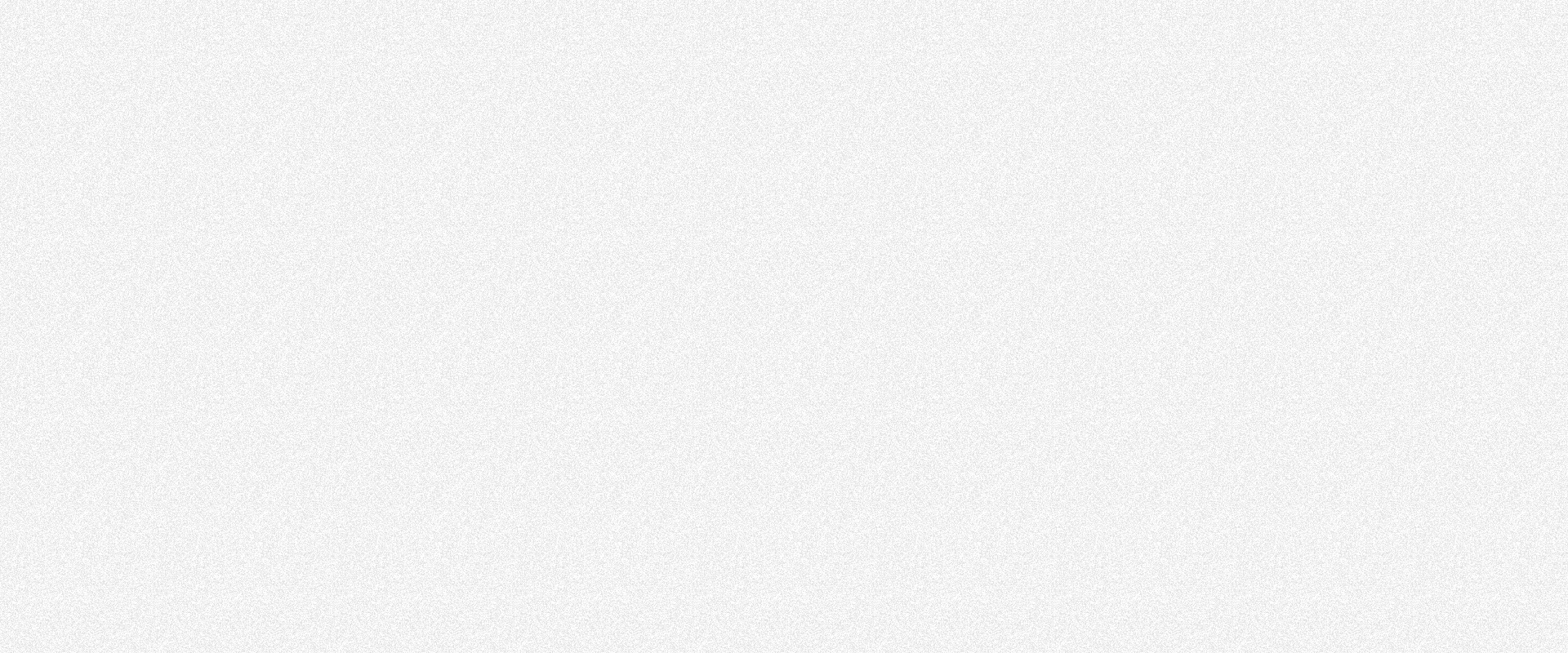
How do you merge two arrays in PHP?
In PHP, merging arrays is a common operation where you combine the elements of two or more arrays into a single array. This can be useful in various scenarios, such as combining data from different sources or adding new elements to an existing array. PHP offers several methods to accomplish array merging, each with its own characteristics and use cases.
Using the array_merge() Function
PHP provides the built-in function array_merge()
to merge two or more arrays. This function accepts multiple arguments, and it returns a new array containing all the elements from the input arrays. It’s important to note that the keys from the input arrays are reindexed in the result.
Merging Indexed Arrays
When merging indexed arrays, the array_merge()
function appends the elements of the second array to the end of the first array. Let’s look at an example:
$firstArray = [1, 2, 3];
$secondArray = [4, 5, 6];
$mergedArray = array_merge($firstArray, $secondArray);
The $mergedArray
will be [1, 2, 3, 4, 5, 6]
.
Merging Associative Arrays
Merging associative arrays with array_merge()
can lead to unexpected results. If both arrays have the same string keys, the values of the second array will overwrite the values of the first array for those keys. Here’s an example:
$firstArray = ['a' => 1, 'b' => 2];
$secondArray = ['b' => 3, 'c' => 4];
$mergedArray = array_merge($firstArray, $secondArray);
The $mergedArray
will be ['a' => 1, 'b' => 3, 'c' => 4]
.
Merging Multidimensional Arrays
array_merge()
is not suitable for merging multidimensional arrays, as it does not handle nested arrays correctly. In such cases, custom solutions are necessary.
Using the array_combine() Function
Another approach to merge arrays is to use the array_combine()
function, which creates an array by using one array for keys and another for its values. The input arrays must have the same number of elements, or a warning will be issued.
