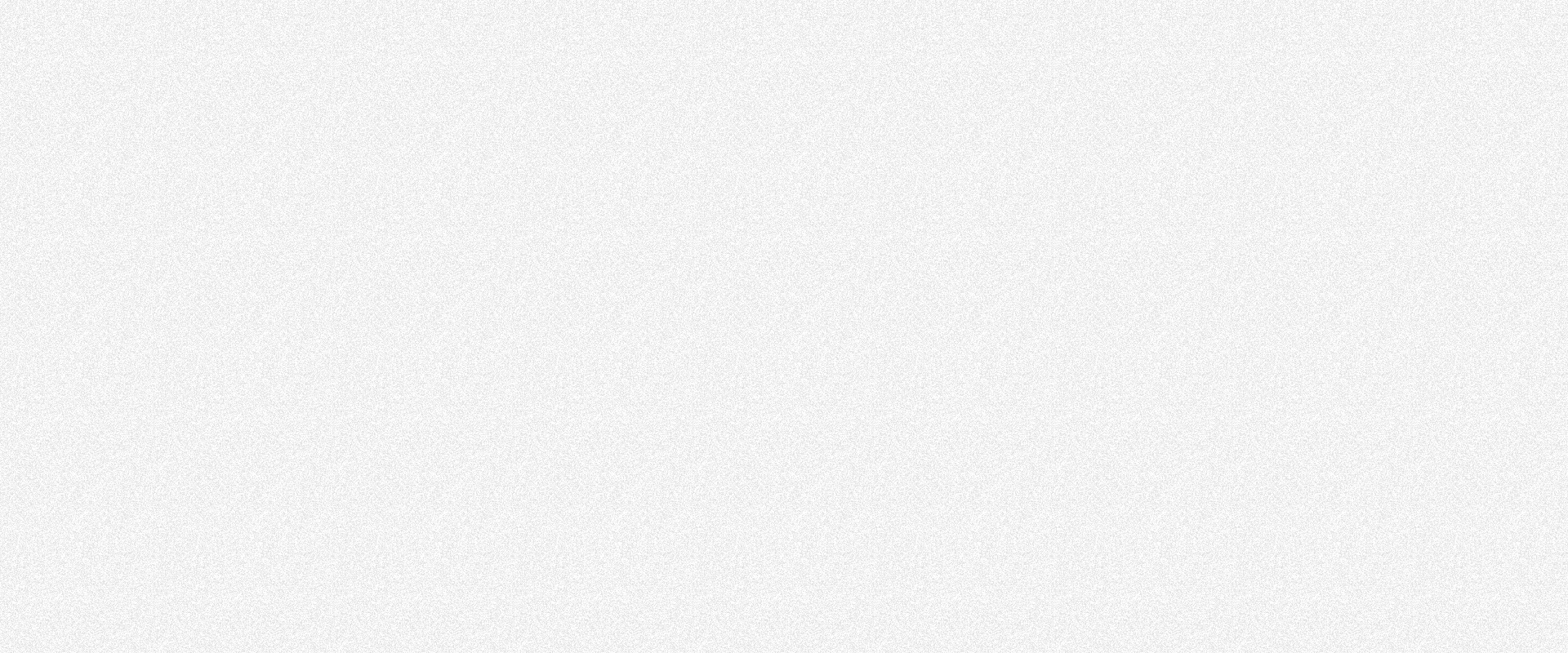
How does React Router work? Explain its core components.
In the world of modern web development, building single-page applications (SPAs) has become a common practice. These applications provide a seamless and fluid user experience by dynamically updating content without requiring a full page reload. React, a popular JavaScript library for building user interfaces, plays a crucial role in this domain. React Router, an essential tool in the React ecosystem, is responsible for handling navigation within SPAs. In this article, we’ll delve into the inner workings of React Router, breaking down its core components to understand how it enables smooth and efficient navigation in React applications.
Introduction to React Router
React Router is a JavaScript library specifically designed to handle routing in React applications. It allows developers to create single-page applications with multiple views while maintaining a coherent and dynamic user experience. React Router abstracts the complexities of managing URLs, ensuring that the application’s state and UI are in sync with the URL.
Installation and Setup
To get started with React Router, you need to install it as a dependency in your project using npm or yarn. After installation, you can import the required components from the React Router library and begin configuring routes.
Route Configuration
Defining Routes
Routes define how different URLs map to different components in your application. By using the Route
component, you can specify which component to render when a particular URL is accessed. This facilitates the dynamic loading of content based on the user’s navigation.
Route Parameters
Route parameters enable the passing of dynamic data through URLs. This is particularly useful for scenarios where you need to display specific information based on user input or selections.
Nested Routes
React Router allows you to create nested routes, meaning that you can render components within components. This is invaluable for creating complex UI structures and organizing your application logically.
