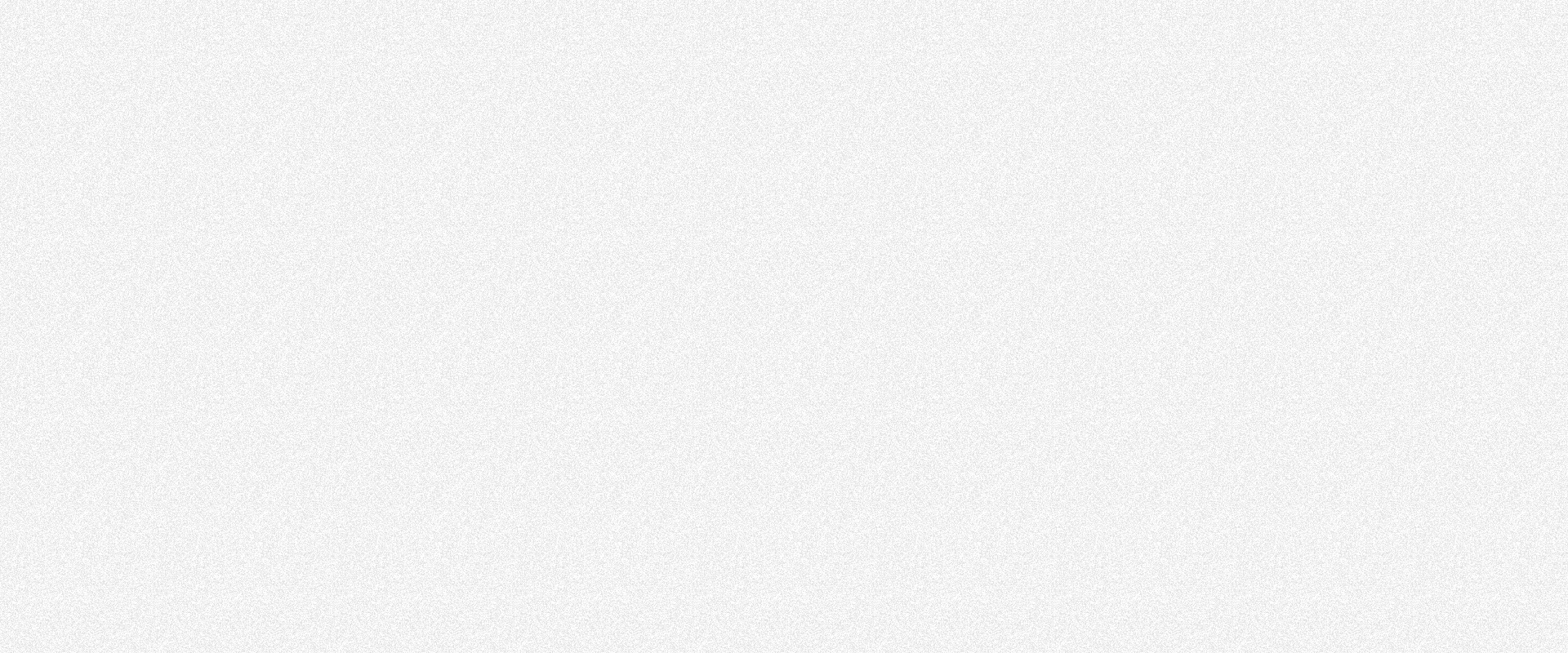
How do you create custom templates for custom post types in WordPress?
If you are a WordPress user and want to display specific types of content differently on your website, custom post types can be a powerful feature. Custom post types allow you to create unique content types with their own distinct attributes. However, just creating custom post types isn’t enough; you also need to know how to create custom templates to display these posts effectively. In this blog post, we will explore how to create custom templates for custom post types in WordPress, including code templates in plain English.
Understanding Custom Post Types
Before we dive into creating custom templates, let’s first understand what custom post types are. In WordPress, by default, you have posts and pages to work with. Custom post types enable you to extend these default types and define your own content types. For example, if you have a photography website, you might want a custom post type called “Gallery” to showcase your images separately from regular blog posts.
