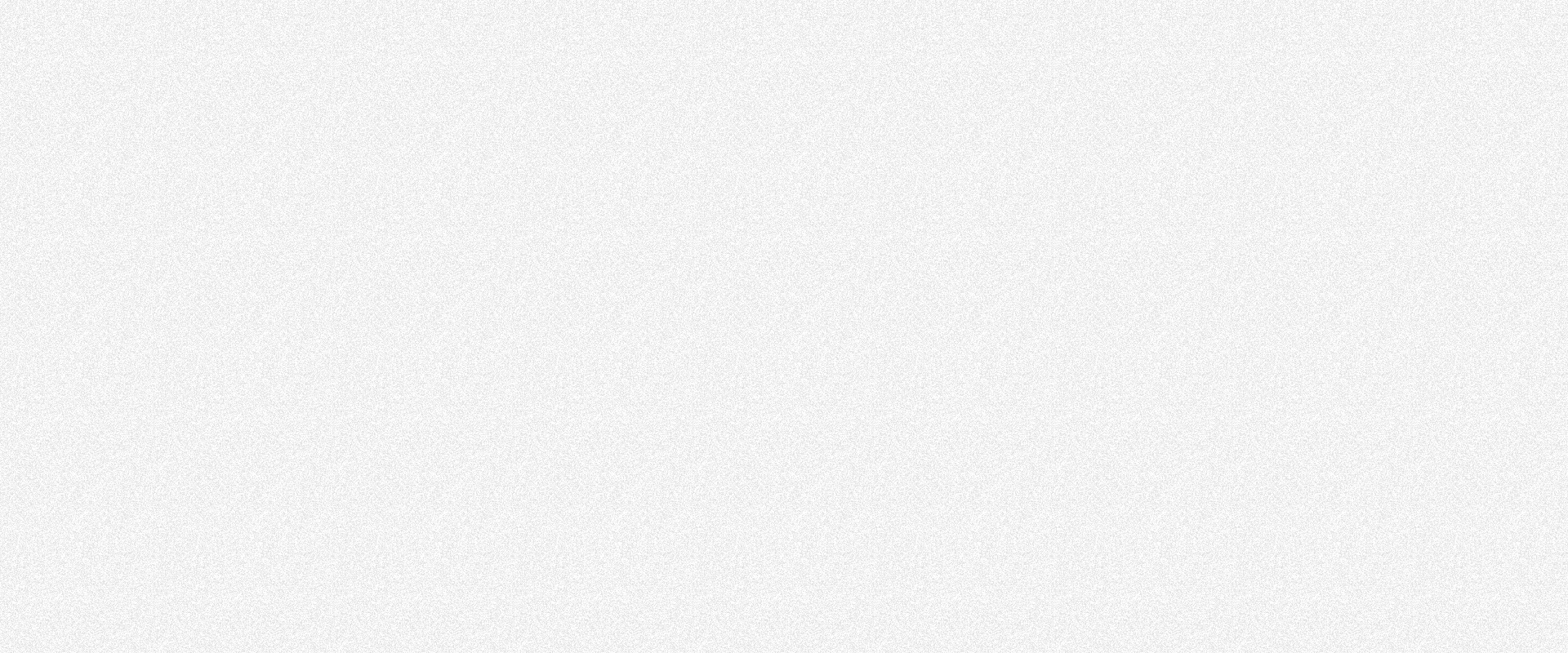
Creating Custom Post Types and Taxonomies in WordPress
Introduction
WordPress provides a powerful content management system (CMS) that allows you to create and manage various types of content. While WordPress comes with built-in post types like posts and pages, you can also create custom post types and taxonomies to organize and display specific types of content on your website. In this article, we will explore the process of creating custom post types and taxonomies in WordPress.
Understanding Custom Post Types
In WordPress, a post type is a specific type of content, such as posts, pages, attachments, or custom content you define. Custom post types allow you to extend the functionality of WordPress and create content that suits your specific needs.
Benefits of Using Custom Post Types
Using custom post types offers several benefits:
- Organized Content: Custom post types help you organize different types of content separately, making it easier to manage and navigate through your website.
- Improved User Experience: By creating custom post types, you can provide a tailored experience for your website visitors, focusing on specific content types and their associated features.
- Enhanced Functionality: Custom post types allow you to add custom fields, taxonomies, and other features specific to the type of content you’re creating.
- SEO Optimization: By structuring your content using custom post types, you can optimize it for search engines, improve indexing, and enhance the overall SEO performance of your website.
Creating Custom Post Types
To create a custom post type in WordPress, you can follow these steps:
- Access your WordPress website’s theme files.
- Open the
functions.php
file of your theme (or create a new file specifically for customizations). - Add the necessary code to define your custom post type, including labels, slug, supports, and other settings.
- Save the file and refresh your website’s admin dashboard.
