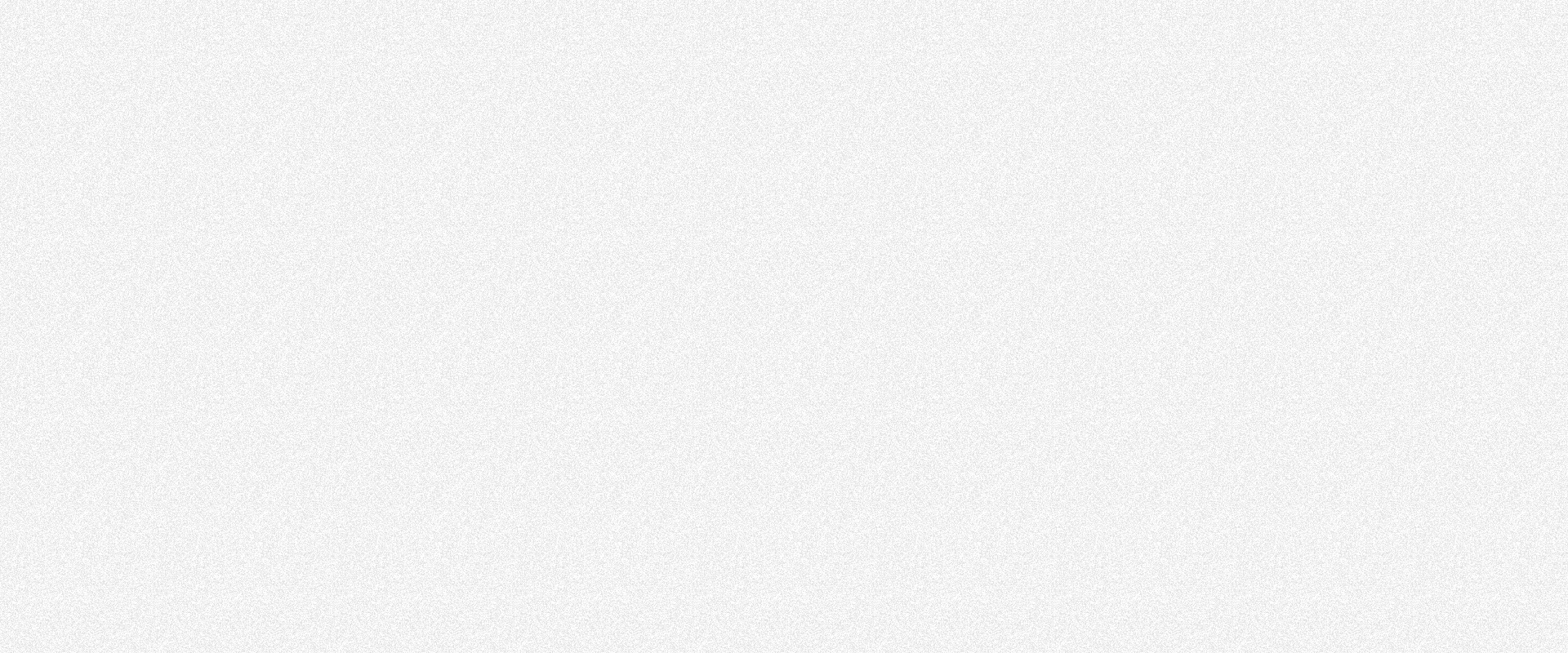
Counter App
Create a Counter App in React.js step by step. In this example, we’ll create a simple app that allows you to increment and decrement a counter value. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
Step 1: Set Up Your Project Open your terminal and follow these commands:
npx create-react-app counter-app
cd counter-app
npm start
This will create a new React app called “counter-app,” navigate you into the project directory, and start the development server.
Step 2: Modify App Component Open the src/App.js
file and replace its content with the following code:
import React, { useState } from 'react';
import './App.css';
function App() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div className="App">
<h1>Counter App</h1>
<div className="counter">
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</div>
</div>
);
}
export default App;
Step 3: Add Styling Open the src/App.css
file and add some basic styling to make the app look better:
.App {
text-align: center;
margin-top: 50px;
}
.counter {
display: flex;
align-items: center;
justify-content: center;
margin-top: 20px;
}
.counter button {
font-size: 20px;
padding: 10px 20px;
margin: 0 10px;
cursor: pointer;
border: none;
background-color: #007bff;
color: #fff;
border-radius: 5px;
}
.counter button:hover {
background-color: #0056b3;
}
Step 4: Run the App With the above code in place, save your files and go back to the terminal. Make sure you’re still in the counter-app
directory. If the development server isn’t running already, start it using:
npm start
Your browser should automatically open, displaying your Counter App. You can now increment and decrement the counter using the buttons.
That’s it! You’ve successfully created a simple Counter App using React.js. You can further customize and expand this app by adding features like maximum and minimum limits, styling improvements, animations, and more.
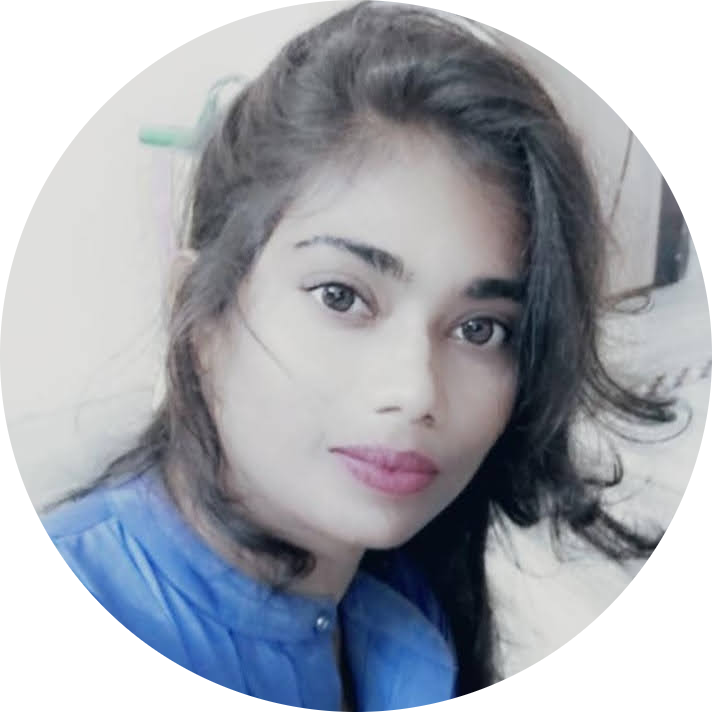
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
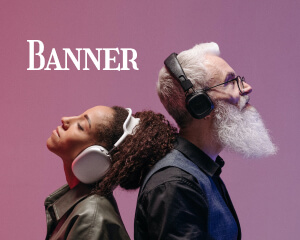