Navigation
Link Component
The Link
component provided by React Router is used to create navigation links between different parts of your application. It ensures that the user can move between views without triggering a full page reload.
Programmatic Navigation
Programmatic navigation is essential for scenarios where navigation needs to be triggered based on user interactions or application logic. React Router provides methods that enable you to navigate programmatically.
Route Rendering
Route Matching
React Router uses a process called route matching to determine which component to render based on the current URL. It compares the URL to the defined routes and selects the appropriate component for rendering.
Switch Component
The Switch
component is used to ensure that only one route is matched and rendered at a time. This prevents multiple components from rendering when a URL partially matches multiple routes.
Redirects
Redirects allow you to send users to a different URL if the URL they initially requested doesn’t match any defined routes. This is useful for handling edge cases and providing a seamless user experience.
History Management
Browser History
React Router manages the browser’s history API, allowing you to navigate back and forth between different views using the browser’s back and forward buttons.
Hash History
Hash history uses the URL hash to manage navigation. It’s commonly used when hosting SPAs on static servers that may not have support for URL rewriting.
Memory History
Memory history is primarily used for testing and server-side rendering. It doesn’t interact with the browser’s URL or history but provides the same API for navigation.
Route Guards and Hooks
Route Guards
Route guards are functions that allow you to control access to certain routes. They can be used to implement authentication and authorization logic before rendering specific components.
Lifecycle Hooks
React Router provides lifecycle hooks that you can use to trigger actions when certain events occur during navigation, such as before a route is rendered or when a route is exited.
Handling 404 Errors
React Router enables the creation of a catch-all route that matches URLs not covered by any defined routes. This ensures that users are directed to an appropriate error page when they access invalid URLs.
React Router vs. Traditional Routing
Comparing React Router with traditional server-side routing, it becomes clear that React Router offers a more dynamic and efficient approach. Traditional routing involves requesting new pages from the server, resulting in slower load times and less interactive user experiences.
Conclusion
In conclusion, React Router is a crucial tool for building modern single-page applications with smooth and dynamic navigation. Its core components enable developers to create a seamless user experience by managing URLs, rendering components based on routes, and controlling navigation flow. By leveraging React Router, developers can ensure their applications provide efficient navigation and an engaging user journey.
FAQs
- Is React Router a standalone library? Yes, React Router is an independent library specifically designed for handling routing in React applications.
- Can I use React Router with other front-end libraries? Yes, React Router can be used alongside other front-end libraries and frameworks, enhancing navigation capabilities.
- Is React Router suitable for complex applications? Absolutely! React Router’s support for nested routes and dynamic navigation makes it well-suited for complex applications.
- Does React Router work with server-side rendering? Yes, React Router offers options for server-side rendering, ensuring compatibility with various deployment strategies.
- Where can I learn more about React Router? For more detailed information and documentation, you can visit the official React Router documentation page.
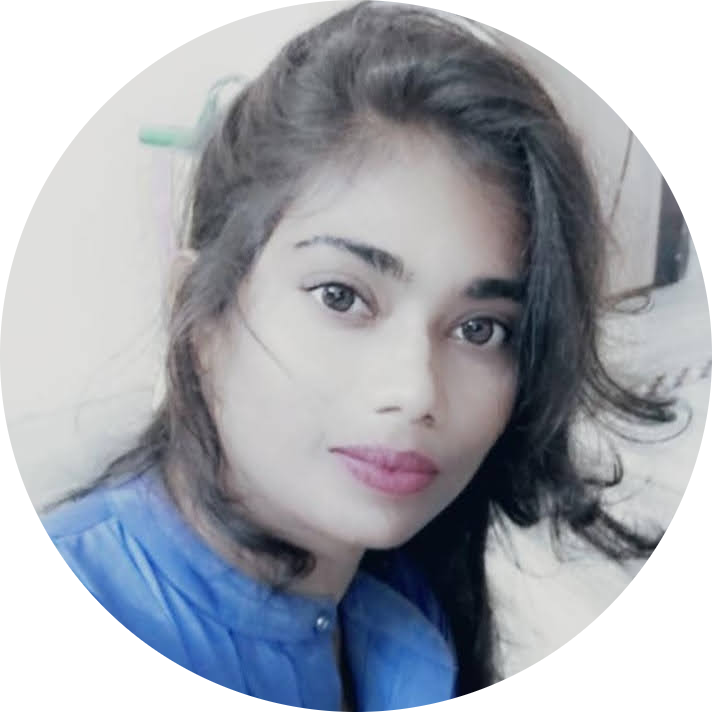
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
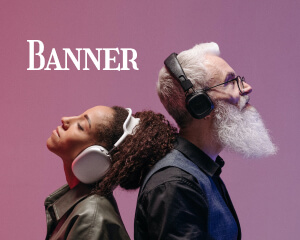