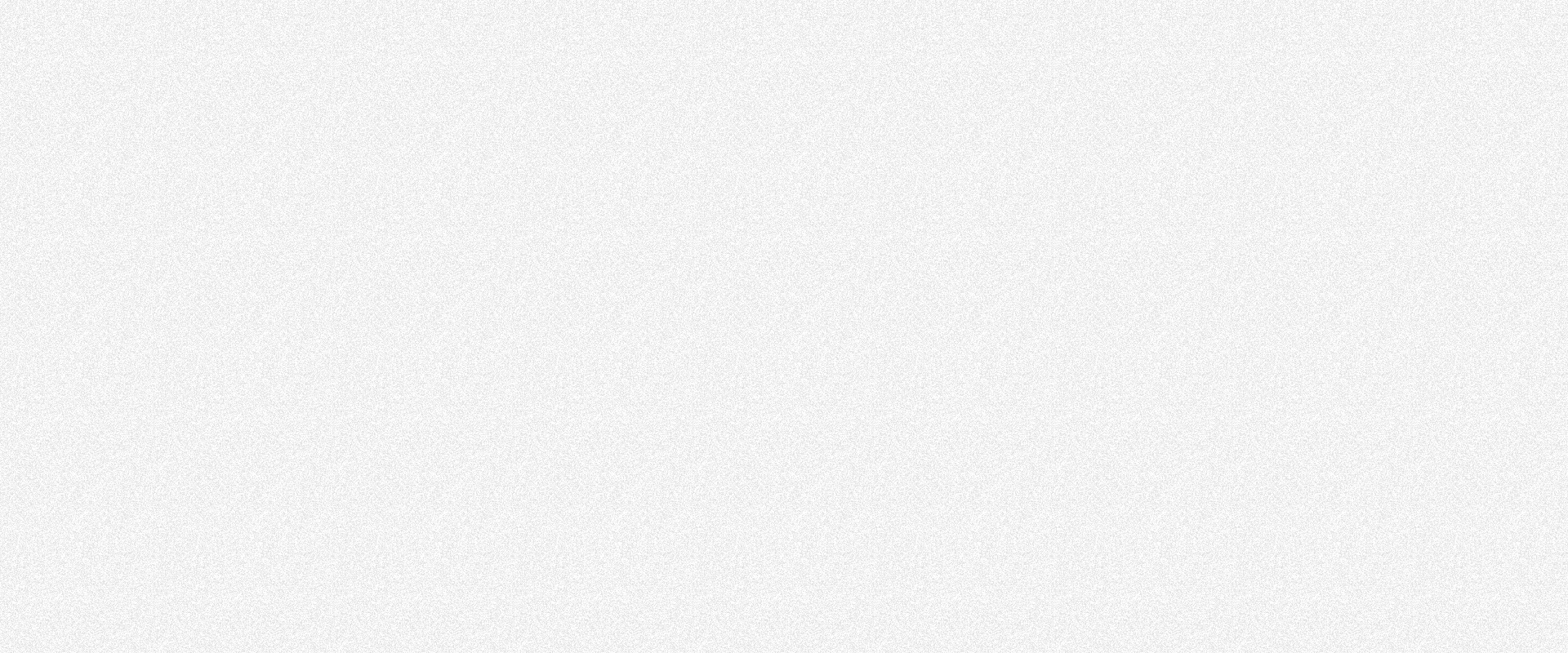
Differentiate between “memo” and “useMemo”.
In the world of React programming, two terms often come up: “memo” and “useMemo.” These terms might sound similar, but they have distinct roles and functionalities in optimizing your React applications. In this article, we’ll delve into the differences between “memo” and “useMemo,” exploring how they can enhance the performance of your applications and make your code more efficient.
Introduction to React Optimization
React is a popular JavaScript library for building user interfaces, known for its component-based architecture. As your application grows, optimizing its performance becomes crucial. This is where techniques like “memo” and “useMemo” come into play. They help prevent unnecessary re-renders and computations, thus enhancing the speed and responsiveness of your app.
Understanding the Purpose of “memo”
“memo” is a higher-order component in React that aims to optimize functional components by preventing unnecessary re-renders. When you wrap a component with “memo,” React checks if the component’s props have changed since the last render. If not, it reuses the previous rendering result, saving valuable resources.
Exploring the Role of “useMemo”
On the other hand, “useMemo” is a hook that focuses on optimizing the performance of individual values computed within a component. It allows you to memoize the result of a computation and reuse it across renders if the dependencies have not changed. This is particularly useful when dealing with complex calculations or expensive operations.
