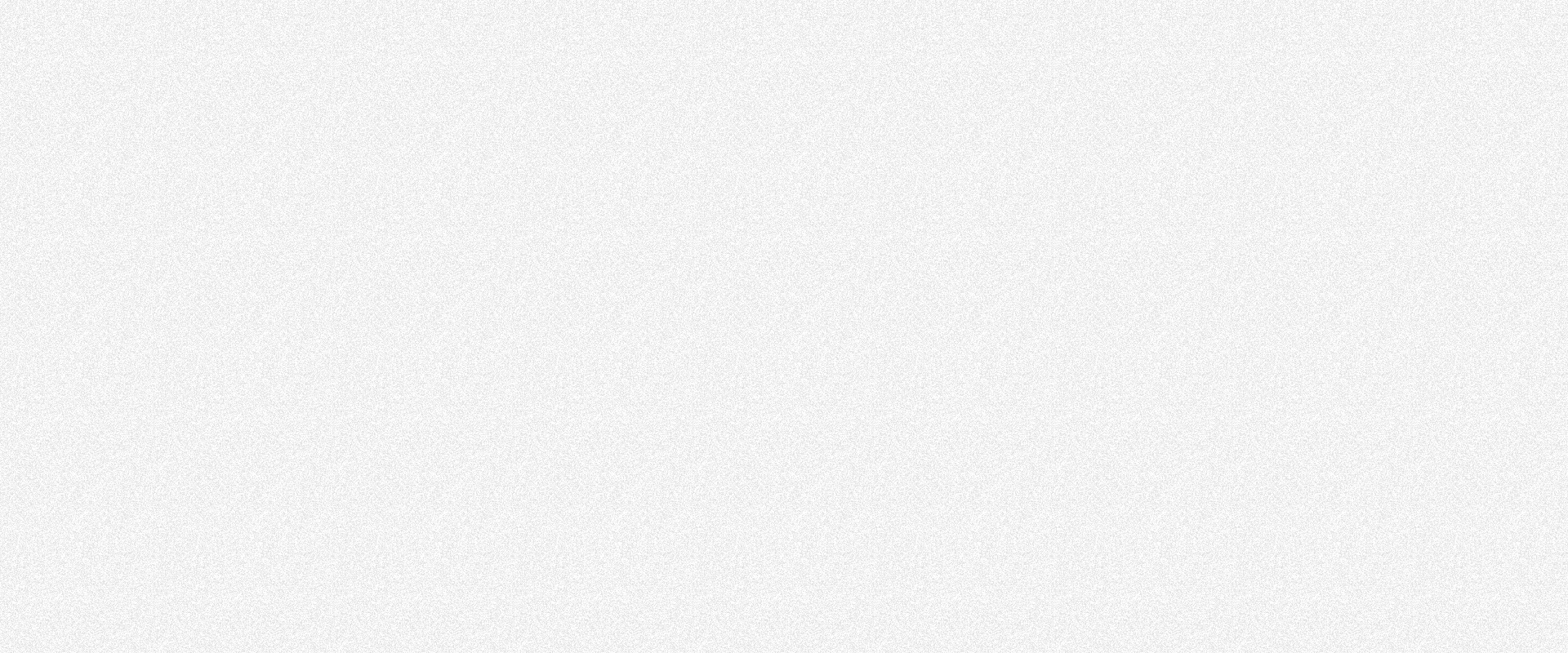
Python topics from basic to advanced
Basic Python Topics:
- Introduction to Python
- What is Python?
