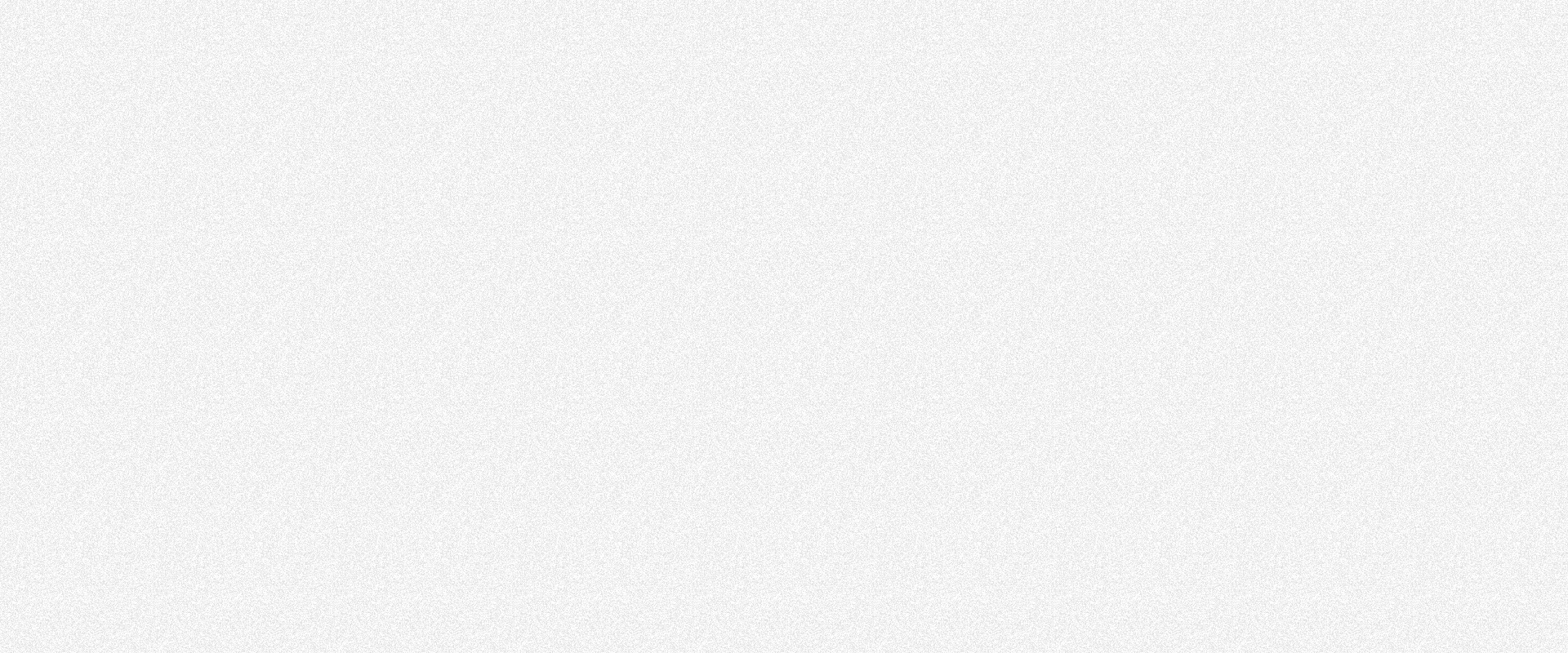
How do you start and destroy a session in PHP?
PHP, a popular server-side scripting language, plays a crucial role in web development. One of its essential features is session management, allowing developers to maintain user-specific data across multiple page requests. Understanding how to start and destroy a session in PHP is fundamental for building interactive and personalized web applications.
In this comprehensive guide, we will walk you through the entire process of creating and handling sessions in PHP. From initialization to termination, we’ll cover the best practices and provide first-hand insights to ensure a seamless user experience.
How do you start and destroy a session in PHP?
Starting and destroying sessions in PHP is a fundamental aspect of web development. Let’s dive into the step-by-step process to initiate and terminate sessions:
1. What is a Session in PHP?
A session in PHP is a way to store and manage user-specific data during a user’s visit to a website. It allows information to be retained across multiple page requests, enabling stateful interactions with users. Sessions are vital for developing dynamic web applications and maintaining user login statuses, shopping carts, and other personalized features.
2. Starting a Session in PHP
To begin a session in PHP, you need to follow these steps:
2.1. Session Start Function
In PHP, you can start a session using the session_start()
function. This function initializes a session or resumes the existing one if it already exists.
2.2. Storing Session Data
Once the session is started, you can store data in the $_SESSION
superglobal array. This associative array allows you to save user-specific information by assigning key-value pairs.
2.3. Common Use Cases
Starting a session is essential for various use cases, such as:
- User Authentication: Sessions help manage user login credentials and permissions throughout their visit to the website.
- Shopping Carts: Sessions facilitate the storage of selected items in a shopping cart during an online shopping session.
- Form Data Persistence: Sessions can be used to retain form data across multiple pages, preventing data loss during navigation.
3. Session ID and Cookies
Behind the scenes, PHP uses a unique session ID to identify users. By default, PHP stores this session ID in a cookie on the user’s browser. This allows PHP to recognize returning users and retrieve their session data.