Comparing “memo” and “useMemo”
Performance Optimization
“memo” optimizes entire functional components, preventing them from re-rendering if their props haven’t changed. “useMemo,” on the other hand, optimizes specific values calculated within a component.
Use Cases
“memo” is ideal for optimizing functional components that receive the same props, reducing unnecessary re-renders. “useMemo” is best suited for scenarios where you need to cache the result of a computation to avoid redundant calculations.
Syntax and Implementation
“memo” is a higher-order component that you wrap around your functional component. On the contrary, “useMemo” is a hook that you use within your functional component.
Dependency Handling
“memo” relies on shallow prop comparisons to determine whether to re-render. “useMemo” allows you to specify dependencies, and it re-calculates only when those dependencies change.
When to Use “memo” and “useMemo”
Use “memo” when you want to prevent functional components from re-rendering due to unchanged props. Choose “useMemo” when you need to optimize specific value computations within a component.
Examples of “memo” and “useMemo” in Action
Let’s consider an example of a weather app. If a component displaying weather details doesn’t receive new props, “memo” can help prevent unnecessary re-renders. On the other hand, if the component performs complex calculations, “useMemo” can be employed to avoid recalculating those values on every render.
Tips for Effective Usage
- Use “memo” for optimizing entire components with unchanged props.
- Employ “useMemo” when dealing with expensive calculations.
- Be mindful of overusing “memo” and “useMemo,” as premature optimization can lead to complexity.
Potential Pitfalls to Avoid
- Misusing “memo” can lead to missed updates when props change.
- Incorrectly specifying dependencies in “useMemo” can result in unexpected behaviors.
Conclusion
In summary, both “memo” and “useMemo” are valuable tools in React optimization, but they serve different purposes. “Memo” optimizes entire components by preventing re-renders, while “useMemo” focuses on caching specific computed values. By understanding their roles and using them effectively, you can significantly enhance the performance of your React applications.
FAQs
- What is the main difference between “memo” and “useMemo”?
- “Memo” optimizes entire functional components, while “useMemo” optimizes specific computed values within a component.
- When should I use “memo”?
- Use “memo” when you want to prevent re-renders of functional components due to unchanged props.
- Can I use “useMemo” on class components?
- No, “useMemo” is a hook that is specifically designed for functional components.
- What are the potential downsides of using “memo” excessively?
- Excessive use of “memo” can lead to unnecessary complexity in your code.
- Is it possible for a component to use both “memo” and “useMemo”?
- Yes, a component can use both “memo” and “useMemo” if the optimization scenarios apply.
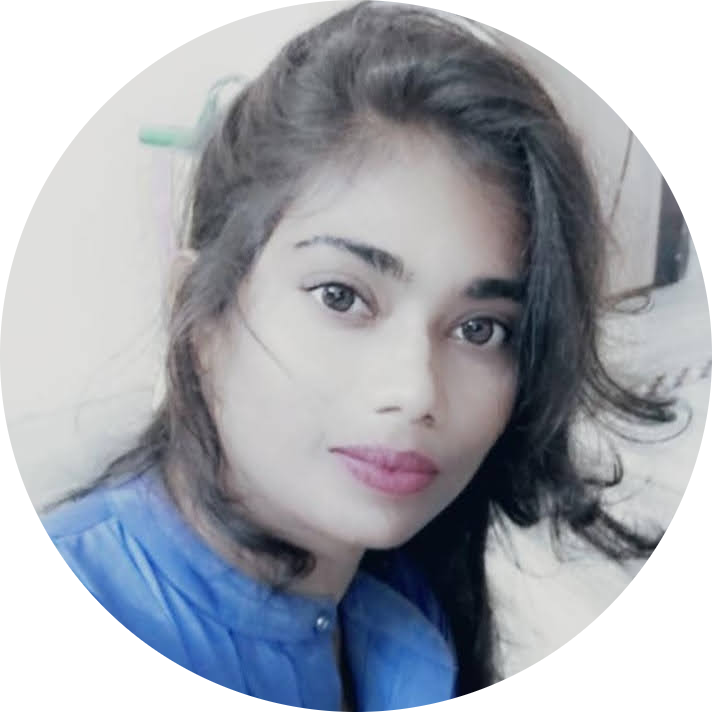
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
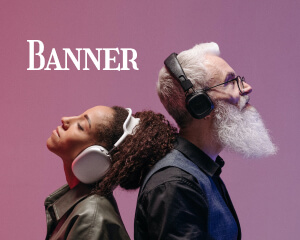