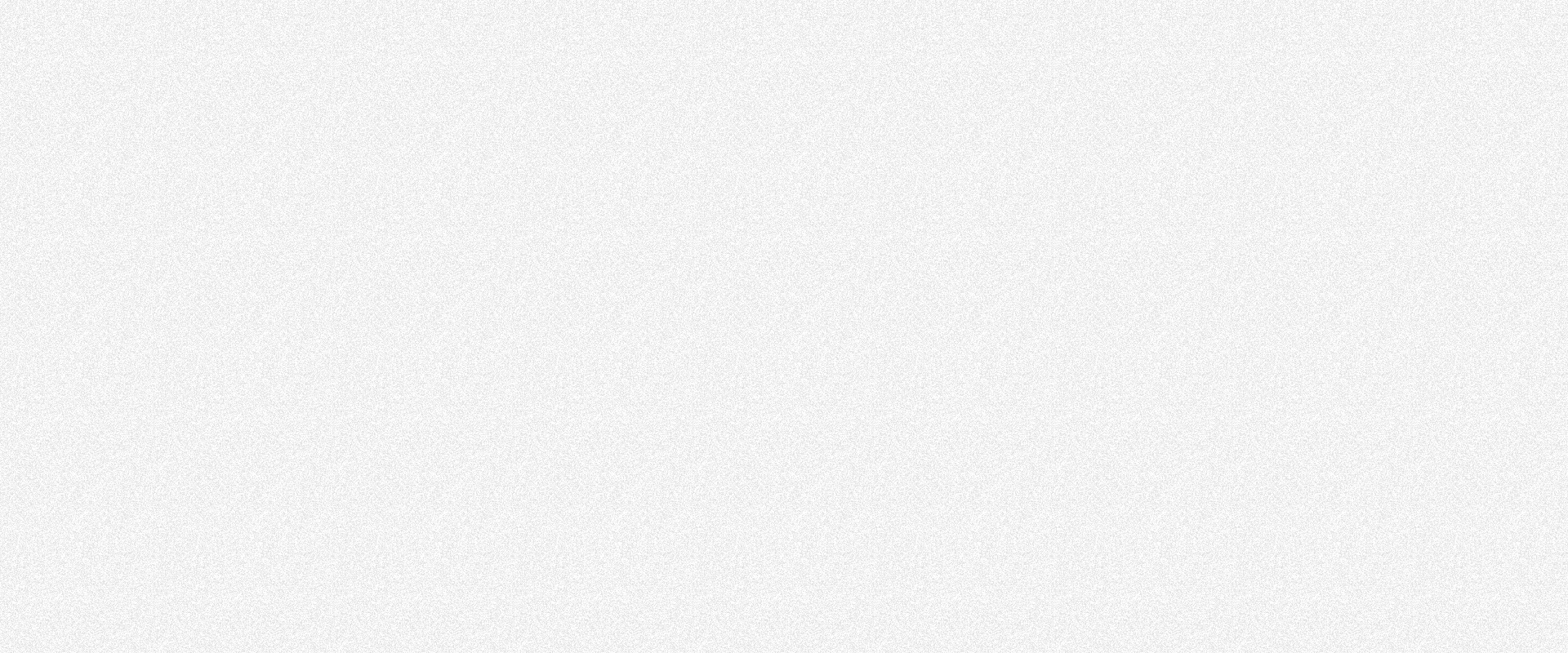
What is the significance of throw statement in PHP?
PHP, being one of the most popular server-side scripting languages, is widely used for web development. While writing code, developers encounter errors and exceptions that need to be handled appropriately. The throw statement plays a crucial role in PHP to handle these exceptions effectively, making code more robust and reliable. In this article, we will explore the significance of the throw statement in PHP, its syntax, usage, and its impact on error handling.
What is the significance of throw statement in PHP?
The throw statement in PHP is a powerful construct used to throw exceptions explicitly. When an unexpected situation arises during the execution of code, developers can throw an exception to indicate that something has gone wrong. This allows developers to manage errors and gracefully handle exceptional cases without interrupting the normal flow of the program.
Understanding Exceptions in PHP
Before diving into the specifics of the throw statement, it’s essential to understand the concept of exceptions in PHP. Exceptions are objects that represent errors or exceptional situations that occur during the execution of code. When an exception is thrown, the normal flow of the program is disrupted, and PHP starts looking for an exception handler to deal with the raised exception.
How to Use the Throw Statement?
To use the throw statement, you first need to create an instance of the Exception class or any custom exception class that extends the base Exception class. Then, you use the “throw” keyword, followed by the created exception object. Let’s take a look at the syntax:
try {
// Code that might raise an exception
if ($some_condition) {
throw new Exception("An error occurred.");
}
// Continue with the normal flow
} catch (Exception $e) {
// Handle the exception here
echo "Caught exception: " . $e->getMessage();
}
Reasons to Use the Throw Statement
The throw statement offers several compelling reasons to use it in your PHP code:
- Error Handling: By using the throw statement, you can explicitly handle errors and exceptional situations in your code, allowing for a more structured and organized approach to error management.
- Custom Exceptions: PHP allows you to define custom exception classes that can carry additional information about the error, making it easier to diagnose and resolve issues.
- Code Readability: The throw statement enhances code readability as it highlights potential exceptional scenarios and the actions taken to handle them, making the code more maintainable.
- Centralized Exception Handling: Throwing exceptions enables centralized exception handling, providing developers with a centralized point to manage and log exceptions.
- Maintainable Codebase: Proper use of the throw statement helps in writing maintainable code, as it encourages developers to address errors at appropriate levels rather than hiding them.
