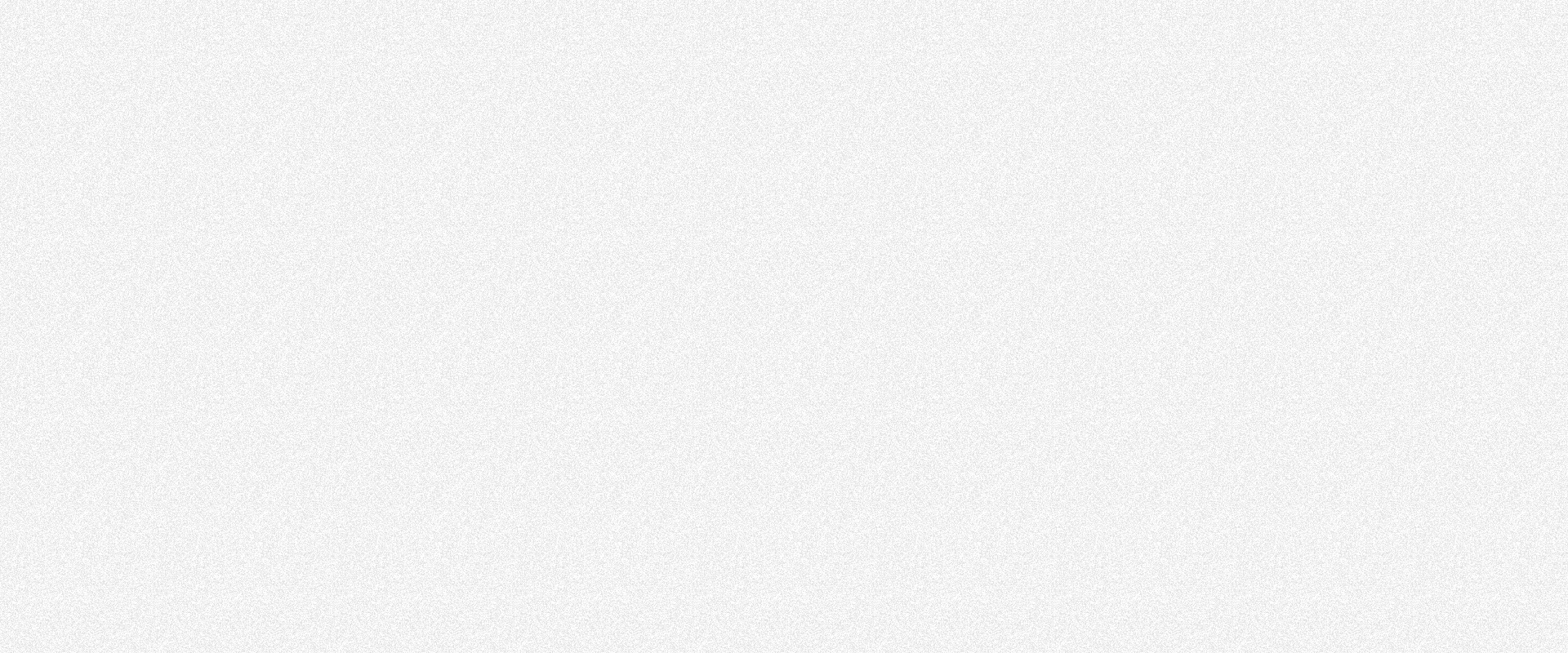
Explain the concept of lazy loading in React.
In the fast-paced world of web development, optimizing the performance of your applications is of paramount importance. One of the techniques that has gained significant traction in recent years is lazy loading. Lazy loading is a strategy that enhances user experience and website performance by loading resources only when they are needed. In this article, we will delve into the concept of lazy loading in the context of React, a popular JavaScript library for building user interfaces.
Introduction to Lazy Loading
Lazy loading is a design pattern used to defer the loading of non-critical resources until they are actually needed. This approach contrasts with eager loading, where all resources are loaded upfront, regardless of whether the user will interact with them. In web development, the primary goal is to reduce initial page load times and improve the overall user experience. This is where lazy loading comes into play.
Why is Lazy Loading Important?
Modern web applications are becoming increasingly complex, incorporating multimedia elements, dynamic content, and intricate components. Eagerly loading all of these resources upfront can lead to slower load times, negatively affecting user engagement. Lazy loading addresses this challenge by loading resources on-demand, which can significantly enhance page performance.
How Lazy Loading Works in React
In the context of React, lazy loading involves splitting your application’s code into smaller chunks called “bundles.” Each bundle contains a specific part of the application. When a user navigates to a particular route or interacts with a component, only the bundle relevant to that action is loaded, reducing the initial load time.
React introduces a built-in method called React.lazy()
that allows you to lazily load components. This function accepts a dynamic import()
statement and returns a new React component. The component is loaded only when it’s required, helping to optimize the application’s performance.
Benefits of Lazy Loading
Lazy loading provides several benefits for both developers and users:
- Faster Initial Load Times: By loading only essential resources initially, the application’s first-time load becomes faster, leading to improved user satisfaction.
- Reduced Data Usage: Users consume less data since only necessary resources are fetched, making the application more mobile-friendly.
- Optimized Performance: Lazy loading prevents unnecessary strain on the user’s device by loading components when they are actually needed.
