Namespaces are a vital concept in PHP programming that helps developers organize and manage their code more efficiently. They play a crucial role in preventing naming conflicts and making code more maintainable. In this article, we’ll delve into the world of namespaces, exploring what they are and how they effectively ward off naming conflicts in PHP.
Table of Contents
1. Introduction to Namespaces
In PHP, namespaces are a way to encapsulate elements like classes, functions, and constants, allowing developers to organize their code logically. A namespace provides a container that holds related code, preventing clashes between different parts of a large application.
2. The Need for Namespaces
As projects grow in complexity, the chances of naming conflicts increase. Imagine having multiple developers working on different parts of a project, all defining classes with the same name. This can lead to confusion and errors that are hard to debug. Namespaces address this issue by segregating code into separate, clearly defined spaces.
3. Declaring Namespaces
A namespace is declared using the namespace
keyword, followed by the namespace name. This declaration is usually at the beginning of a PHP file and affects all the code within that file.
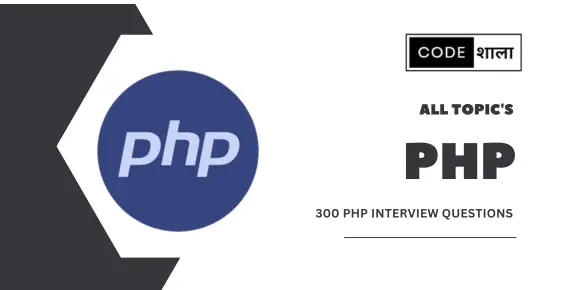
4. Using Namespaced Classes
To use a class within a namespace, you typically include the full namespace path before the class name. For instance, if you have a class named Logger
within the App\Utilities
namespace, you would access it as App\Utilities\Logger
.
5. Alias and Import
Long namespace paths can become cumbersome. PHP allows you to create aliases for namespaces using the use
keyword. This not only shortens the code but also enhances readability.
6. Preventing Naming Conflicts
When classes, functions, or constants share the same name, conflicts arise. By placing related code within a namespace, the chance of conflict diminishes significantly. Namespaces make each class unique within its own namespace.
7. Global Namespace
The global namespace is used for elements that aren’t explicitly placed within a namespace. However, relying on the global namespace is discouraged, as it increases the likelihood of naming conflicts.
8. Best Practices for Using Namespaces
- Choose meaningful and descriptive namespace names.
- Organize your codebase into logical namespaces.
- Avoid relying on the global namespace.
- Use proper indentation and formatting for namespace declarations.
9. Real-world Examples of Namespace Usage
Large frameworks like Laravel and Symfony extensively use namespaces to structure their code. This enables easy integration and minimizes the chances of naming conflicts when combining different packages.
10. Common Mistakes to Avoid
- Not using namespaces and cluttering the global namespace.
- Choosing overly complex or ambiguous namespace names.
- Neglecting to update namespace references after refactoring code.
11. Benefits of Namespace
Namespaces bring several benefits, including:
- Better code organization and maintainability.
- Reduced potential for naming conflicts.
- Clearer code structure, aiding collaboration.
- Easier integration of third-party libraries.
12. Namespaces in PHP 8
PHP 8 introduced improvements to namespaces, including attributes within namespaces and adding namespaces to functions.
13. Future of Namespacing in PHP
As PHP continues to evolve, namespaces will likely play an even more integral role in managing codebases. Their usage will become more standardized and widespread.
14. Conclusion
In the dynamic realm of PHP development, namespaces offer a powerful tool to maintain clarity and prevent naming conflicts. By compartmentalizing code into logical units, developers can streamline collaboration and build more robust applications.
15. Frequently Asked Questions (FAQs)
Q1: Can I define multiple namespaces in a single file? A: Yes, you can define multiple namespaces in one file. However, it’s generally recommended to have one namespace per file for clarity.
Q2: Are namespaces only useful for large projects? A: No, namespaces are beneficial for projects of all sizes. They can help prevent conflicts and improve code organization, regardless of the project’s scale.
Q3: Can I nest namespaces within each other? A: Yes, you can nest namespaces to create a hierarchical structure, reflecting the organization of your code.
Q4: Do namespaces impact performance? A: Negligibly. The impact of namespaces on performance is minimal, and the benefits they provide far outweigh any minor overhead.
Q5: Where can I learn more about PHP namespaces? A: You can find comprehensive information in the official PHP documentation and various online tutorials.