Registering a Custom Post Type
To create a custom template, you must first register your custom post type in your WordPress theme or plugin. You can achieve this by adding code to your theme’s functions.php
file or by creating a custom plugin.
function custom_post_type_gallery() {
$labels = array(
'name' => 'Galleries',
'singular_name' => 'Gallery',
'menu_name' => 'Galleries',
'add_new_item' => 'Add New Gallery',
'edit_item' => 'Edit Gallery',
'new_item' => 'New Gallery',
'view_item' => 'View Gallery',
'view_items' => 'View Galleries',
'search_items' => 'Search Galleries',
'not_found' => 'No galleries found',
'not_found_in_trash' => 'No galleries found in Trash',
'all_items' => 'All Galleries',
);
$args = array(
'labels' => $labels,
'public' => true,
'has_archive' => true,
'rewrite' => array('slug' => 'gallery'),
'menu_icon' => 'dashicons-images-alt2',
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('gallery', $args);
}
add_action('init', 'custom_post_type_gallery');
In this code snippet, we’ve registered a custom post type called “Gallery” with various options such as labels, slug, and support for title, editor, and thumbnail.
Creating a Custom Template File
Once you’ve registered your custom post type, you can create a custom template to control how the content of this post type is displayed. To do this, simply create a new file in your theme directory and name it single-gallery.php
. This file will be used by WordPress to display individual gallery posts.
Here’s a simple code template in plain English to get you started:
<?php get_header(); ?>
<div id="primary" class="content-area">
<main id="main" class="site-main">
<?php
while (have_posts()) :
the_post();
// Display the post content here
the_title('<h1>', '</h1>');
the_content();
endwhile;
?>
</main><!-- #main -->
</div><!-- #primary -->
<?php get_sidebar(); ?>
<?php get_footer(); ?>
In this template, we are using standard HTML tags along with PHP functions to display the title and content of the custom post type “Gallery.”
Styling Your Custom Template
To make your custom template visually appealing, you can add CSS styles to your theme’s style.css
file. This will allow you to customize the layout, colors, fonts, and other design elements for your custom post type.
Here’s an example of adding some basic styles:
/* Custom post type "Gallery" styles */
.single-gallery {
padding: 20px;
border: 1px solid #eaeaea;
border-radius: 5px;
background-color: #f7f7f7;
}
.single-gallery h1 {
color: #0073aa;
}
.single-gallery img {
max-width: 100%;
height: auto;
}
Using Plugins for Custom Templates
If you find coding custom templates a bit challenging, there are plugins available that can simplify the process. One popular plugin is “Custom Post Type UI,” which allows you to create custom post types and taxonomies through a user-friendly interface, without writing any code.
Conclusion
Custom post types are a fantastic way to organize and present your content in WordPress. By creating custom templates, you can ensure that your content is displayed exactly how you want it. With the code templates provided in this blog, you can easily get started with your own custom post types and templates, showcasing your unique content to the world.
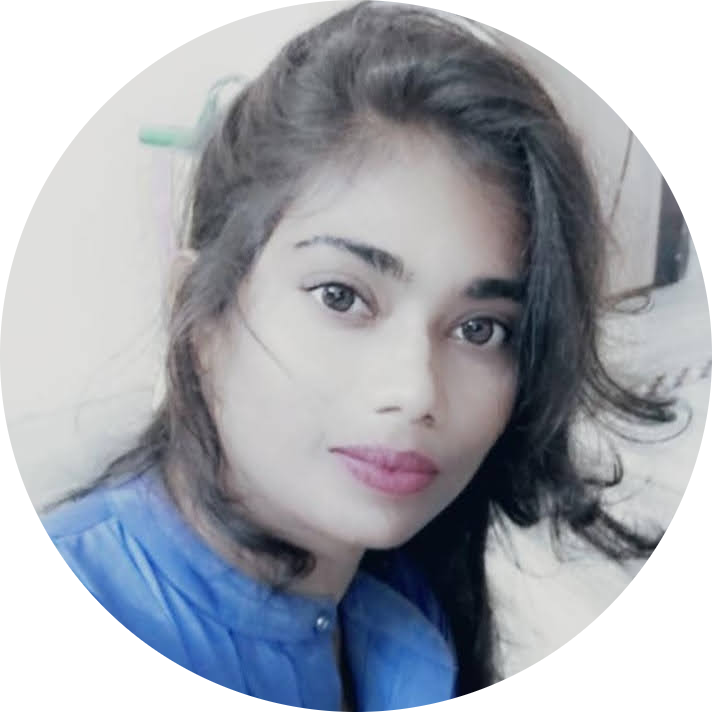
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
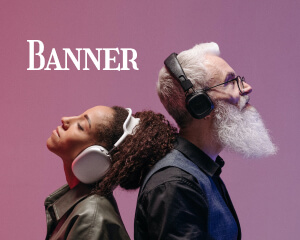