$keys = ['a', 'b', 'c'];
$values = [1, 2, 3];
$mergedArray = array_combine($keys, $values);
The $mergedArray
will be ['a' => 1, 'b' => 2, 'c' => 3]
.
Using the “+” Operator
In PHP, you can merge arrays using the “+” operator. Unlike array_merge()
, the “+” operator does not reindex numeric keys. Instead, it keeps the keys of the first array and ignores duplicates from the second array.
$firstArray = ['a' => 1, 'b' => 2];
$secondArray = ['b' => 3, 'c' => 4];
$mergedArray = $firstArray + $secondArray;
The $mergedArray
will be ['a' => 1, 'b' => 2, 'c' => 4]
.
Custom Array Merge Function
For more control over the merging process, you can create a custom function to merge arrays based on specific requirements. This allows you to handle complex cases, such as merging multidimensional arrays or implementing custom logic for duplicate keys.
function customArrayMerge($array1, $array2) {
// Custom merging logic here
// ...
return $resultArray;
}
Dealing with Duplicate Values
When merging arrays, it’s essential to consider how duplicate values should be handled. Depending on the use case, you might want to keep the values from the first array, the second array, or concatenate them into the resulting array.
Handling Numeric Keys
Merging arrays with numeric keys can lead to unexpected results. It’s crucial to ensure that numeric keys are handled appropriately, as they might get reindexed or overwritten during the merging process.
Performance Considerations
When dealing with large arrays, the performance of the merging operation becomes significant. Depending on the size of the arrays and the chosen method, the execution time can vary. It’s essential to select the most efficient method for merging arrays based on your specific use case.
Best Practices for Merging Arrays
To ensure smooth and reliable array merging, consider the following best practices:
- Choose the appropriate merging method based on the type of arrays you are dealing with.
- Handle duplicate values and numeric keys with care, depending on your application’s requirements.
- Test the merging process with various scenarios to ensure its correctness and efficiency.
Conclusion
Merging arrays in PHP is a fundamental operation used to combine data from different sources into a single array. In this article, we explored various methods for merging arrays, including array_merge()
, array_combine()
, and the “+” operator. We also discussed custom array merging functions and best practices to ensure a seamless merging process. By understanding the different techniques and their implications, you can efficiently handle array merging in your PHP applications.
FAQs
- Q: Can I merge more than two arrays at once using
array_merge()
?- A: Yes, you can pass multiple arrays as arguments to
array_merge()
, and it will merge all of them into a single array.
- A: Yes, you can pass multiple arrays as arguments to
- Q: What happens if the input arrays have the same keys when using
array_combine()
?- A: If the input arrays have the same keys, the function will use the values from the first array and ignore the values from the second array.
- Q: Is the “+” operator the same as
array_merge()
in all cases?- A: No, the “+” operator and
array_merge()
behave differently, especially when dealing with associative arrays.
- A: No, the “+” operator and
- Q: How can I handle duplicate values during array merging?
- A: You can define custom logic in a custom merge function to handle duplicate values as per your requirements.
- Q: Are there any performance implications when merging large arrays?
- A: Yes, the performance of array merging can vary based on the method and the size of the arrays. It’s important to choose an efficient method for better performance.
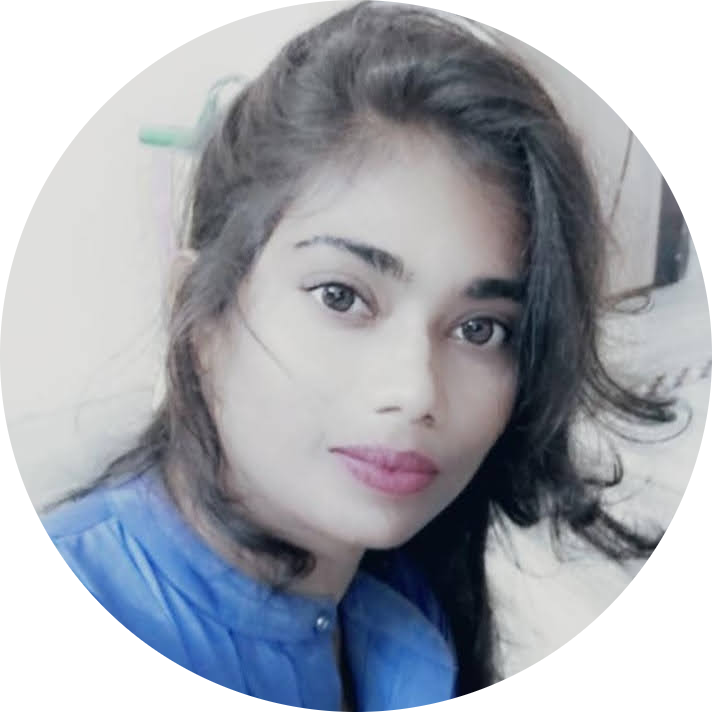
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
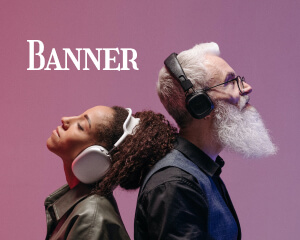