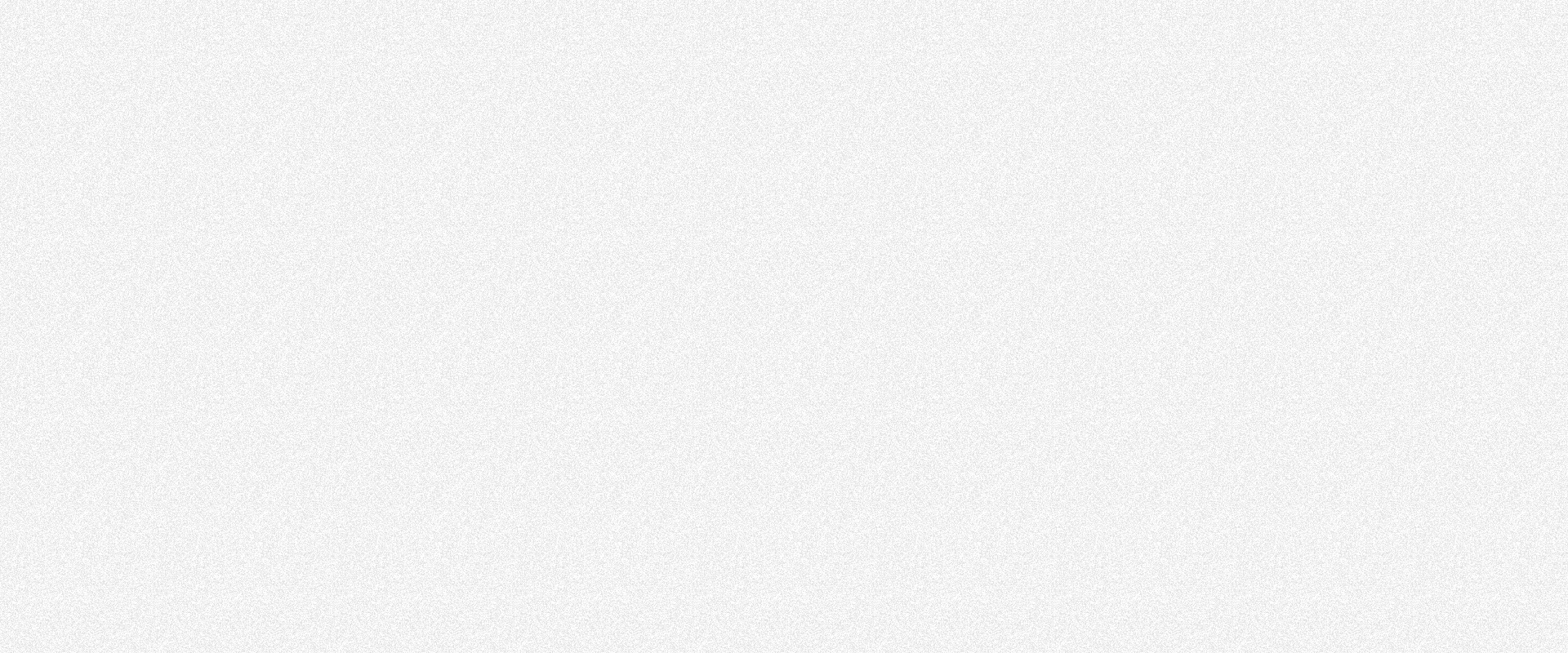
How do you create a custom meta box in a WordPress plugin?
If you’re a WordPress developer or looking to dive into WordPress plugin development, you may come across the need to add custom meta boxes to enhance the functionality of your plugins. Custom meta boxes allow users to input and store additional data for posts, pages, or custom post types. In this blog post, we will explore how to create a custom meta box in a WordPress plugin step by step. Don’t worry; we’ll keep it simple and easy to understand, even for a primary school student.
1. Introduction to Custom Meta Boxes
1.1 What is a Meta Box?
In WordPress, a meta box is a UI element that appears on the post-editing screen. It allows users to input and save extra information related to a particular post or page. These meta boxes are essential when you want to extend the default WordPress editor with custom fields.
1.2 Why Use Custom Meta Boxes?
Custom meta boxes offer a great way to add flexibility and customization to your WordPress plugins. Instead of relying solely on the default fields, you can create custom fields to cater to the specific requirements of your plugin users. This helps keep the interface clean and user-friendly.
2. Getting Started
2.1 Set Up Plugin File
First, create a new folder inside the “wp-content/plugins” directory and give it a unique name. Inside this folder, create a new PHP file, and this will serve as the main plugin file. Remember to start the file with the necessary plugin information like plugin name, description, author, version, etc.
