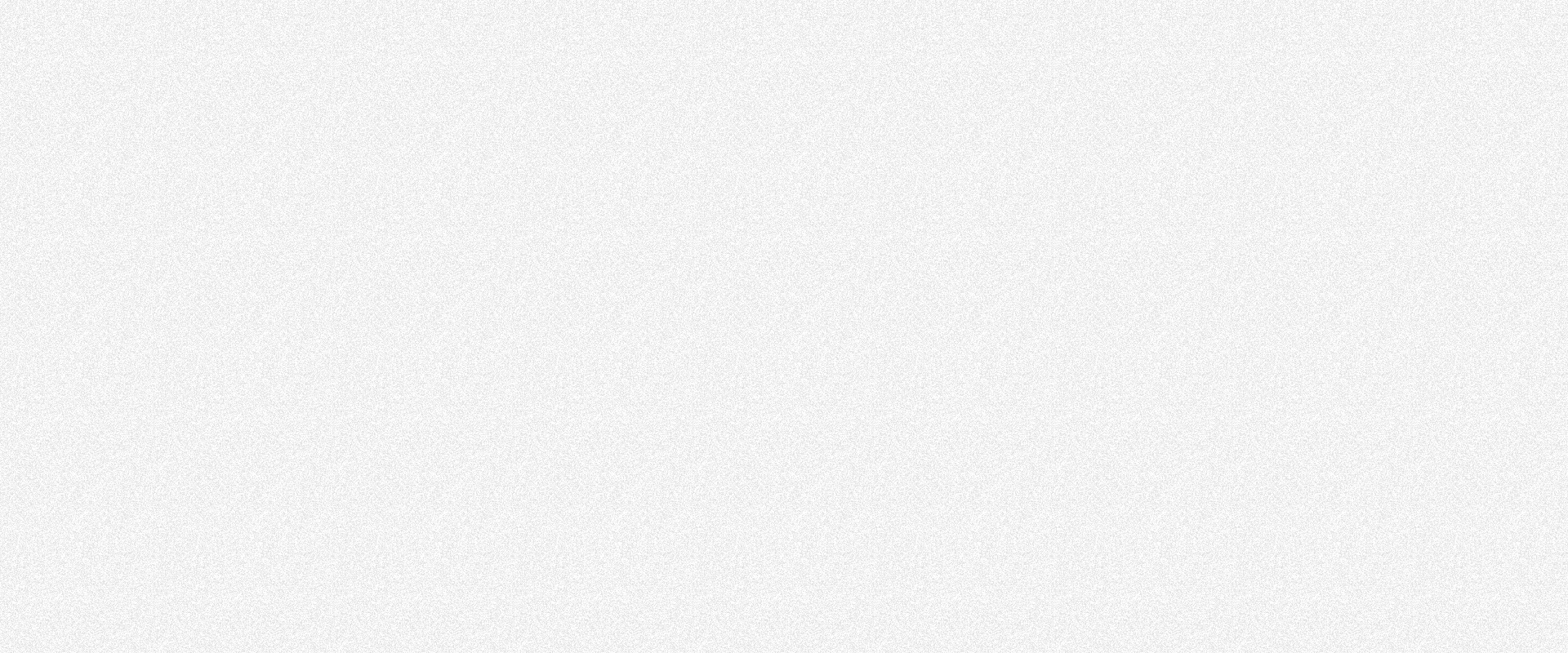
What are variable-length argument lists in PHP functions?
PHP is a versatile and widely used programming language, especially in web development. One of its powerful features is the ability to create functions that can take a variable number of arguments. These are called variable-length argument lists or “variadic” functions.
In this article, we will dive deep into the topic of variable-length argument lists in PHP functions. You will gain a clear understanding of how they function, their practical applications, and best practices for using them in your PHP code. Whether you are a seasoned PHP developer or just getting started, this guide will equip you with valuable knowledge to enhance your coding skills.
What are Variable-Length Argument Lists in PHP Functions?
Variable-length argument lists in PHP functions allow you to pass an indefinite number of arguments to a function. Typically, functions have a fixed number of parameters defined when they are declared. However, variadic functions enable you to create more flexible and adaptable functions that can handle varying amounts of input.
How do Variable-Length Argument Lists Work?
In PHP, you can use the ellipsis (...
) before the last parameter in a function’s parameter list to indicate that it accepts multiple arguments. Within the function, these arguments will be accessible as an array. Let’s take a look at a simple example:
function sumNumbers(...$numbers) {
$sum = 0;
foreach ($numbers as $num) {
$sum += $num;
}
return $sum;
}
$result = sumNumbers(2, 4, 6, 8, 10);
echo $result; // Output: 30
In this example, the sumNumbers()
function accepts multiple arguments (numbers) and calculates their sum.
The Advantages of Using Variable-Length Argument Lists
Variable-length argument lists offer several advantages in PHP development:
- Flexibility: Variadic functions allow developers to create more versatile functions that can handle different numbers of arguments, accommodating a wide range of use cases.
- Code Reusability: By using variadic functions, you can write more generalized code that can be reused in various contexts, reducing code duplication.
- Simplified Code: Instead of defining multiple functions with varying parameter counts, you can use a single variadic function to handle different scenarios.
- Scalability: When working with functions that need to process varying amounts of data, variable-length argument lists provide a scalable solution.
- Ease of Maintenance: Using a variadic function can make your codebase easier to maintain and update in the future.
When to Use Variable-Length Argument Lists?
