in PHP?
Yes, $_COOKIE
is a superglobal array in PHP that allows direct access to cookies. However, keep in mind that it only contains cookies set in the current request and does not include any cookies set in the response headers.
How long do cookies persist?
The persistence of cookies depends on the expiration time set when creating the cookie. You can set the expiration time to any desired interval, ranging from seconds to years.
Can I store sensitive data in cookies?
While cookies are convenient for storing small amounts of non-sensitive data, it is not recommended to store highly sensitive information, such as passwords or personal identification numbers (PINs), in cookies. Always use secure server-side storage for sensitive data.
How can I delete a cookie?
To delete a cookie, set its expiration time to a past date. This will cause the browser to remove the cookie from the user’s device.
Is it possible to set multiple cookies in PHP?
Yes, you can set multiple cookies by calling the setcookie()
function multiple times with different cookie names and values.
How can I check if a user’s browser supports cookies?
You can use JavaScript to check if cookies are enabled in the user’s browser. If cookies are disabled, you can display a message or use an alternative method for storing data.
Conclusion
Managing cookies in PHP using $_COOKIE
is a fundamental skill for web developers. By understanding the basics of cookies, setting, retrieving, updating, and deleting them, handling security concerns, and employing best practices, you can enhance the user experience and ensure the smooth functioning of your web applications. Always prioritize security and comply with privacy regulations when working with cookies. Now that you have a comprehensive understanding of managing cookies in PHP, go ahead and apply this knowledge to your web development projects.
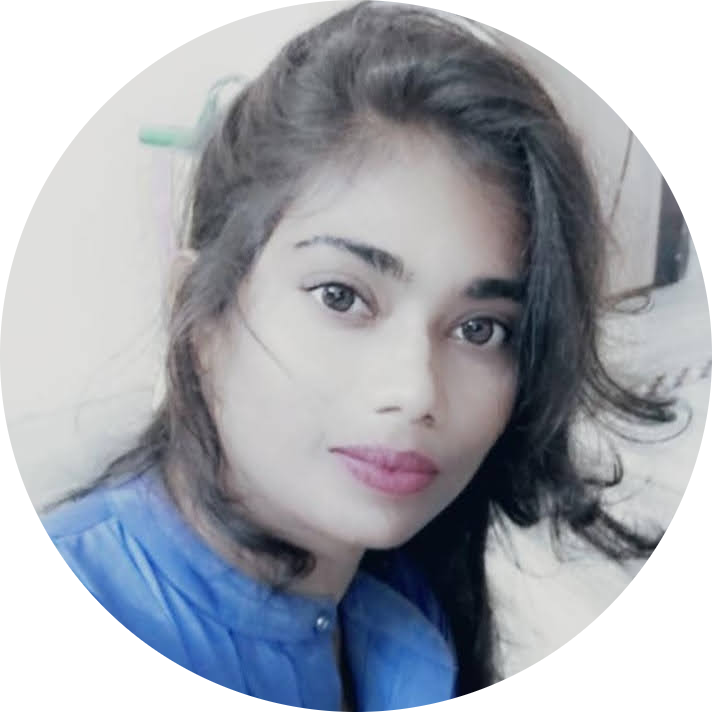
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
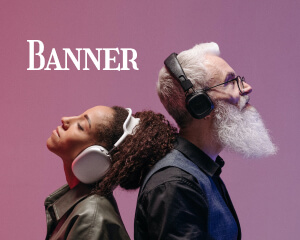