- History of Python
- Python 2 vs. Python 3
- Setting up Python
- Installing Python
- Using an Integrated Development Environment (IDE)
- Variables and Data Types
- Variables and Assignments
- Numeric Data Types (int, float)
- Strings (str)
- Lists
- Tuples
- Dictionaries
- Basic Operators
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Control Flow
- Conditional Statements (if, elif, else)
- Loops (for, while)
- Break and Continue statements
- Functions
- Defining Functions
- Parameters and Arguments
- Return Statements
- Scope and Lifetime of Variables
- Data Structures
- Lists, Tuples, and Dictionaries (in-depth)
- List Comprehensions
- Sets
- Stacks and Queues
- File Handling
- Reading and Writing Files
- File Modes
- Working with CSV and JSON
Intermediate Python Topics:
- Object-Oriented Programming (OOP)
- Classes and Objects
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
- Error Handling and Exception Handling
- Try, Except, Finally blocks
- Custom Exceptions
- Modules and Packages
- Importing Modules
- Creating and Using Packages
- The
__init__.py
file
- Functional Programming
- Lambda Functions
- Map, Filter, and Reduce
- Closures and Decorators
- Regular Expressions (Regex)
- Pattern Matching
- Searching and Replacing
- Regex Groups
- Working with Dates and Times
datetime
module- Formatting Dates and Times
- Time Zones
Advanced Python Topics:
- Concurrency and Multithreading
- Threading vs. Multiprocessing
threading
andmultiprocessing
modules- Locks and Semaphores
- Networking and Socket Programming
- Creating Sockets
- TCP and UDP Communication
- Web Scraping
- Database Connectivity
- Connecting to Databases
- SQL Queries with Python
- Object-Relational Mapping (ORM) with libraries like SQLAlchemy
- Web Development
- Introduction to Web Frameworks (e.g., Flask, Django)
- Creating Web Applications
- RESTful APIs
- Machine Learning and Data Science with Python
- Libraries like NumPy, pandas, scikit-learn, TensorFlow, and PyTorch
- Data Visualization (matplotlib, seaborn)
- Machine Learning Algorithms
- Advanced Topics in Python
- Metaclasses
- Decorators with Arguments
- Context Managers (the
with
statement) - Garbage Collection and Memory Management
- Testing and Debugging
- Unit Testing with
unittest
andpytest
- Debugging Techniques
- Profiling Python Code
- Unit Testing with
- Performance Optimization
- Identifying Bottlenecks
- Using Profilers and Performance Tools
- Optimizing Code
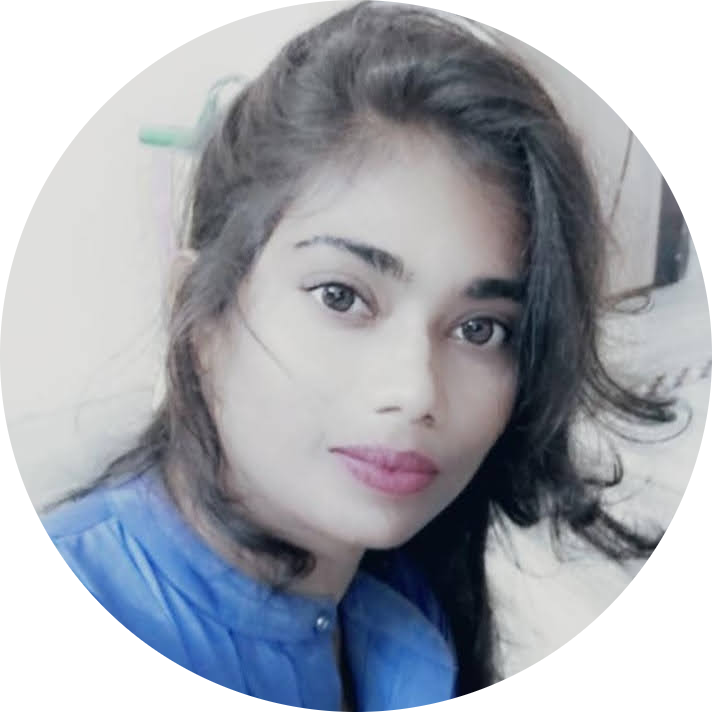
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
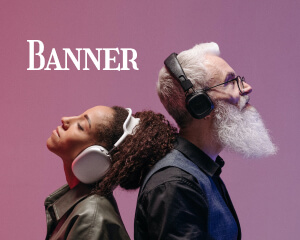