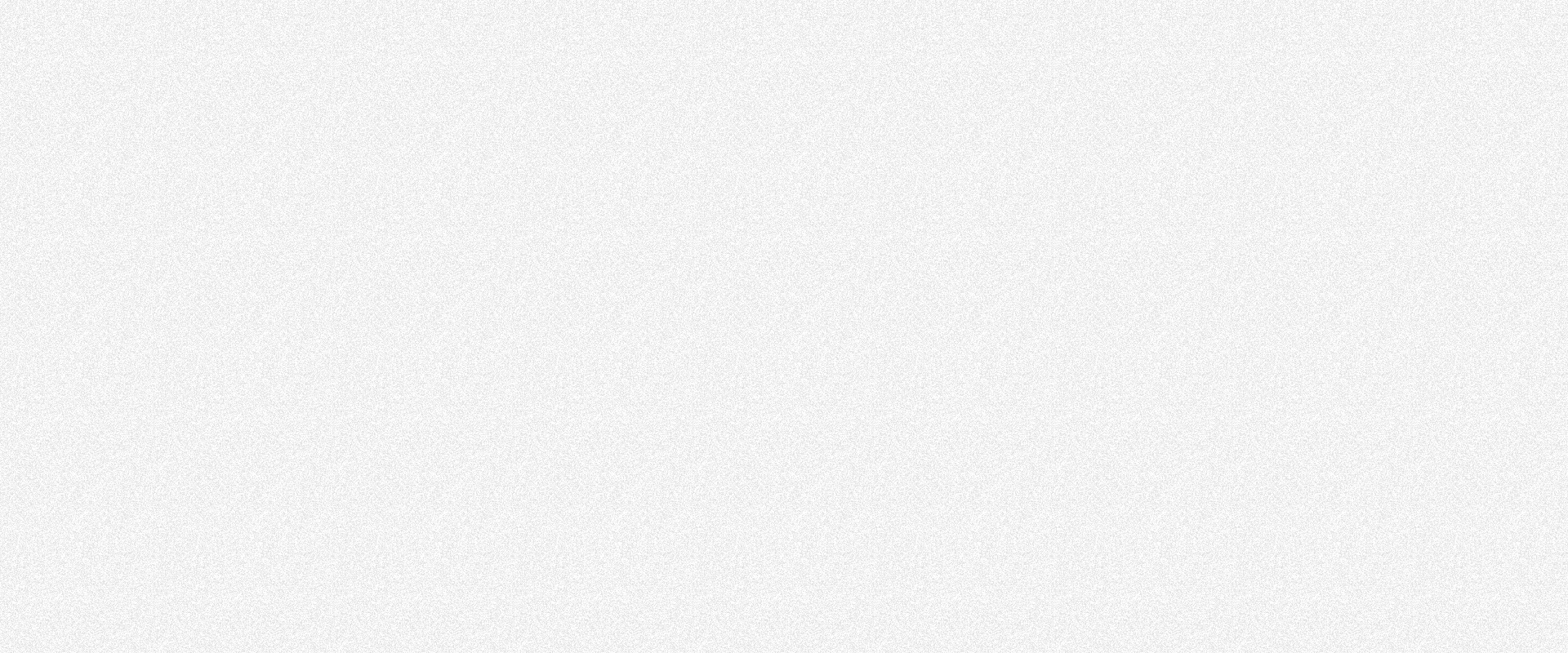
What are multidimensional arrays in PHP?
Arrays are essential data structures in programming languages, including PHP. They allow developers to store and manage multiple values under a single variable name. One of the most powerful variations of arrays in PHP is the multi-dimensional array, which provides a way to organize data in a structured manner, making it easier to handle complex information.
Understanding Arrays in PHP
2.1. What is an Array?
An array is a collection of elements, where each element has a unique index or key associated with it. PHP arrays can hold a mix of data types, such as integers, strings, objects, and even other arrays.
2.2. One-dimensional Arrays
One-dimensional arrays, also known as indexed arrays, are arrays where each element is identified by its numeric index. These arrays are straightforward and commonly used for storing a list of related values.
2.3. Multi-dimensional Arrays
A multi-dimensional array is an array of arrays. It allows you to create a more complex data structure where each element can hold another array or set of values. This nesting can be done to multiple levels, creating multi-dimensional arrays.
