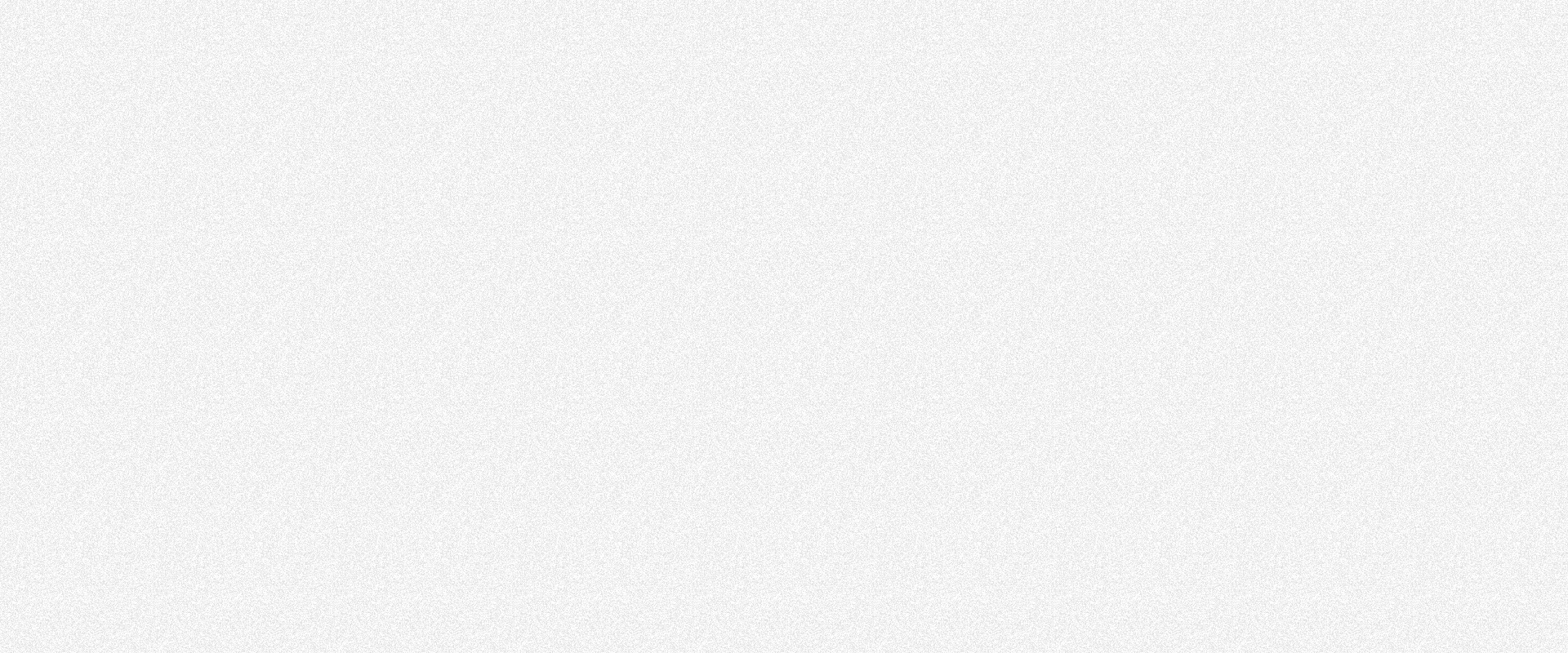
What is the use of the final keyword in PHP classes?
In PHP, the final keyword plays a crucial role in defining class properties. It imparts additional meaning to classes, indicating that certain elements are immutable and cannot be overridden or extended by child classes. Understanding the use of the final keyword is essential for PHP developers seeking to write robust, secure, and maintainable code.
What is the use of the final keyword in PHP classes?
The final keyword is employed in PHP classes to prevent inheritance or extension of specific elements, such as methods and properties, by child classes. When a class or method is marked as final, it becomes unalterable, safeguarding its core functionality from potential modifications that could lead to unforeseen consequences.
Ensuring Method Integrity
When a method is marked as final, it remains unchanged throughout the class hierarchy. This is particularly useful when a class’s behavior should remain consistent, and any attempts to override the method’s implementation by child classes must be restricted.
Restricting Class Inheritance
By declaring a class as final, it becomes an endpoint in the inheritance chain. Child classes can no longer extend or inherit from a final class, ensuring that the final class retains its unique characteristics without any alterations.
Preserving Data Integrity with Final Properties
Using the final keyword for properties prevents them from being redefined or shadowed in subclasses. It ensures that the class maintains control over its data members, reducing the risk of unintentional changes.