Variable-length argument lists are beneficial in specific scenarios, such as:
1. Dynamic Number of Inputs
When you have a function that needs to process an unknown or variable number of inputs, using variable-length argument lists can be extremely useful. It allows you to pass any number of arguments without altering the function’s definition.
2. Mathematical Operations
Functions like the one we saw earlier, sumNumbers()
, are excellent examples of using variable-length argument lists. Mathematical operations often involve multiple inputs, and variadic functions can handle this efficiently.
3. Logging and Error Handling
In logging or error handling functions, you might want to log or handle multiple messages or errors passed as arguments. Variadic functions simplify the process by accepting any number of input arguments.
4. Formatting Output
When generating formatted output, you might have functions that require a varying number of parameters. Variadic functions can manage these scenarios without the need for different function declarations.
Best Practices for Using Variable-Length Argument Lists
While variable-length argument lists can be beneficial, using them wisely is essential to maintain clean and readable code. Here are some best practices to consider:
1. Limit Complexity
Avoid creating overly complex functions that rely heavily on variable-length argument lists. Complex functions can be difficult to debug and maintain.
2. Provide Default Values
When defining a variadic function, consider providing default values for optional parameters. This ensures that the function remains flexible and can be called with fewer arguments if necessary.
3. Document Your Code
Properly document the purpose of your variadic functions, including information about the expected arguments and return values. This documentation will be helpful for other developers who use your code.
4. Validate Input
Always validate the input received in a variadic function. Ensure that the data is of the correct type and format before processing it.
5. Be Mindful of Performance
While variable-length argument lists offer flexibility, they may have a slight performance overhead compared to fixed-parameter functions. Be mindful of performance considerations, especially in performance-critical sections of your application.
FAQs
Can a PHP function have both fixed and variable-length argument lists?
Yes, a PHP function can have both fixed and variable-length argument lists. You can define fixed parameters before the variadic parameter, allowing your function to accept a combination of fixed and variable arguments.
Is there a limit to the number of arguments I can pass to a variadic function?
There is no fixed limit to the number of arguments you can pass to a variadic function in PHP. However, keep in mind that excessive use of arguments can impact performance and readability.
Can I pass no arguments to a variadic function?
Yes, you can call a variadic function without passing any arguments. In such cases, the function will receive an empty array as the value of the variadic parameter.
How do I unpack an array into a variadic function?
You can use the ...
operator to unpack an array and pass its elements as separate arguments to a variadic function. For example:
function myFunction($arg1, $arg2, ...$args) {
// Function logic
}
$myArray = [1, 2, 3];
myFunction(...$myArray);
Can I use type hints with variable-length argument lists?
Yes, you can use type hints with variable-length argument lists starting from PHP 7.1. This allows you to specify the expected data type of the arguments passed to the function.
What happens if I change the order of parameters in a variadic function?
Changing the order of parameters in a variadic function does not affect its behavior. The variadic parameter must always be the last one defined, and PHP will automatically handle the argument packing and unpacking.
Conclusion:
Variable-length argument lists in PHP functions are a powerful tool for creating more flexible and adaptable functions. They allow you to pass an indefinite number of arguments, making your code more reusable and scalable. By following best practices and using variable-length argument lists judiciously, you can enhance your PHP coding skills and improve the efficiency of your applications.
Remember, while variable-length argument lists offer great flexibility, using them responsibly and documenting your code is essential for maintaining clean and readable codebases.
Next time you encounter a situation where you need a function to handle varying numbers of inputs, consider using variable-length argument lists and witness the advantages they bring to your PHP development journey.
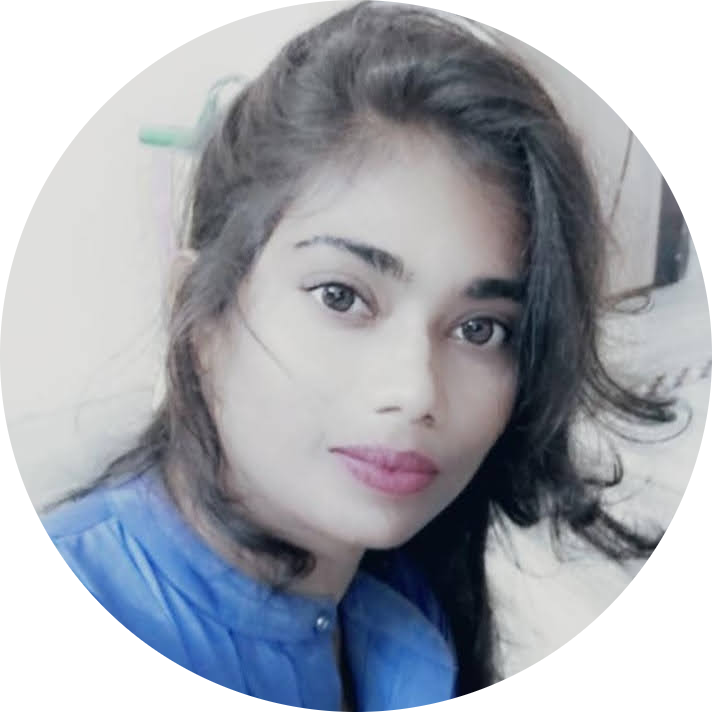
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
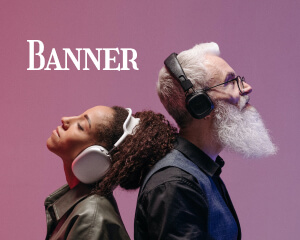