Exploring Multi-dimensional Arrays
3.1. Creating Multi-dimensional Arrays
To create a multi-dimensional array in PHP, you can simply nest arrays within arrays. Here’s an example of a two-dimensional array representing a simple table:
$students = array(
array("John", 25, "Male"),
array("Emily", 22, "Female"),
array("Michael", 24, "Male")
);
3.2. Accessing Elements in Multi-dimensional Arrays
To access elements within multi-dimensional arrays, you need to use multiple indices. For instance, to retrieve the age of Emily from the $students
array, you would use:
$age = $students[1][1]; // Result: 22
3.3. Adding and Modifying Elements in Multi-dimensional Arrays
You can add new elements or modify existing ones in multi-dimensional arrays using the appropriate indices. For example:
$students[0][2] = "Non-binary"; // Modifying John's gender
$students[] = array("Alice", 23, "Female"); // Adding a new student
3.4. Traversing Multi-dimensional Arrays
Traversing multi-dimensional arrays requires nested loops to access each element. It’s crucial to use loop structures like foreach
or for
to navigate through the data efficiently.
Practical Use Cases of Multi-dimensional Arrays
4.1. Storing Tabular Data
Multi-dimensional arrays are particularly useful for storing and managing tabular data, such as information from a database query or a spreadsheet.
4.2. Handling Complex Data Structures
When dealing with complex data structures like hierarchical data or trees, multi-dimensional arrays provide an intuitive way to represent and manage the information.
4.3. Working with Nested Data
Nested data, such as JSON or XML responses from APIs, can be efficiently stored and processed using multi-dimensional arrays.
Common Mistakes and Best Practices
5.1. Avoiding Common Errors
Some common mistakes when working with multi-dimensional arrays include incorrect indexing and not handling empty or non-existent values.
5.2. Organizing Multi-dimensional Arrays Effectively
Proper organization of data within multi-dimensional arrays is essential for the readability and maintainability of code.
5.3. Performance Considerations
While multi-dimensional arrays are powerful, they can become unwieldy for extremely large datasets. In such cases, alternative data structures and optimization techniques should be considered.
Conclusion
Multi-dimensional arrays in PHP offer developers a versatile tool for managing complex data structures. They are invaluable for organizing and processing data efficiently. By understanding how to create, access, and traverse multi-dimensional arrays, PHP developers can harness the full potential of this powerful data structure.
FAQs
- Q: Can I have a multi-dimensional array with different data types in PHP? A: Yes, PHP allows multi-dimensional arrays with various data types, offering flexibility in structuring your data.
- Q: How many dimensions can a multi-dimensional array have in PHP? A: PHP supports multi-dimensional arrays with an arbitrary number of dimensions, but it’s essential to strike a balance between complexity and readability.
- Q: Are multi-dimensional arrays memory-intensive? A: Multi-dimensional arrays can consume more memory, especially for large datasets, so it’s crucial to consider performance trade-offs.
- Q: Can I use multi-dimensional arrays for database operations? A: Yes, multi-dimensional arrays are commonly used to handle data fetched from databases, providing an organized way to work with query results.
- Q: Are multi-dimensional arrays exclusive to PHP? A: No, multi-dimensional arrays are a common data structure found in many programming languages, each with its specific syntax and features.
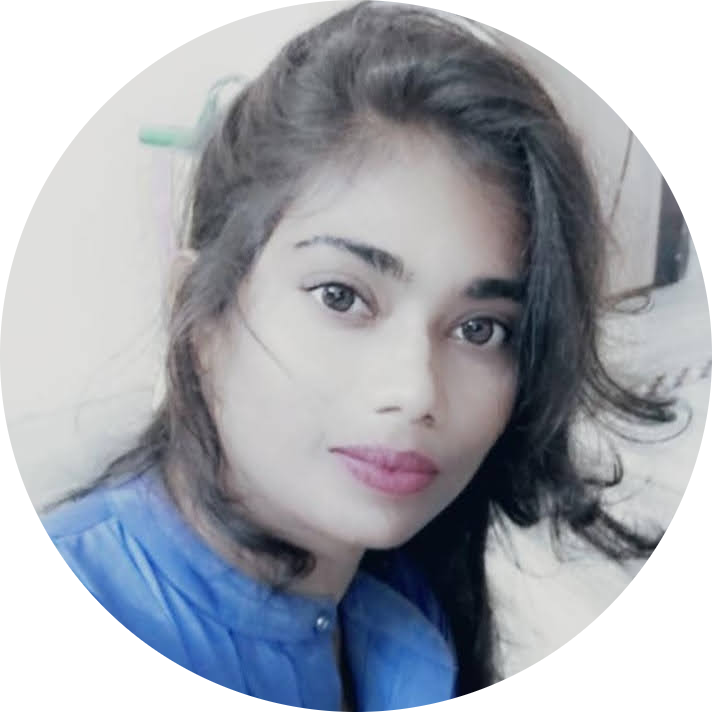
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
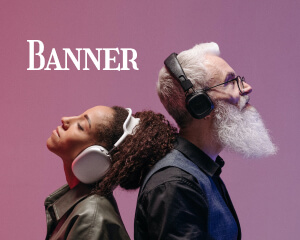