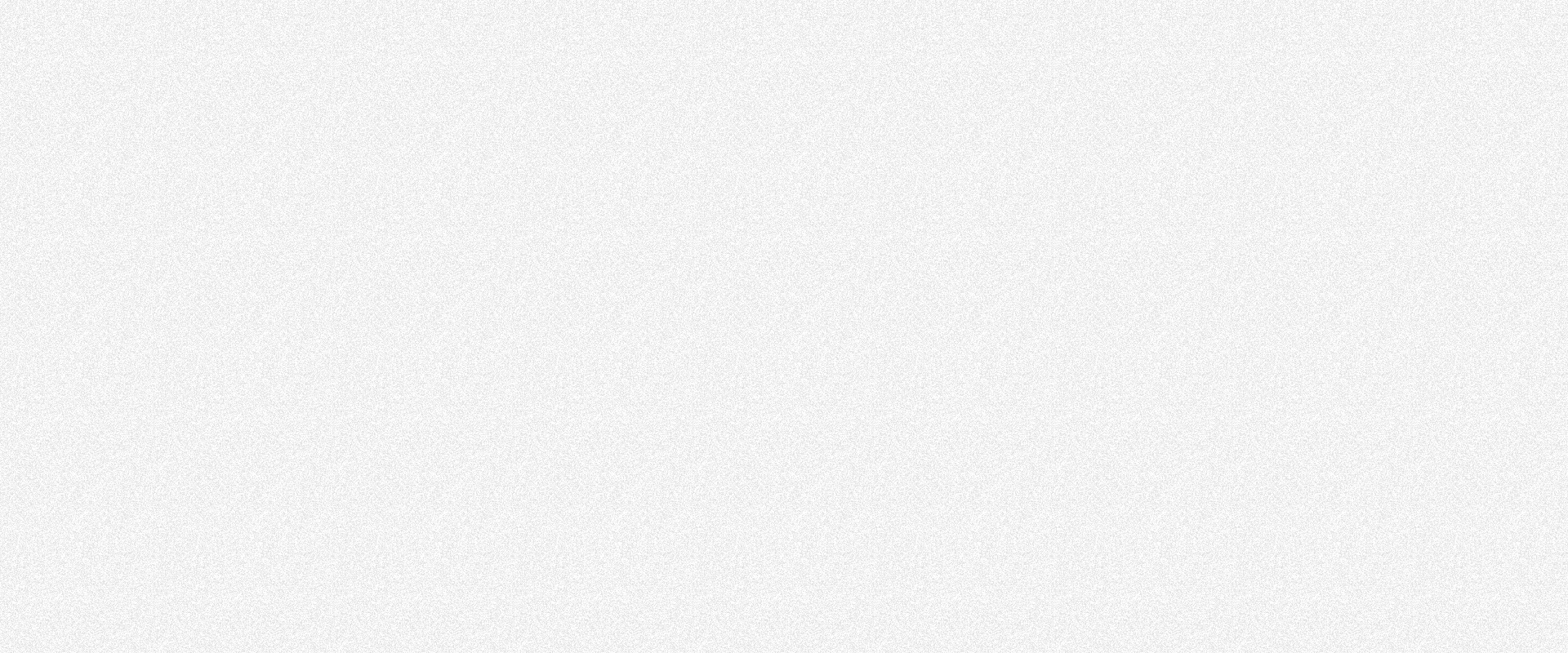
How do you add custom sections to the Theme Customizer?
The Theme Customizer is a powerful tool that allows website owners to personalize their themes and make real-time changes without altering the code directly. While most themes come with predefined sections, sometimes, you may want to add custom sections to meet specific design or functional requirements. In this article, we will explore how to do just that. We will provide you with complete code examples for adding custom sections, both in functions and templates, empowering you to create a website that truly stands out.
How do you add custom sections to the Theme Customizer?
