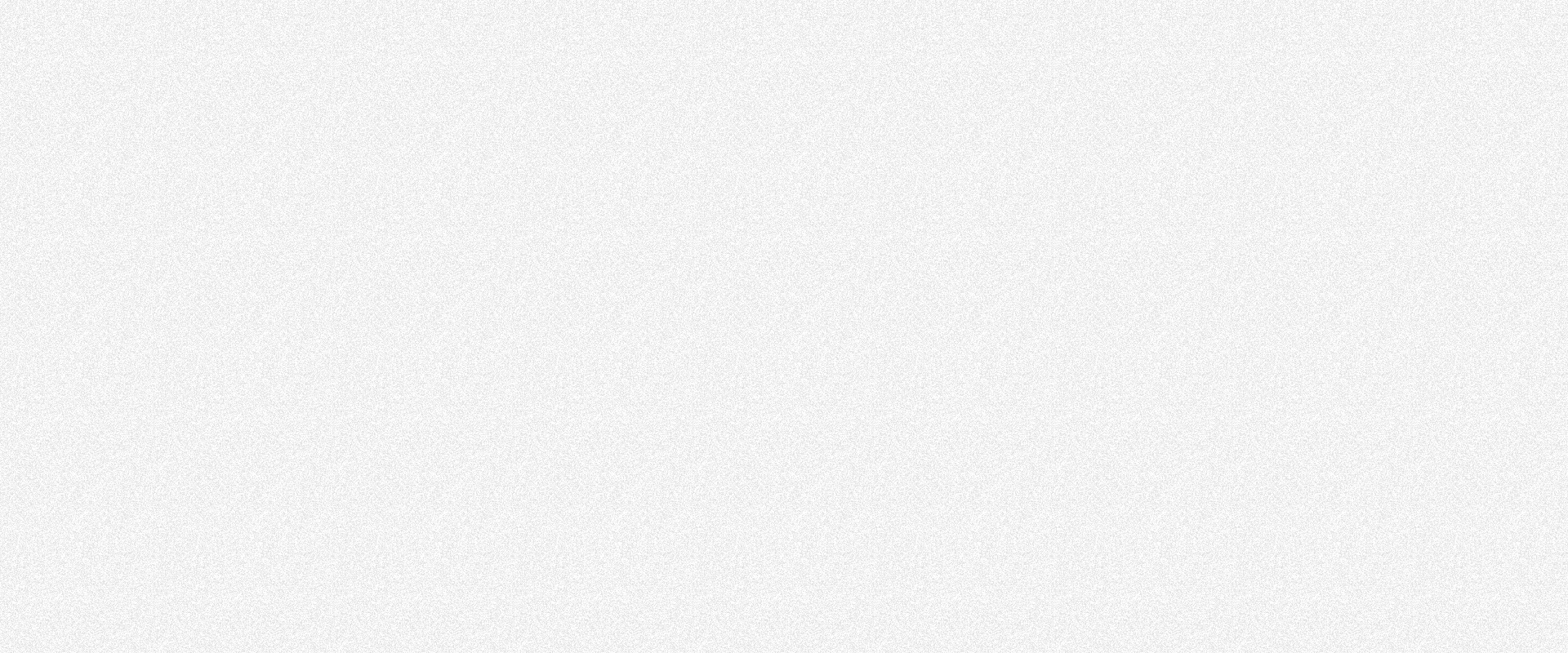
How do you use the for each loop in PHP?
PHP is a powerful server-side scripting language widely used for web development. It provides various loop structures to iterate through data efficiently. One of the essential loops in PHP is the for each
loop, also known as the foreach
loop. In this article, we will explore how to use the for each
loop and its advantages in PHP programming.
Understanding Loops in PHP
2.1 What are Loops?
Loops are programming constructs that enable repetitive execution of a block of code until a specific condition is met. They are invaluable for handling arrays, lists, or any data structure with multiple elements.
2.2 Types of Loops in PHP
PHP offers several loop types, including for
, while
, do-while
, and for each
loops. Each type has its use case and syntax, allowing developers to choose the most suitable loop for their specific requirements.
Exploring the for each
Loop
3.1 Syntax of the for each
Loop
The for each
loop in PHP is specially designed for iterating over arrays. It provides an easy and intuitive way to access each element of an array without the need for explicit indexing. The basic syntax of the for each
loop is as follows:
foreach ($array as $value) {
// Code to be executed for each element in the array
}
3.2 Working with Arrays
Arrays are fundamental data structures in PHP used to store multiple values under a single variable name. The for each
loop simplifies the process of traversing arrays and performing actions on their elements.