4. Controlling Session Duration
PHP provides options to control the session duration and behavior using configuration settings. Developers can adjust the session timeout, define session save paths, and specify other session-related parameters.
5. How to Destroy a Session in PHP?
Terminating a session properly is vital for efficient resource management and ensuring data security. Here’s how you can destroy a session in PHP:
5.1. Session Destruction Function
To end a session, you can use the session_destroy()
function. This function removes all session data associated with the current session ID.
5.2. Clearing Session Data
Before calling session_destroy()
, developers can clear specific session variables using unset()
or clear all session data using $_SESSION = array()
.
5.3. Logging Out Users
When implementing user authentication, destroying the session during logout ensures users’ data is cleared, preventing unauthorized access.
6. Best Practices for Session Management
Maintaining session security and efficiency is paramount in web development. Follow these best practices to ensure effective session management:
6.1. Use Strong Session IDs
Generate cryptographically secure session IDs to prevent session hijacking and ensure data privacy.
6.2. Regenerate Session ID
Frequently regenerate session IDs during critical actions (e.g., login, authentication) to minimize the risk of session fixation attacks.
6.3. Store Minimal Data
Avoid storing sensitive information in session variables. Only save essential data needed for user interactions.
6.4. Implement HTTPS
Use HTTPS to encrypt data transmitted during the session, safeguarding it from interception.
6.5. Set Proper Session Timeout
Adjust the session timeout according to the nature of your application to balance security and user convenience.
6.6. Destroy Inactive Sessions
Implement a mechanism to automatically destroy idle sessions after a certain period of inactivity.
FAQs
How long do PHP sessions last?
PHP session duration can be adjusted using the session.gc_maxlifetime
setting in the PHP configuration. By default, a session lasts until the user closes their browser, but you can set a specific timeout period.
Can I use sessions in PHP without cookies?
While PHP primarily relies on cookies to manage session IDs, you can also use URL rewriting to pass the session ID between pages if cookies are disabled. However, this approach has its limitations, and cookies are recommended for a better user experience.
How do sessions improve website performance?
Sessions reduce the need to fetch user data repeatedly from the server, improving website performance by storing data on the client-side.
How do I secure PHP sessions?
To secure PHP sessions, use strong session IDs, regenerate session IDs, avoid storing sensitive data, and implement HTTPS to encrypt data transmission.
Can I destroy a single session variable without ending the entire session?
Yes, you can remove a specific session variable by using the unset()
function, without terminating the entire session.
How do I prevent session hijacking?
To prevent session hijacking, use secure session handling methods, enable HTTPS, and avoid exposing session IDs in URLs.
Conclusion
Understanding how to start and destroy sessions in PHP is a fundamental skill for web developers. Sessions play a crucial role in maintaining user-specific data and creating interactive web applications. By following best practices and employing secure session management techniques, you can ensure a seamless and safe user experience on your PHP-powered websites.
Remember to use strong session IDs, set appropriate timeouts, and encrypt data transmission to protect user information. Implementing these strategies will elevate your web development projects and create trust among your users.
In conclusion, mastering session management in PHP opens up a world of possibilities for crafting personalized and dynamic web experiences.
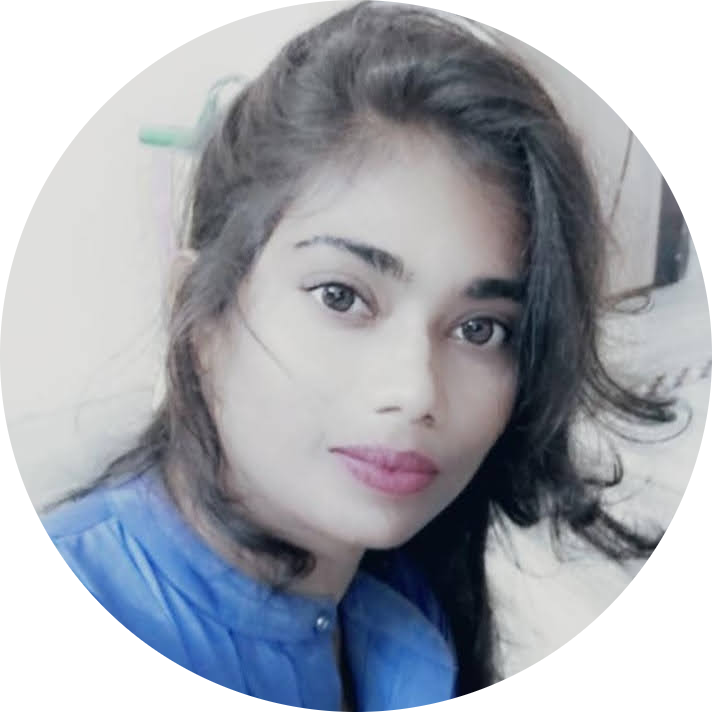
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
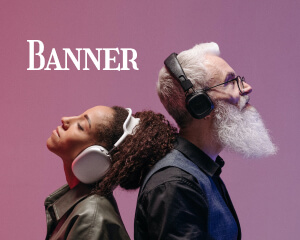