In the fast-paced world we live in, staying organized is paramount to success. Crafting an efficient Todo List app using React JS can be a game-changer in managing your tasks effectively. This guide will walk you through the process, providing you with detailed instructions, code snippets, and valuable insights to create a feature-rich Todo List app tailored to your needs.
How React JS Enhances Task Management
React JS, a popular JavaScript library, is known for its flexibility and robustness in building user interfaces. Leveraging React JS for your Todo List app provides several advantages, including:
- Component-Based Architecture: React’s component-based approach allows you to create reusable UI components, streamlining the app development process.
- Real-time Updates: React’s virtual DOM ensures efficient updates, enabling instant changes without reloading the entire page.
- Interactive User Experience: React’s declarative syntax simplifies UI updates, resulting in a smoother and more responsive user experience.
- Efficient State Management: React’s state management mechanisms enable seamless handling of app data, crucial for a dynamic Todo List app.
Setting Up Your Development Environment
Before diving into the coding process, ensure you have a conducive development environment. Follow these steps:
- Install Node.js: If you haven’t already, download and install Node.js, which comes with npm (Node Package Manager).
- Create a New React App: In your preferred terminal, run the command:
npx create-react-app todo-list-app
. - Navigate to App Directory: Use
cd todo-list-app
to move to your app’s directory. - Start Development Server: Launch the development server by running
npm start
.
Building the Todo List App: Step-by-Step Guide
Designing the User Interface
The first step is creating an appealing UI for your Todo List app. Use HTML and CSS alongside React components to design a visually pleasing interface.
First, create a folder components
inside of src
folder, open the components folder and create TodoItem.js
and TodoList.js
.
Now again open the src folder and open app.js add the below code
import React, { useState } from 'react';
import './App.css';
import TodoList from './components/TodoList';
function App() {
const [todos, setTodos] = useState([
{ id: 1, text: 'Learn React From Codesala', completed: false },
{ id: 2, text: 'Learn Node From Codesala', completed: false },
]);
const [newTodoText, setNewTodoText] = useState('');
const toggleTodo = (id) => {
setTodos((prevTodos) =>
prevTodos.map((todo) =>
todo.id === id ? { ...todo, completed: !todo.completed } : todo
)
);
};
const addTodo = () => {
if (newTodoText.trim() === '') {
return; // Don't add empty todos
}
const newTodo = {
id: todos.length + 1,
text: newTodoText,
completed: false,
};
setTodos((prevTodos) => [...prevTodos, newTodo]);
setNewTodoText(''); // Clear input after adding
};
const deleteTodo = (id) => {
setTodos((prevTodos) => prevTodos.filter((todo) => todo.id !== id));
};
const editTodo = (id, newText) => {
setTodos((prevTodos) =>
prevTodos.map((todo) =>
todo.id === id ? { ...todo, text: newText } : todo
)
);
};
return (
<div className="App">
<h1>Todo List App</h1>
<div className="add-todo">
<input
type="text"
value={newTodoText}
onChange={(e) => setNewTodoText(e.target.value)}
placeholder="Add a new todo..."
/>
<button onClick={addTodo}>Add</button>
</div>
<TodoList
todos={todos}
toggleTodo={toggleTodo}
deleteTodo={deleteTodo}
editTodo={editTodo}
/>
</div>
);
}
export default App;
TodoItem.js
import React, { useState } from 'react';
const TodoItem = ({ todo, toggleTodo, deleteTodo, editTodo }) => {
const [editMode, setEditMode] = useState(false);
const [editedText, setEditedText] = useState(todo.text);
const handleEdit = () => {
setEditMode(true);
};
const handleSave = () => {
if (editedText.trim() === '') {
return; // Don't save empty edits
}
editTodo(todo.id, editedText);
setEditMode(false);
};
return (
<div className={`todo-item ${todo.completed ? 'completed' : ''}`}>
<input
type="checkbox"
checked={todo.completed}
onChange={() => toggleTodo(todo.id)}
/>
{editMode ? (
<input
type="text"
value={editedText}
onChange={(e) => setEditedText(e.target.value)}
/>
) : (
<p>{todo.text}</p>
)}
<button className="edit-button" onClick={handleEdit}>
Edit
</button>
<button onClick={() => deleteTodo(todo.id)}>Delete</button>
{editMode && <button onClick={handleSave}>Save</button>}
</div>
);
};
export default TodoItem;
Managing Task Data with State
React’s state is the key to dynamic app behavior. Manage task-related data using React state, ensuring real-time updates and smooth user interactions.
Adding Task Components
Break down your app’s UI into smaller components, like TaskItem and TaskInput. This modular approach enhances reusability and maintainability.
Implementing Task Addition
Allow users to add tasks to the list using a controlled input component. Capture user input and update the state accordingly.
Enabling Task Deletion
Implement a delete functionality that removes tasks from the list upon user interaction. React’s state management ensures seamless updates.
Incorporating Task Completion
Enhance user experience by implementing task completion functionality. Toggle task completion status while updating the app’s state.
Styling Your Todo List App
Apply CSS to your components to enhance the app’s aesthetics and usability. Consider using CSS frameworks like Bootstrap for faster styling.
Adding Local Storage Support
Implement local storage to persist tasks even after the user closes the app. This ensures data integrity and a seamless user experience.
Testing and Debugging
Thoroughly test your app’s functionality, identifying and resolving any bugs. Utilize React DevTools for efficient debugging.
Deploying Your App
Prepare your app for deployment by creating a production build using npm run build
. Choose a hosting platform like Netlify or Vercel for hassle-free deployment.
Frequently Asked Questions (FAQs)
- How do I start a new React app? To start a new React app, use the command
npx create-react-app your-app-name
in your terminal. Replace “your-app-name” with your desired app name. - What is React’s virtual DOM? React’s virtual DOM is a lightweight representation of the actual DOM. It improves performance by minimizing direct manipulation of the DOM and batch updating changes.
- Can I use other JavaScript libraries with React? Yes, you can. React’s flexibility allows you to integrate other libraries seamlessly to enhance your app’s functionality.
- How can I style my Todo List app? You can style your app using CSS, inline styles, or CSS frameworks like Bootstrap. Apply styles directly to your components to achieve the desired look.
- Is it possible to share my app with others? Absolutely. Once you’ve deployed your app, you can share the live link with others, allowing them to access and use your Todo List app.
- What are the benefits of using a controlled input in React forms? Controlled inputs provide a single source of truth for form data, making it easier to manage and validate user input.
Conclusion
Crafting an efficient Todo List app using React JS is a rewarding endeavor that enhances your task management efficiency. By following this guide, you’ve learned the ins and outs of creating a feature-rich app that streamlines your daily activities. Embrace the power of React JS and transform the way you organize your tasks.
Related posts
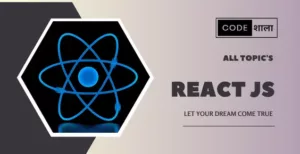
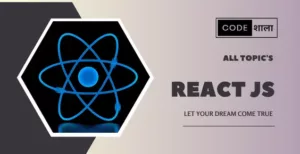