2.2 The WordPress Action Hook
In your main plugin file, you’ll need to hook into WordPress to add your custom meta box. The most suitable action hook for this purpose is “add_meta_boxes.” This action allows you to add meta boxes when the post editor is initialized.
function custom_meta_box_setup() {
add_meta_box('custom_meta_box', 'Custom Meta Box Title', 'custom_meta_box_content', 'post', 'normal', 'high');
}
add_action('add_meta_boxes', 'custom_meta_box_setup');
In the above code snippet, we’ve created a custom meta box with the ID “custom_meta_box” and provided it with a title. It will be displayed on the post editing screen for the ‘post’ post type.
3. Creating the Meta Box Content
Now that we have set up the meta box, it’s time to define the content that will be displayed inside it.
3.1 Creating the Callback Function
The callback function is responsible for rendering the content of the meta box. We’ll create a function named “custom_meta_box_content” to handle this.
function custom_meta_box_content() {
// Add your meta box content here
}
3.2 Adding Input Fields
Inside the “custom_meta_box_content” function, you can add various input fields depending on your plugin’s requirements. Let’s add a simple text input field and a textarea:
function custom_meta_box_content() {
// Get the current value of the custom field
$custom_field_value = get_post_meta(get_the_ID(), 'custom_field', true);
// Output the text input field
echo '<label for="custom_field">Custom Field:</label>';
echo '<input type="text" id="custom_field" name="custom_field" value="' . esc_attr($custom_field_value) . '" style="width: 100%; margin-bottom: 15px;" />';
// Output the textarea
echo '<label for="custom_textarea">Custom Textarea:</label>';
echo '<textarea id="custom_textarea" name="custom_textarea" style="width: 100%;" rows="5">' . esc_textarea($custom_field_value) . '</textarea>';
}
4. Saving the Meta Box Data
Now that we have added input fields to our meta box, we need to save the data when the user updates or saves the post.
4.1 The Save Post Action
We’ll use the “save_post” action hook to save the custom meta box data when the user saves or updates a post.
function save_custom_meta_box_data($post_id) {
// Check if the current user has permission to edit the post.
if (!current_user_can('edit_post', $post_id)) {
return;
}
// Save custom field data
if (isset($_POST['custom_field'])) {
update_post_meta($post_id, 'custom_field', sanitize_text_field($_POST['custom_field']));
}
// Save custom textarea data
if (isset($_POST['custom_textarea'])) {
update_post_meta($post_id, 'custom_textarea', sanitize_textarea($_POST['custom_textarea']));
}
}
add_action('save_post', 'save_custom_meta_box_data');
In the above code, we’ve created a function named “save_custom_meta_box_data” that checks if the user has permission to edit the post and then updates the custom meta fields accordingly.
Conclusion
Congratulations! You’ve successfully learned how to create a custom meta box in a WordPress plugin. Meta boxes provide an excellent way to extend the functionality of your plugins and offer users a more tailored experience. As you delve deeper into WordPress development, you’ll find various possibilities for using custom meta boxes to enhance your projects. Keep experimenting and happy coding!
Remember, it’s essential to keep your plugins user-friendly, and custom meta boxes play a significant role in achieving that. So go ahead and start creating amazing WordPress plugins with custom meta boxes to delight your users!
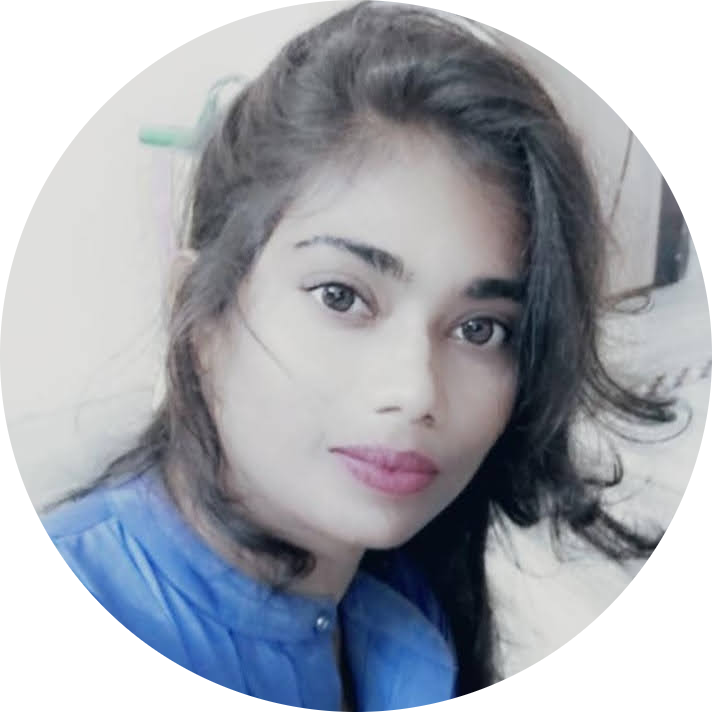
Komal
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
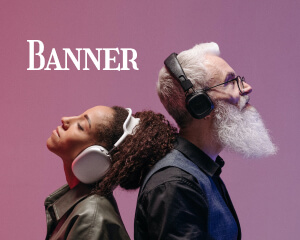