To add custom sections to the Theme Customizer, follow these steps:
- Understanding the Theme Customizer Structure: Before we dive into adding custom sections, it’s essential to understand the structure of the Theme Customizer. The Theme Customizer is based on the WordPress Customizer API, which provides a set of functions and classes to control theme options. Familiarize yourself with how the Customizer works and the available controls and settings.
- Creating a Child Theme: Before making any changes to your theme, it’s best practice to create a child theme. A child theme inherits the parent theme’s functionality and styling, allowing you to make modifications without affecting the original theme’s code. Create a new folder in your themes directory, and inside that folder, create a
style.css
file with the necessary theme information. - Adding the Customizer Code: Open your child theme’s
functions.php
file and add the necessary code to enable the Theme Customizer. This code registers your custom sections, controls, and settings within the Customizer. Remember to use unique names for your custom sections to avoid conflicts with other themes or plugins. - Defining the Custom Sections: Now that you’ve enabled the Theme Customizer, it’s time to define your custom sections. You can create sections for various elements, such as header, footer, colors, typography, etc. Each section can contain multiple controls, giving users a wide range of options to customize their website.
- Adding Controls and Settings: Controls allow users to interact with the Customizer and make changes. Settings are responsible for storing the selected values. For instance, you can create a color picker control to change the website’s primary color and a text input control to modify the site title.
- Sanitizing and Validating Input: It’s essential to sanitize and validate user input to ensure data security and integrity. Sanitizing removes any unwanted characters or tags from user input, while validating ensures that the input meets specific criteria. WordPress provides built-in functions for sanitization and validation, making it easy to implement these measures.
- Previewing Changes: The Theme Customizer offers a live preview feature, allowing users to see the changes they make in real-time. Ensure that your custom sections are fully functional in the live preview, providing users with a seamless customization experience.
- Adding Custom Sections to Templates: While the Theme Customizer handles the backend customization, you may need to make corresponding changes to your theme templates to reflect the frontend changes. Update your templates to retrieve and apply the custom settings defined in the Customizer.
Function-based Code Example
Now, let’s dive into the code and see how you can add custom sections using functions. For this example, we’ll add a custom section to change the website’s logo.
// Step 1: Register the custom section
function custom_theme_customizer($wp_customize) {
$wp_customize->add_section('custom_logo_section', array(
'title' => __('Custom Logo', 'your_theme_domain'),
'priority' => 30,
));
}
add_action('customize_register', 'custom_theme_customizer');
// Step 2: Add the custom setting
function custom_logo_setting($wp_customize) {
$wp_customize->add_setting('custom_logo', array(
'default' => '',
'sanitize_callback' => 'esc_url_raw',
));
$wp_customize->add_control(new WP_Customize_Image_Control($wp_customize, 'custom_logo', array(
'label' => __('Upload a Custom Logo', 'your_theme_domain'),
'section' => 'custom_logo_section',
'settings' => 'custom_logo',
)));
}
add_action('customize_register', 'custom_logo_setting');
This code registers a new custom section named “Custom Logo” with a priority of 30. Inside the section, we add a custom setting for the logo. Users can upload their logo using the WP_Customize_Image_Control
control, and the logo URL is stored using the custom_logo
setting.
In HTML you can use by following code :
<?php
// Call the custom logo function to display the logo
display_custom_logo();
?>
Template-based Code Example
Now, let’s move on to adding custom sections to templates. In this example, we’ll apply the logo changes to the website header.
// Step 1: Retrieve the custom logo URL
function get_custom_logo_url() {
return get_theme_mod('custom_logo', '');
}
// Step 2: Display the custom logo in the header
function display_custom_logo() {
$custom_logo_url = get_custom_logo_url();
if (!empty($custom_logo_url)) {
echo '<img src="' . esc_url($custom_logo_url) . '" alt="Custom Logo">';
} else {
// Display the default logo if the custom logo is not set
echo '<img src="' . esc_url(get_template_directory_uri() . '/images/default-logo.png') . '" alt="Default Logo">';
}
}
In the above code, we create two functions. The get_custom_logo_url
function retrieves the custom logo URL saved in the Customizer settings. The display_custom_logo
function displays the logo in the header. If a custom logo is set, it will be displayed; otherwise, the default logo will be shown.