Understanding Taxonomies
In WordPress, taxonomies are used to classify and group content. The built-in taxonomies are categories and tags. However, you can create custom taxonomies to organize your content more specifically.
Benefits of Using Taxonomies
Using custom taxonomies provides several benefits:
- Improved Content Organization: Custom taxonomies allow you to classify content into specific categories or terms, making it easier for users to find relevant information on your website.
- Flexible Content Filtering: Taxonomies provide a powerful way to filter and display content based on specific categories or terms, enhancing the user experience.
- SEO Optimization: Properly structured taxonomies can improve the SEO of your website by creating a logical hierarchy and enhancing the overall site structure.
Creating Custom Taxonomies
To create a custom taxonomy in WordPress, you can follow these steps:
- Access your WordPress website’s theme files.
- Open the
functions.php
file of your theme (or create a new file specifically for customizations). - Add the necessary code to define your custom taxonomy, including labels, slug, associated post types, and other settings.
- Save the file and refresh your website’s admin dashboard.
Displaying Custom Post Types and Taxonomies
To display custom post types and taxonomies on your WordPress website, you can utilize various methods:
- Template Files: Create custom template files to control the display of your custom post types and taxonomies.
- Custom Queries: Use custom queries to retrieve and display specific custom post types or taxonomies.
- Plugins: Utilize plugins that offer advanced display options and flexibility for custom post types and taxonomies.
Best Practices for Custom Post Types and Taxonomies
Consider the following best practices when creating custom post types and taxonomies in WordPress:
- Plan Ahead: Define your content structure and requirements before creating custom post types and taxonomies to ensure they align with your website’s goals.
- Use Descriptive Labels: Provide clear and descriptive labels for your custom post types and taxonomies to enhance usability and user understanding.
- Maintain Consistency: Follow consistent naming conventions and design patterns to ensure a cohesive user experience across your website.
- Keep Performance in Mind: Be mindful of the impact custom post types and taxonomies may have on your website’s performance and optimize accordingly.
- Regularly Review and Update: Periodically review your custom post types and taxonomies to ensure they meet the evolving needs of your website.
Here are five examples of custom post types along with code snippets to create and display them in WordPress:
Example 1: Portfolio Post Type
// Register Custom Post Type
function custom_portfolio_post_type() {
$labels = array(
'name' => 'Portfolio',
'singular_name' => 'Portfolio',
'menu_name' => 'Portfolio',
);
$args = array(
'label' => 'Portfolio',
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('portfolio', $args);
}
add_action('init', 'custom_portfolio_post_type');
To display the Portfolio post type, you can create a template file named archive-portfolio.php
in your theme directory. Customize the template to suit your design and use the following code to display the portfolio posts:
<?php
$args = array(
'post_type' => 'portfolio',
'posts_per_page' => 10,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
// Display your portfolio post content here
}
}
wp_reset_postdata();
?>
Example 2: Testimonial Post Type
// Register Custom Post Type
function custom_testimonial_post_type() {
$labels = array(
'name' => 'Testimonials',
'singular_name' => 'Testimonial',
'menu_name' => 'Testimonials',
);
$args = array(
'label' => 'Testimonials',
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor'),
);
register_post_type('testimonial', $args);
}
add_action('init', 'custom_testimonial_post_type');
To display the Testimonial post type, you can create a template file named archive-testimonial.php
in your theme directory. Customize the template and use the following code to display the testimonials:
<?php
$args = array(
'post_type' => 'testimonial',
'posts_per_page' => 5,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
// Display your testimonial content here
}
}
wp_reset_postdata();
?>
Example 3: Event Post Type
// Register Custom Post Type
function custom_event_post_type() {
$labels = array(
'name' => 'Events',
'singular_name' => 'Event',
'menu_name' => 'Events',
);
$args = array(
'label' => 'Events',
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('event', $args);
}
add_action('init', 'custom_event_post_type');
To display the Event post type, you can create a template file named archive-event.php
in your theme directory. Customize the template and use the following code to display the events:
<?php
$args = array(
'post_type' => 'event',
'posts_per_page' => 5,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
// Display your event content here
}
}
wp_reset_postdata();
?>
Example 4: Recipe Post Type
// Register Custom Post Type
function custom_recipe_post_type() {
$labels = array(
'name' => 'Recipes',
'singular_name' => 'Recipe',
'menu_name' => 'Recipes',
);
$args = array(
'label' => 'Recipes',
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('recipe', $args);
}
add_action('init', 'custom_recipe_post_type');
To display the Recipe post type, you can create a template file named archive-recipe.php
in your theme directory. Customize the template and use the following code to display the recipes:
<?php
$args = array(
'post_type' => 'recipe',
'posts_per_page' => 10,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
// Display your recipe content here
}
}
wp_reset_postdata();
?>
Example 5: Book Post Type
// Register Custom Post Type
function custom_book_post_type() {
$labels = array(
'name' => 'Books',
'singular_name' => 'Book',
'menu_name' => 'Books',
);
$args = array(
'label' => 'Books',
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('book', $args);
}
add_action('init', 'custom_book_post_type');
To display the Book post type, you can create a template file named archive-book.php
in your theme directory. Customize the template and use the following code to display the books:
<?php
$args = array(
'post_type' => 'book',
'posts_per_page' => 10,
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
// Display your book content here
}
}
wp_reset_postdata();
?>
Conclusion
Creating custom post types and taxonomies in WordPress allows you to extend the functionality of your website and organize content in a way that suits your specific needs. By understanding the benefits, following best practices, and utilizing appropriate display methods, you can create a more tailored and user-friendly experience for your website visitors.