Boosting Performance
When certain methods or classes are marked as final, PHP’s internal engine can optimize the code more effectively. It eliminates the need for runtime checks for potential overrides, resulting in improved performance.
Practical Applications of the Final Keyword
The final keyword finds numerous applications in real-world PHP development scenarios, contributing to code stability and preventing unintended modifications. Let’s explore some practical use cases:
Designing Immutable Classes
In scenarios where classes need to remain constant and not subject to inheritance, using the final keyword ensures the immutability of these classes. Immutable classes can be beneficial for data objects and utility classes.
Securing Core Framework Classes
PHP frameworks often have essential classes that should not be altered by developers to maintain consistency and security. Marking these classes as final helps protect the framework’s core integrity.
Ensuring Security in Database Operations
When implementing database connection classes or query builders, certain methods should remain unchanged to avoid potential security vulnerabilities. Marking these methods as final reinforces the security measures.
Implementing Singleton Design Pattern
In singleton design pattern implementations, the final keyword can be used to guarantee that only one instance of a class exists within the application.
The Final Keyword in Action
final class DatabaseConnection {
private $host;
private $username;
private $password;
public function __construct($host, $username, $password) {
$this->host = $host;
$this->username = $username;
$this->password = $password;
}
final public function connect() {
// Connection logic here
}
// Other methods for query execution and data retrieval
}
Frequently Asked Questions (FAQs)
Can a final class be inherited in PHP?
No, a final class cannot be inherited in PHP. Once a class is marked as final, it becomes the endpoint of the inheritance chain, and no other class can extend it.
Can final methods be overridden?
No, final methods cannot be overridden by child classes. They retain their implementation throughout the inheritance hierarchy.
What happens if I try to extend a final class?
If you attempt to extend a final class, PHP will throw an error, preventing the inheritance from occurring.
Can a class have both final methods and final properties?
Yes, a class can have both final methods and final properties. Marking methods or properties as final serves different purposes, but they can coexist in the same class.
Are final classes and abstract classes the same?
No, final classes and abstract classes are opposite concepts. A final class cannot be inherited, while an abstract class is meant to be inherited and cannot be instantiated directly.
Can final classes have abstract methods?
No, a final class cannot have abstract methods. Abstract methods are meant to be implemented in child classes, but final classes cannot have child classes.
Conclusion
The final keyword in PHP classes serves as a valuable tool to ensure code integrity, security, and stability. By using the final keyword, developers can control class inheritance, prevent method overrides, and safeguard essential class properties. Understanding and correctly utilizing the final keyword empowers PHP developers to write reliable and maintainable code, resulting in efficient and secure applications.
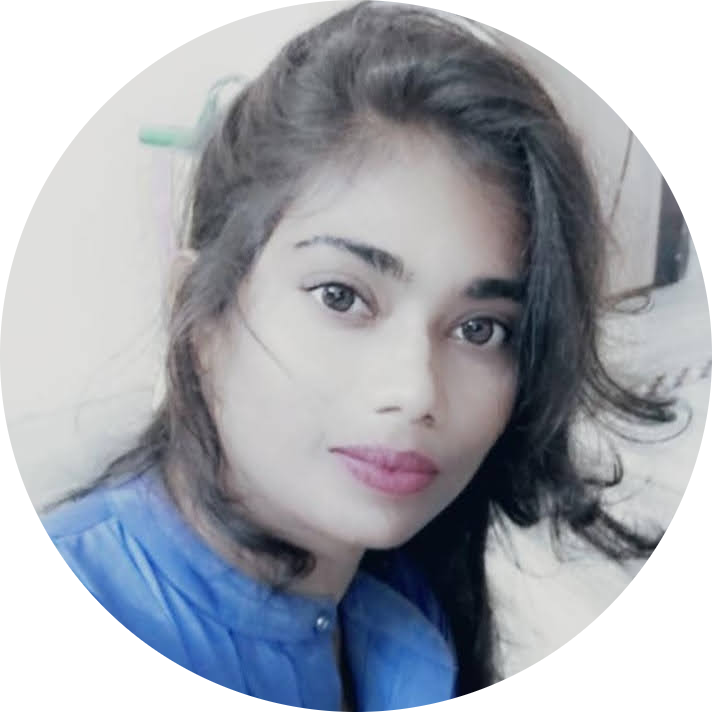
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
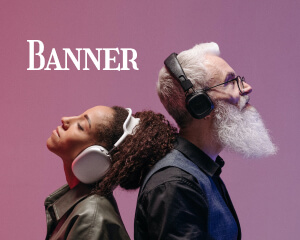