FAQs
Q: Can I add multiple custom sections to the Theme Customizer? A: Absolutely! You can add as many custom sections as you need to meet your website’s requirements. Each section can contain multiple controls, allowing users to customize various aspects of your theme.
Q: Do I need to know PHP to add custom sections to the Theme Customizer? A: Yes, some knowledge of PHP is necessary to add custom sections. However, with the provided code examples and understanding of the WordPress Customizer API, you can easily follow along and make the necessary changes.
Q: Will adding custom sections affect my theme’s performance? A: When done correctly, adding custom sections to the Theme Customizer should not significantly impact your theme’s performance. Ensure that you optimize your code and follow best practices to maintain optimal performance.
Q: Can I remove custom sections once they are added? A: Yes, you can remove custom sections by unregistering them using the remove_section
method. This allows you to make changes to your customizations easily.
Q: Is it necessary to create a child theme before adding custom sections? A: While it’s not strictly necessary, creating a child theme is highly recommended. Using a child theme ensures that your customizations won’t be lost when updating the parent theme.
Q: Can I extend the functionality of existing custom sections? A: Yes, you can extend the functionality of existing custom sections by adding new controls and settings. This allows you to enhance the user experience and provide more customization options.
Conclusion
Congratulations! You’ve now learned how to add custom sections to the Theme Customizer using complete code examples for both functions and templates. Customizing your theme gives you the creative freedom to make your website unique and appealing to your audience. Remember to follow best practices and always test your customizations before deploying them to ensure a smooth user experience. Now go ahead and make your website stand out with a custom look and feel!
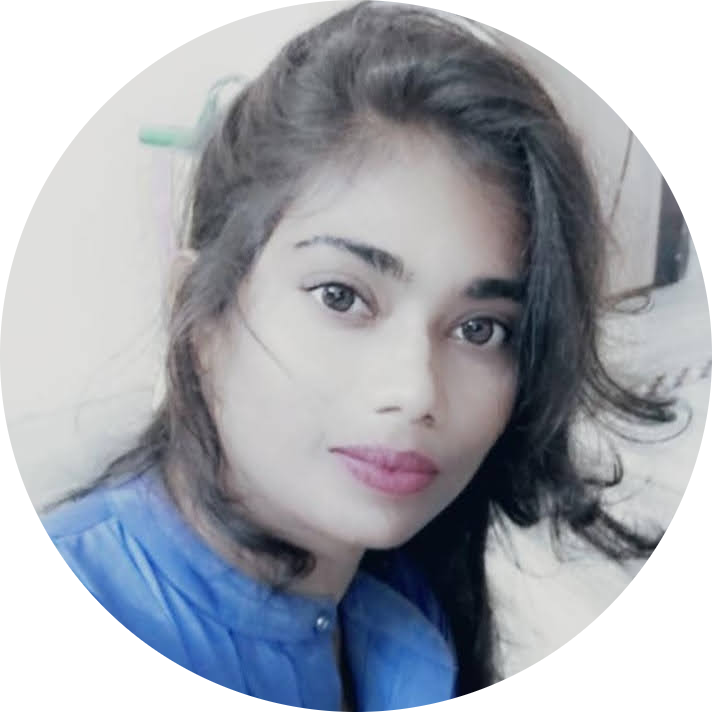
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
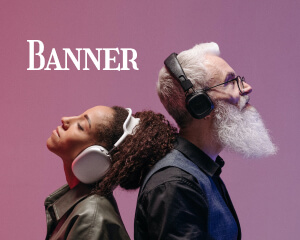