FAQs
Q1: Can I create multiple custom post types on my WordPress website?
Yes, you can create multiple custom post types on your WordPress website. Each custom post type can have its own set of labels, settings, and functionality.
Q2: Can I associate custom taxonomies with multiple post types?
Yes, you can associate custom taxonomies with multiple post types. This allows you to classify and group content across different post types based on the defined taxonomy terms.
Q3: Do I need coding knowledge to create custom post types and taxonomies?
Creating custom post types and taxonomies typically requires some coding knowledge. However, there are plugins available that offer user-friendly interfaces to create and manage custom post types and taxonomies without writing code.
Q4: Can I modify existing post types or taxonomies in WordPress?
While it is generally recommended not to modify the built-in post types and taxonomies, you can customize their behavior and appearance using hooks and filters provided by WordPress.
Q5: Will creating custom post types and taxonomies affect my existing content?
Creating custom post types and taxonomies should not affect your existing content, as they are separate from the default post types and taxonomies in WordPress. However, it is always recommended to perform backups before making any significant changes to your website’s structure.
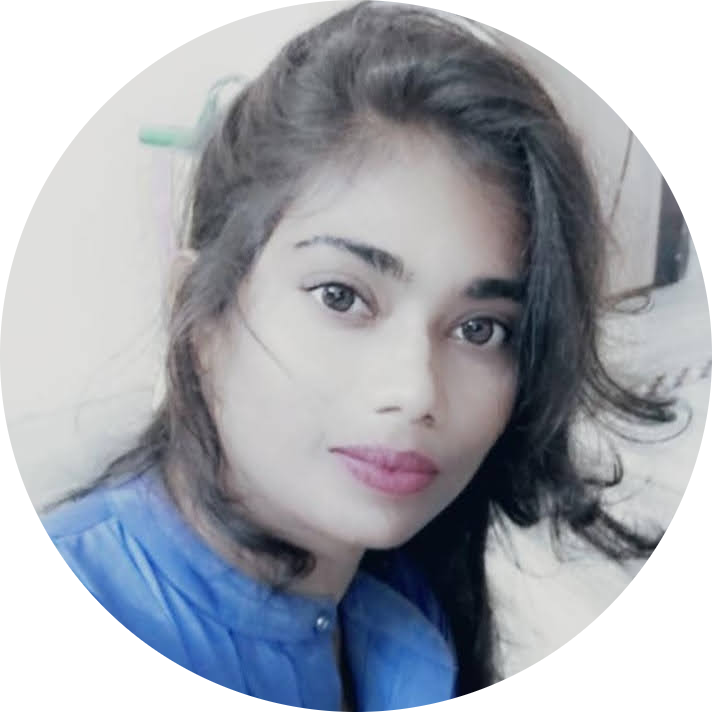
kumar
The Managing Director oversees the overall operations and strategic direction of the organization.
Category
- .NET (3)
- Blog (2)
- Common Technology (1)
- CSS (1)
- Topics (1)
- HTML (4)
- Basic HTML (4)
- Javascript (1)
- Topics (1)
- Laravel (32)
- Php (153)
- PHP Arrays (9)
- Php Basic (1)
- PHP interview (152)
- PHP Strings (12)
- Python (15)
- Python interview (13)
- React.js (32)
- React Js Project (3)
- Uncategorized (118)
- WordPress (114)
- Custom Post Types (7)
- Plugin Development (12)
- Theme Customizer (3)
- Theme Development (1)
- Troubleshooting (9)
- WordPress Customization (13)
- Wordpress Database (10)
- WordPress Example (5)
- WordPress Multisite (9)
- Wordpress Project (3)
- WordPress REST API (14)
- WordPress SEO (10)
Recent Posts
Tags
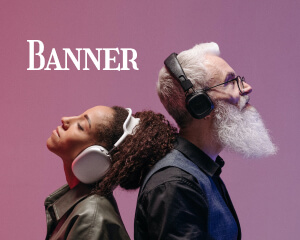