Implementing Lazy Loading in React Applications
To implement lazy loading in a React application, follow these steps:
- Identify components that are not immediately required on the initial page load.
- Use the
React.lazy()
function to dynamically import these components. - Update your route configuration to use lazy-loaded components.
Common Use Cases for Lazy Loading
Lazy loading is particularly effective in scenarios such as:
- Image Galleries: Loading images only when they come into the viewport.
- Infinite Scroll: Loading additional content as the user scrolls down.
- Modal Windows: Loading modal content when the user clicks to open a modal.
Performance Boost with Lazy Loading
Lazy loading contributes to better performance metrics, including:
- First Contentful Paint (FCP): The browser renders content faster, enhancing perceived load times.
- Time to Interactive (TTI): Users can interact with the application sooner since critical resources load quickly.
- Reduced Bounce Rates: Faster load times lead to lower bounce rates and higher engagement.
Potential Drawbacks and Considerations
While lazy loading offers significant benefits, it’s important to consider:
- SEO: Search engine crawlers may have difficulty indexing lazy-loaded content, affecting search rankings.
- JavaScript Enabled: Lazy loading relies on JavaScript; users with disabled JavaScript may not experience the same benefits.
Lazy Loading vs. Eager Loading
Lazy loading and eager loading serve different purposes:
- Lazy Loading: Delays loading until necessary, optimizing performance.
- Eager Loading: Loads all resources upfront, suitable for smaller applications with fewer resources.
Tools and Libraries for Lazy Loading in React
Apart from React’s built-in React.lazy()
, other libraries like loadable-components
and react-loadable
provide additional features for managing lazy-loaded components.
Tips for Effective Implementation
To maximize the benefits of lazy loading:
- Strategic Selection: Choose components that have a noticeable impact on performance when loaded lazily.
- Test Thoroughly: Ensure lazy-loaded components work seamlessly across different scenarios and devices.
Real-world Examples of Lazy Loading
- YouTube: Loads thumbnail images of videos initially, deferring the loading of actual video players.
- E-commerce Websites: Lazy loads product images as users scroll down, enhancing page load speed.
- News Websites: Delays loading images and ads until they are in the viewport, improving initial load times.
Future of Lazy Loading
As web development continues to evolve, lazy loading will likely become even more integral to creating seamless user experiences. Developers will explore innovative ways to optimize performance without compromising on functionality.
Conclusion
Lazy loading is a powerful technique that significantly enhances the performance of React applications by loading resources only when they are needed. By deferring non-critical resource loading, developers can create faster, more efficient, and user-friendly applications. As the web landscape evolves, lazy loading will remain a fundamental tool for crafting exceptional digital experiences.
FAQs
1. What is the main goal of lazy loading in web development? Lazy loading aims to improve page load times and user experience by loading resources only when they are necessary.
2. Is lazy loading suitable for all types of web applications? Lazy loading is particularly beneficial for applications with complex components, multimedia, and dynamic content.
3. Are there any SEO implications of using lazy loading? Yes, lazy loading can impact SEO since search engines might not easily index content loaded dynamically.
4. Can lazy loading be used with third-party libraries and components? Yes, lazy loading can be applied to third-party libraries and components to optimize performance.
5. How does lazy loading contribute to mobile-friendly websites? Lazy loading reduces data usage and speeds up load times, making websites more accessible and efficient for mobile users.
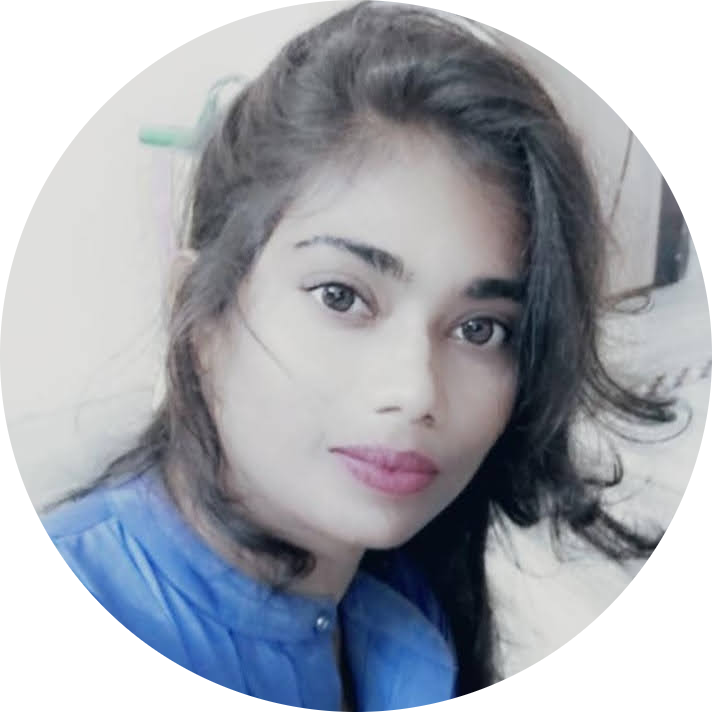
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
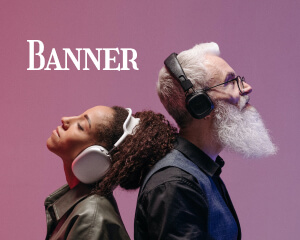