Benefits of Using the for each
Loop
The for each
loop offers several advantages over traditional loops like for
and while
. Some of the key benefits include:
- Easy iteration over arrays without explicit indexing.
- Automatic handling of array keys and values, making code concise.
- Prevention of array modification during iteration, ensuring data integrity.
Examples of for each
Loop in PHP
5.1 Example 1: Iterating Over an Array
Let’s begin with a simple example of using the for each
loop to iterate over an array of numbers:
$numbers = [1, 2, 3, 4, 5];
foreach ($numbers as $num) {
echo $num . " ";
}
// Output: 1 2 3 4 5
5.2 Example 2: Working with Associative Arrays
The for each
loop is also suitable for traversing associative arrays:
$student = [
"name" => "John Doe",
"age" => 25,
"course" => "Computer Science"
];
foreach ($student as $key => $value) {
echo $key . ": " . $value . "\n";
}
// Output:
// name: John Doe
// age: 25
// course: Computer Science
5.3 Example 3: Nested for each
Loop
The for each
loop can be used in conjunction with other loops to handle multi-dimensional arrays effectively:
$matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
foreach ($matrix as $row) {
foreach ($row as $element) {
echo $element . " ";
}
echo "\n";
}
// Output:
// 1 2 3
// 4 5 6
// 7 8 9
Tips for Using the for each
Loop Effectively
- Ensure the variable used to store the array is initialized and not empty.
- Avoid modifying the array within the
for each
loop to prevent unexpected behavior. - Use descriptive variable names to improve code readability.
Common Mistakes to Avoid
- Forgetting to use the correct syntax of the
for each
loop. - Attempting to modify the array directly during iteration.
- Not verifying the existence of the array before applying the
for each
loop.
Frequently Asked Questions (FAQs)
8.1 What is the difference between for
and for each
loops in PHP?
The main difference is that the for
loop is used for numerical iteration, whereas the for each
loop is specifically designed for iterating over arrays.
8.2 Can I use the for each
loop with other data structures besides arrays?
No, the for each
loop is exclusive to arrays in PHP.
8.3 How do I break out of a for each
loop prematurely?
You can use the break
statement to exit the for each
loop before its natural end.
8.4 Is the order of elements guaranteed when using the for each
loop?
Yes, the for each
loop preserves the order of elements in the array.
8.5 Can I modify array elements directly while using the for each
loop?
It is not recommended to modify array elements directly within the for each
loop as it may lead to unexpected results.
Conclusion
In conclusion, the for each
loop is a valuable tool in PHP for iterating over arrays efficiently and conveniently. Its simplicity and flexibility make it an essential construct for any PHP developer. By using the for each
loop correctly, you can enhance the readability and maintainability of your code while processing arrays with ease.
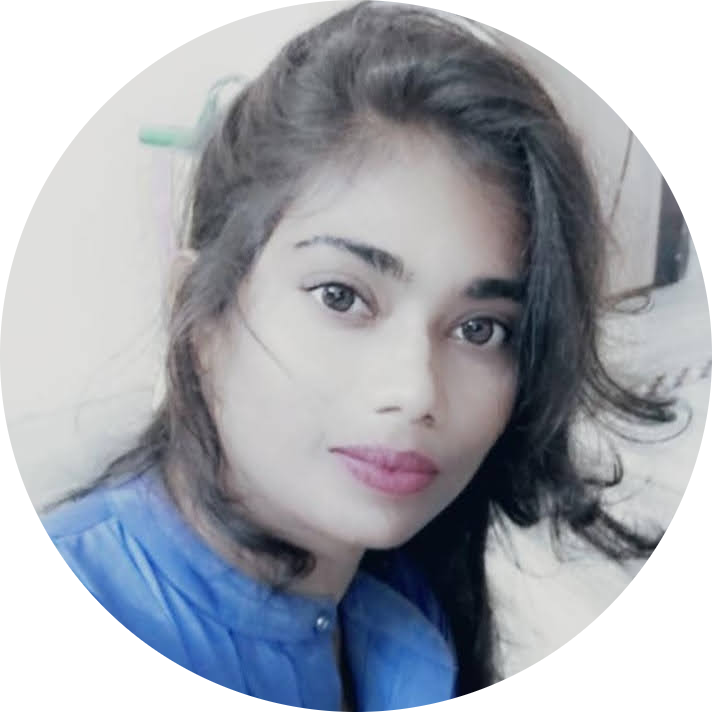
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
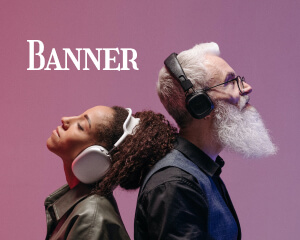