Error Reporting and Exception Handling in PHP
PHP provides robust error reporting mechanisms, but it’s essential to understand the difference between traditional error reporting and exception handling. While error reporting involves displaying errors directly to users, exception handling allows for a more graceful approach, presenting user-friendly messages without revealing sensitive information.
LSI Keyword: PHP Error Handling
PHP Error Handling is closely related to the throw statement as it encompasses various methods to manage and deal with errors in PHP applications.
Handling Multiple Exceptions
In some cases, multiple exceptions may arise during the execution of a code block. PHP offers the “catch” block to handle different types of exceptions. You can use multiple “catch” blocks, each handling specific exception types.
try {
// Code that might raise exceptions
} catch (ExceptionType1 $e) {
// Handle ExceptionType1
} catch (ExceptionType2 $e) {
// Handle ExceptionType2
} catch (Exception $e) {
// Handle other exceptions
}
Dealing with Uncaught Exceptions
When an exception is thrown but not caught by any catch block, it becomes an uncaught exception. In such cases, PHP halts the execution and displays a fatal error. To handle uncaught exceptions, you can use the “set_exception_handler” function to define a custom exception handler.
function customExceptionHandler($e) {
// Handle uncaught exceptions here
echo "Uncaught exception: " . $e->getMessage();
}
set_exception_handler("customExceptionHandler");
LSI Keyword: PHP Exception Handler
The PHP Exception Handler is a vital component that deals with uncaught exceptions and allows you to define custom actions for different exception scenarios.
Exception Best Practices
To make the most of the throw statement and exception handling in PHP, consider the following best practices:
- Specific Exceptions: Use specific exception classes rather than generic ones, as it provides better context and makes the code more maintainable.
- Use Custom Exceptions: Create custom exception classes to differentiate between different types of exceptions and improve error reporting.
- Avoid Silent Failures: Never suppress exceptions without handling them appropriately, as it can lead to difficult-to-trace bugs.
- Log Exceptions: Always log exceptions to track errors and identify potential issues in your application.
- Keep Exception Messages User-Friendly: Exception messages should be informative but not reveal sensitive details about the application’s inner workings.
- Graceful Degradation: Plan for graceful degradation when an exception occurs, ensuring that the application can handle exceptional cases without crashing.
- Test Exception Handling: Regularly test your exception handling code to ensure it works as expected in various scenarios.
Common FAQs
Can I nest try-catch blocks in PHP?
Yes, PHP allows nesting try-catch blocks. You can use this approach when you need to handle exceptions at different levels of code execution.
What happens if I don’t catch an exception?
If an exception is not caught, it becomes an uncaught exception, and PHP halts the script’s execution, displaying a fatal error message.
Should I use the throw statement for all errors in my code?
No, you should use the throw statement for exceptional situations that require immediate attention and handling. For minor errors or warnings, consider using traditional error reporting mechanisms.
Is it mandatory to create custom exception classes?
Creating custom exception classes is not mandatory but highly recommended. It improves code clarity and allows you to create specialized exception types based on different error scenarios.
How can I handle exceptions in a production environment?
In a production environment, you can set up a custom exception handler to log exceptions and display user-friendly messages while keeping sensitive details hidden.
Can I rethrow an exception in PHP?
Yes, you can rethrow an exception within a catch block using the “throw” keyword without any arguments.
Conclusion
The throw statement in PHP is a fundamental feature that enables developers to manage errors and exceptions effectively. By using this powerful construct, you can make your PHP applications more robust, maintainable, and user-friendly. Remember to follow exception handling best practices and use custom exception classes to achieve better code clarity and reliability.
Incorporating the throw statement and exception handling into your PHP projects not only enhances error management but also elevates the overall quality of your code. Embrace the power of the throw statement and write code that gracefully handles exceptional cases, ensuring a seamless experience for your users.
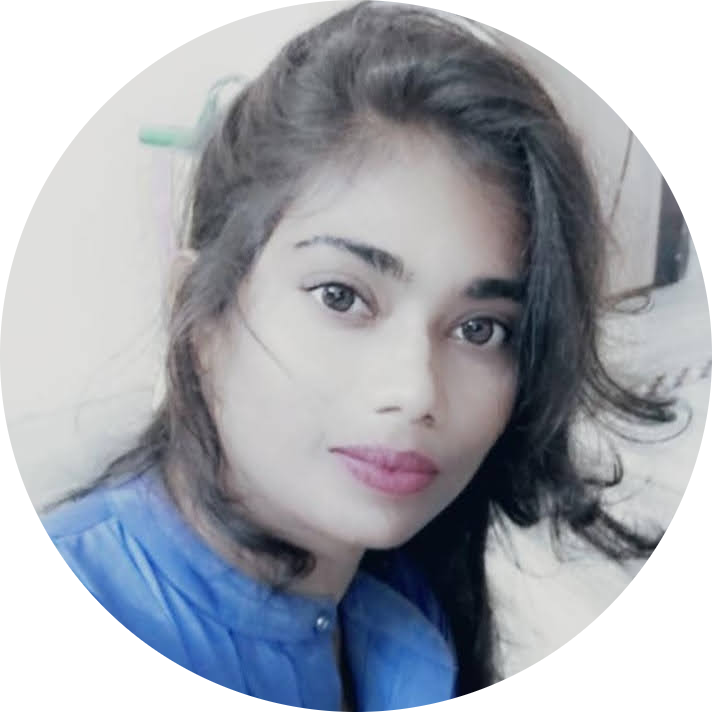
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
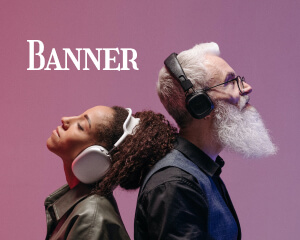